Obter Tamanho do ficheiro em C++
-
Utilize a função
std::filesystem::file_size
para obter o tamanho do ficheiro em C++ -
Utilizar
std::filesystem::file_size
Comstd::filesystem::path
para obter o tamanho do ficheiro em C++ -
Utilize
std::filesystem::file_size
Comerror_code
para obter o tamanho do ficheiro em C++ -
Utilize a função
stat
para obter o tamanho do ficheiro em C++
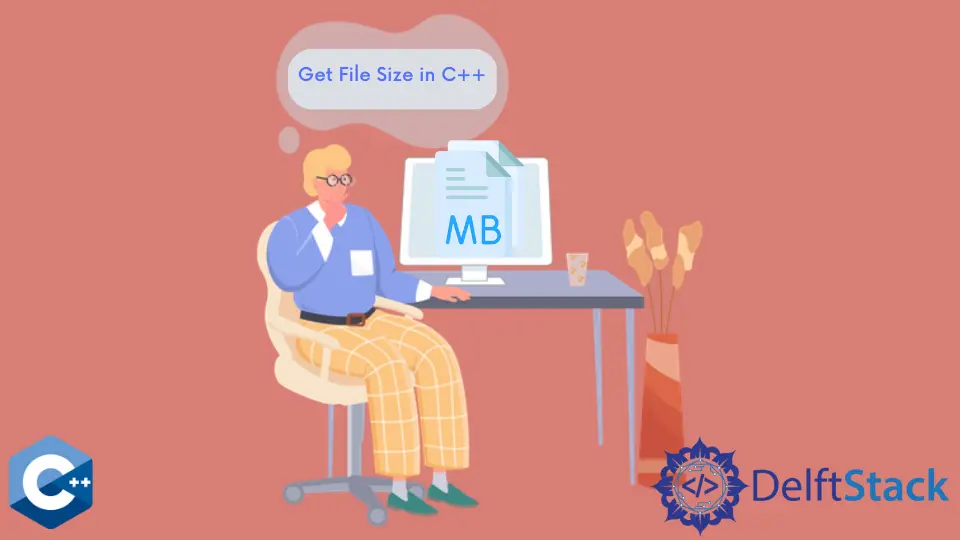
Este artigo irá demonstrar múltiplos métodos de como obter o tamanho do ficheiro em C++.
Utilize a função std::filesystem::file_size
para obter o tamanho do ficheiro em C++
std::filesystem::file_size
é a função da biblioteca do sistema de ficheiros C++ que recupera o tamanho do ficheiro em bytes. std::filesystem::file_size
toma o caminho do ficheiro como argumento da função, que é do tipo std::filesystem::path
. No exemplo seguinte, porém, passamos o valor string
do caminho do ficheiro, que é depois utilizado para construir o objecto correspondente e recuperar o tamanho do ficheiro.
#include <filesystem>
#include <fstream>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::ifstream;
int main() {
string path = "input.txt";
cout << "size of file '" << path << "' = " << std::filesystem::file_size(path)
<< endl;
return EXIT_SUCCESS;
}
Resultado:
size of file 'inbox.txt' = 10440
Utilizar std::filesystem::file_size
Com std::filesystem::path
para obter o tamanho do ficheiro em C++
A utilização alternativa da função std::filesystem::file_size
é inserir o valor do caminho do ficheiro como a variável do tipo path
. No início, seria necessário incluir a declaração use std::filesystem::path
antes de utilizar o tipo. A seguir, podemos declarar o objecto path
, como mostrado no seguinte código de exemplo, e inicializá-lo com uma string literal. A variável construída é passada para a função file_size
para obter o tamanho do ficheiro.
#include <fcntl.h>
#include <sys/stat.h>
#include <unistd.h>
#include <filesystem>
#include <fstream>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::error_code;
using std::ifstream;
using std::string;
using std::filesystem::path;
int main() {
class path fd_path = "input.txt";
cout << "size of file '" << fd_path
<< "' = " << std::filesystem::file_size(fd_path) << endl;
return EXIT_SUCCESS;
}
Resultado:
size of file 'inbox.txt' = 10440
Utilize std::filesystem::file_size
Com error_code
para obter o tamanho do ficheiro em C++
Uma vez que std::filesystem::file_size
utiliza serviços de sistema operativo subjacentes para recuperar o tamanho do ficheiro, há boas probabilidades de este falhar, levantando alguma excepção. Para melhor lidar com cada chamada de função file_size
, recomenda-se utilizar o objecto error_code
como segundo argumento e armazenar a mensagem de erro em caso de falha. Consequentemente, podemos avaliar o objecto error_code
e implementar o código de tratamento de erros utilizando declarações if...else
.
#include <filesystem>
#include <fstream>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::error_code;
using std::ifstream;
using std::string;
using std::filesystem::path;
int main() {
string path = "input.txt";
error_code ec{};
auto size = std::filesystem::file_size(path, ec);
if (ec == error_code{})
cout << "size of file '" << path << "' = " << size << endl;
else
cout << "Error accessing file '" << path << "' message: " << ec.message()
<< endl;
return EXIT_SUCCESS;
}
Resultado:
size of file 'inbox.txt' = 10440
Utilize a função stat
para obter o tamanho do ficheiro em C++
O stat
é a função compatível com POSIX que está disponível em múltiplos sistemas operativos. É muitas vezes a função que o método std::filesystem::file_size
por baixo do capô. O stat
toma a string como primeiro argumento para denotar o caminho do endereço do ficheiro do objecto do tipo struct stat
como segundo argumento.
O struct stat
é um objecto especial pré-definido que armazena múltiplos parâmetros de ficheiro após a chamada bem sucedida da função stat
. Note-se que só precisamos de aceder ao membro de dados st_size
do objecto struct stat
, que armazena o tamanho do ficheiro como um número de bytes.
#include <fcntl.h>
#include <sys/stat.h>
#include <unistd.h>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
int main() {
string path = "input.txt";
struct stat sb {};
if (!stat(path.c_str(), &sb)) {
cout << "size of file '" << path << "' = " << sb.st_size << endl;
} else {
perror("stat");
}
return EXIT_SUCCESS;
}
Resultado:
size of file 'inbox.txt' = 10440
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook