Function Returns the Address of a Local Variable Error in C++
- Function Returns the Address of a Local Variable Error in C++
- Cause of the Function Returns the Address of a Local Variable in C++
- Examples Where the Function Returns the Address of a Local Variable Error Occurs in C++
- Conclusion
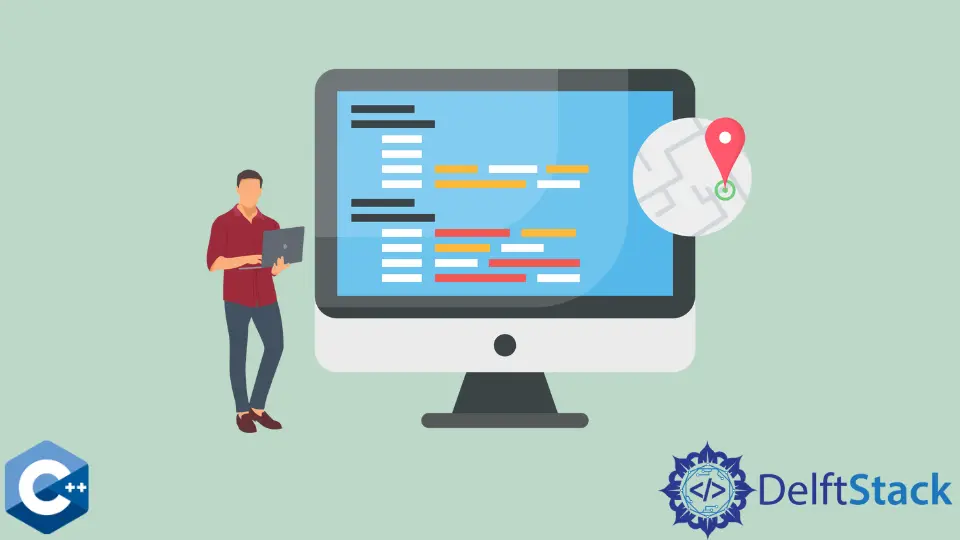
Based on the scope, variables in C and C++ are divided into local and global variables. While the global variables can be accessed from any part of the program, the same is not true for local variables.
Thus, using them carefully when various functions are involved in the code is important. Otherwise, you might encounter the address of local variable returned
error.
Let us discuss why this error occurs and how to fix it.
Function Returns the Address of a Local Variable Error in C++
To understand the reasons and possible fixes for the function returns the address of the local variable
error, we must know how a local variable works. Let us discuss this first.
The Behavior of a Local Variable in C++
A local variable is a variable that is defined inside a function and cannot be used outside that particular function. In other words, it exists only inside the function where it was defined and cannot be accessed from any other part of the program.
And here is a very important piece of information - the life of a local variable is destroyed as soon as the function ends its execution.
Look at this example. Here, the variable cost
is declared inside the function demo()
; hence, its scope is limited to this function only.
Inside the main
block, we try to access this local variable, and therefore, the program runs into an error.
This is essentially how a local variable works.
#include <iostream>
using namespace std;
void demo() {
int cost = 5000;
cout << "The variable cost is local to the demo function." << endl;
}
int main() {
int cakes = 10;
demo();
cout << "Payable amount: " << cost;
}
Output:
In function 'int main()':
error: 'cost' was not declared in this scope
13 | cout << "Payable amount: " << cost;
| ^~~~
Now you know how using a local variable outside its scope is illegal. Let us discuss why the function returns the address of the local variable
error occurs.
To know more about local variables and functions in C++, refer to this documentation.
Cause of the Function Returns the Address of a Local Variable in C++
The function returns the address of the local variable
error usually occurs while working with functions and local variables. The way we cannot access a local variable outside its defined scope, we also cannot access its address outside that scope.
Look at it like this. When you try to access a local variable outside the defined scope, you get the variable not declared in this scope
error, as seen in the example above.
However, if you try to access the address of a local variable outside the defined scope, you get the function returns the address of the local variable
error.
Here is why this happens.
We know a local variable is destroyed once the function completes its execution. This means that the pointer returning the address of the local variable now points to an object that does not even exist.
Pointers to local variables cannot go outside the function where the local variable lives. But when this happens, we can say that the pointer changes into a dangling pointer, and thus, we get the function returns the address of the local variable
error.
This error might occur in various cases. Let us discuss them one by one.
Examples Where the Function Returns the Address of a Local Variable Error Occurs in C++
While the cause of all such codes running into the function returns the address of the local variable
error is the same as discussed above, let us look at a few examples in detail.
Function Returns a Pointer to a Local Variable
Look at the code given below. We have a function called demo()
, which takes two integer values and adds them.
The function stores the sum in the variable cost
and then returns the address of this variable. But when you run this code, you get the address of local variable returned
error.
Note two things here.
- The variable
cost
is defined inside the functiondemo()
; thus, its scope is limited to this function only. It is a local variable. - The
return
statement is returning a pointer having the address of the local variable,cost
.
We know that as soon as the function demo()
finishes its execution, the local variable cost
present inside it also gets destroyed. This means that the pointer that was earlier pointing to the address of the variable cost
now starts pointing to a place in memory that we no longer own.
Thus, we get this error.
#include <iostream>
using namespace std;
int* demo(int cherry, int pie) {
int cost = cherry + pie;
return &cost;
}
int main() { int* ans = demo(100, 20); }
Output:
In function 'int* demo(int, int)':
warning: address of local variable 'cost' returned [-Werturn-local-addr]
7 | return &cost;
| ^~~~~
note: declared here
6 | int cost = cherry + pie;
| ^~~~
Let us see how to fix this. The problem is that the return address is that of a local variable; thus, dynamic memory allocation will help resolve this.
Inside the main
block, we dynamically define the variable cost
and allocate memory. Then, we pass this variable as a parameter to the demo()
function.
#include <iostream>
using namespace std;
int* demo(int cherry, int pie, int* cost) {
*cost = cherry + pie;
return cost;
}
int main() {
int* cost = new int;
cost = demo(100, 20, cost);
cout << "The total is stored at address: " << cost;
}
Output:
The total is stored at address: 0x55c7997a7eb0
When we run this code, we get the correct output this time. This is because the variable cost
is no longer a local variable as it is declared outside the demo()
function.
Function Returns a String Whose Scope Is Local
Look at the following code snippet. Here, we take a name as an input from the user and check if it is valid using the checkname()
function.
Inside the checkname()
function, we define a string called fullname
and return it if it passes the check condition. But the code runs into the function returns the address of the local variable
error.
Here is why this happens.
We have a string called fullname
whose scope is limited to the checkname()
function. Also, a string is like an array that is reduced to a pointer before it can be passed.
When we return to the main
block, the function’s local variables will be destroyed because the control has moved out of that function.
This is why we get the error.
#include <cstring>
#include <iostream>
using namespace std;
char* checkname() {
char fullname[20];
//....
//......
//....
if ((strlen(fullname)) >= 4 && (strlen(fullname)) < 30) {
return fullname;
} else {
return NULL;
}
}
int main(void) {
char name[20];
cout << "Enter name:" << endl;
name = checkname();
}
Output:
warning: address of local variable 'fullname' returned [-Wreturn-local-addr]
To fix this, instead of returning the data, pass it to the function like this:
#include <cstring>
#include <iostream>
using namespace std;
void checkname(char *fullname) {
if ((strlen(fullname)) >= 4 && (strlen(fullname)) < 30) {
cout << "Success";
} else {
cout << "In else block";
}
}
int main(void) {
char name[20];
cout << "Enter name:" << endl;
checkname(name);
}
Output:
Enter name:
In else block
See, the code runs fine this time, and since we have not taken any input, we go inside the else
block.
While this solves the problem, a more robust solution would be to dynamically allocate the memory to the variable fullname
like this:
char *fullname = malloc(sizeof(char) * the_size);
.......return fullname;
When we allocate memory dynamically, it is allocated from the heap whose lifetime is valid until the entire program’s execution. Therefore, when we return the variable fullname
now, we get the address defined by malloc
, and the code runs fine.
Also, freeing the memory once done is a good practice to avoid memory leaks.
Conclusion
This article discussed the function returns the address of a local variable
error in C++. We learned how local variables behave and how trying to access them outside their scope is illegal.
We saw the reasons for this error and the possible solutions with the help of some use cases.