Too Many Arguments to Function Error in C++
- Functions in C++
-
the
too many arguments to function
Error in C++ -
Resolve the
too many arguments to function
Error in C++ - Conclusion
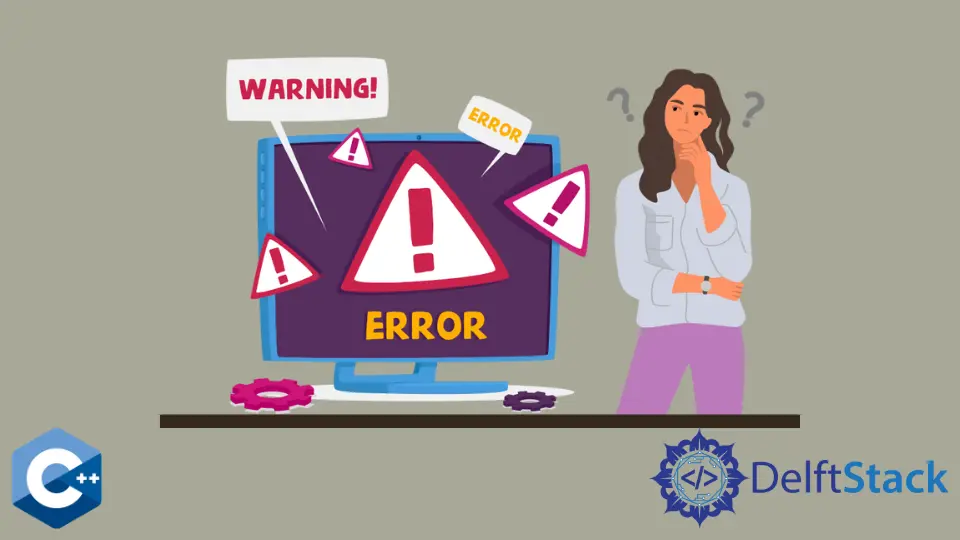
We encounter a lot of errors while writing a piece of code. Solving errors is one of the most crucial parts of programming.
This article will discuss one error we encounter in C++: too many arguments to function
.
Functions in C++
The too many arguments to function
is an error in C++ that we encounter when we specify different arguments in the declaration and the implementation of a function.
To understand this error and fix it, we should first discuss what functions are.
A function is a block of code that is reusable and is used to perform repetitive tasks. Suppose we want to print "Hello World"
in a program five times.
Now, one way to do so is to write the print
statement every time we want to print it, whereas the other efficient way would be to make a function where the code to write "Hello World"
would be written, and this function would be called whenever we need it.
The function that prints "Hello World"
will be implemented below.
#include <iostream>
using namespace std;
void print();
int main() {
print();
print();
print();
print();
return 0;
}
void print() { cout << "Hello World" << endl; }
Output:
Hello World
Hello World
Hello World
Hello World
However, if we write the print()
function after the main()
, then we need to first declare the function before the main()
so that the compiler knows that a function named print()
exists in the program.
the too many arguments to function
Error in C++
Arguments are values that are defined when a function is called. The too many arguments to function
error occur because of these arguments in a function.
The number of arguments should be the same in the function call and the function declaration of a program. We get this error when the number of arguments is different in the function declaration and function call.
Let us see a code to depict what these different arguments mean. Below is the code snippet that throws an error stating that there are too many arguments in a function.
#include <iostream>
using namespace std;
void addition();
int main() {
addition(4, 5);
return 0;
}
void addition(int a, int b) {
cout << "Addition of the numbers " << a << " and " << b << " = " << (a + b)
<< endl;
}
Output:
./6e359960-d9d7-4843-86c0-b9394abfab38.cpp: In function 'int main)':
./6e359960-d9d7-4843-86c0-b9394abfab38.cpp:7:14: error: too many arguments to function 'void addition)'
addition4,5);
^
./6e359960-d9d7-4843-86c0-b9394abfab38.cpp:4:6: note: declared here
void addition);
^
In the above code snippet, we have used the function addition()
to add the two numbers. On the third line, we have declared the function addition()
with no arguments (arguments are present in the parentheses of a function).
However, when we make or define the addition()
function, we have passed two arguments, a
and b
, to it so that these two numbers can be added. Therefore, this mismatch in the number of arguments causes the too many arguments to function
.
Resolve the too many arguments to function
Error in C++
The too many arguments to function
error in C++ can be resolved by considering the number of arguments passed to the function when declaring and defining it.
Let us take the same example as above and resolve its error.
#include <iostream>
using namespace std;
void addition(int, int);
int main() {
addition(4, 5);
return 0;
}
void addition(int a, int b) {
cout << "Addition of the numbers " << a << " and " << b << " = " << (a + b)
<< endl;
}
Output:
Addition of the numbers 4 and 5 = 9
Therefore, as you can see, the error has been removed, and our result of adding two numbers has been displayed successfully. We have just added the two int
types to the declaration of the function on the 3rd line.
This way, before encountering the addition()
function, the compiler knows that an addition()
function exists in our program that will take arguments in it.
Conclusion
This article has discussed too many arguments to function
. We have discussed functions briefly, how this error occurs in the C++ program and how to resolve the same.
However, the main thing while resolving this error would be that there should be the same number of arguments wherever the function is defined or declared.