How to Handle Errors in C++
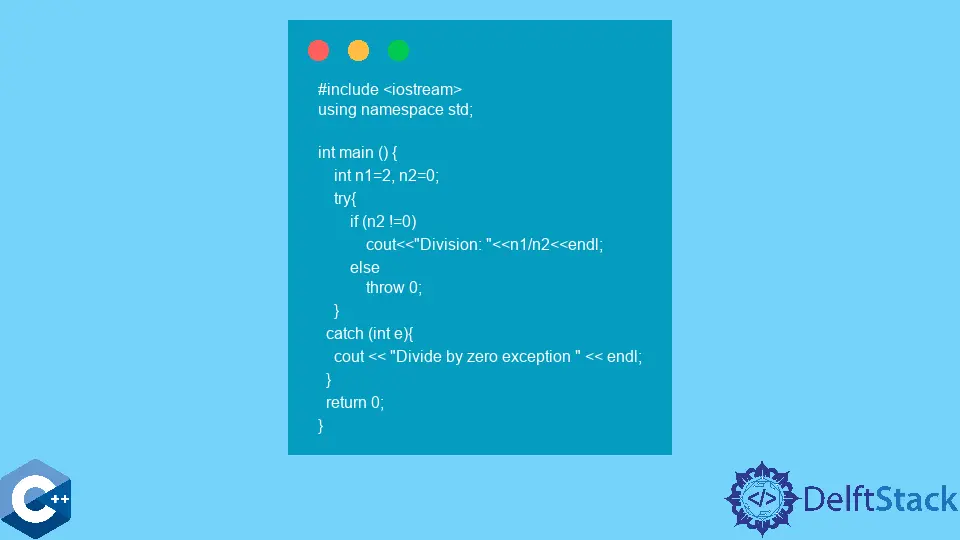
Error handling is a crucial aspect of programming, especially in C++. When you write a program, unexpected situations can arise, leading to runtime errors and crashes. Knowing how to handle these errors effectively can save you from hours of debugging and frustration. This guide will walk you through the various methods to handle errors in C++, ensuring that your code runs smoothly and efficiently. Whether you are a novice or a seasoned developer, mastering error handling will significantly enhance your programming skills. Let’s dive into the different strategies you can use to gracefully manage errors in your C++ applications.
Understanding Error Types
Before we jump into handling errors, it’s essential to understand the types of errors you might encounter in C++. Broadly speaking, errors can be categorized into three types: syntax errors, runtime errors, and logical errors.
- Syntax Errors: These occur when your code violates the grammatical rules of C++. The compiler flags these errors, making them relatively easy to fix.
- Runtime Errors: These happen during the execution of the program. They can be caused by invalid operations, such as dividing by zero or accessing out-of-bounds array elements.
- Logical Errors: These are tricky as they do not cause the program to crash but lead to incorrect results. Debugging these errors requires careful examination of the code logic.
Understanding these error types is the first step in implementing effective error handling strategies.
Using Try-Catch Blocks
One of the most common methods for handling errors in C++ is using try-catch blocks. This mechanism allows you to catch exceptions and handle them gracefully without crashing your program.
#include <iostream>
#include <stdexcept>
int divide(int a, int b) {
if (b == 0) {
throw std::runtime_error("Division by zero!");
}
return a / b;
}
int main() {
try {
std::cout << divide(10, 0) << std::endl;
} catch (const std::runtime_error& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
Output:
Error: Division by zero!
In this code, we define a function called divide
that throws a runtime error if the divisor is zero. The main
function contains a try block where we attempt to call divide
. If an exception occurs, the catch block captures it and prints an error message. This structure allows your program to continue running even when an error occurs, improving user experience.
Using Error Codes
Another method for error handling in C++ is using error codes. This approach involves returning a specific error code from functions to indicate success or failure.
#include <iostream>
enum ErrorCode {
SUCCESS,
DIVIDE_BY_ZERO
};
ErrorCode divide(int a, int b, int& result) {
if (b == 0) {
return DIVIDE_BY_ZERO;
}
result = a / b;
return SUCCESS;
}
int main() {
int result;
ErrorCode code = divide(10, 0, result);
if (code == DIVIDE_BY_ZERO) {
std::cerr << "Error: Division by zero!" << std::endl;
} else {
std::cout << "Result: " << result << std::endl;
}
return 0;
}
Output:
Error: Division by zero!
In this example, we use an enumeration to define error codes. The divide
function returns an error code, which the main
function checks to determine how to proceed. This method provides clear feedback about the success or failure of operations, making it easier to handle errors systematically.
Using Assertions
Assertions are another useful tool for error handling in C++. They allow you to set conditions that must be true during runtime. If an assertion fails, the program terminates, providing immediate feedback about the issue.
#include <iostream>
#include <cassert>
int divide(int a, int b) {
assert(b != 0 && "Division by zero!");
return a / b;
}
int main() {
std::cout << divide(10, 2) << std::endl;
std::cout << divide(10, 0) << std::endl; // This will trigger the assertion
return 0;
}
Output:
5
Assertion failed: Division by zero!
In this code, the assert
function checks if the divisor is not zero. If the condition is false, the program will terminate and print an assertion failure message. While assertions are not a replacement for error handling, they are beneficial during the development phase to catch programming mistakes early.
Conclusion
Handling errors in C++ is vital for creating robust applications. By implementing methods such as try-catch blocks, error codes, and assertions, you can manage errors effectively and enhance the stability of your code. Remember, the goal is not just to catch errors but to handle them gracefully, ensuring a smooth user experience. As you continue to develop your C++ skills, mastering error handling will be a valuable asset in your programming toolkit.
FAQ
- What are the common types of errors in C++?
Syntax errors, runtime errors, and logical errors are the most common types of errors in C++.
-
What is the purpose of try-catch blocks?
Try-catch blocks allow you to catch exceptions and handle errors gracefully without crashing your program. -
How do error codes work in C++?
Error codes are returned from functions to indicate success or failure, allowing developers to handle errors systematically. -
What are assertions, and when should I use them?
Assertions are conditions that must be true during runtime. They are useful for catching programming mistakes during development. -
Can I combine different error handling methods in C++?
Yes, you can combine different methods like try-catch blocks and error codes to create a comprehensive error handling strategy.