How to Compare Arrays in C++
-
Compare Arrays in C++ Using a
for
Loop Statement - Compare Arrays in C++ Using Custom Defined Function
-
Compare Arrays in C++ Using the
std::equal
Algorithm -
Compare Arrays in C++ Using the
std::memcmp
Function -
Compare Arrays in C++ Using the
std::vector
Comparison -
Compare Arrays in C++ Using the
std::mismatch
Algorithm - Conclusion
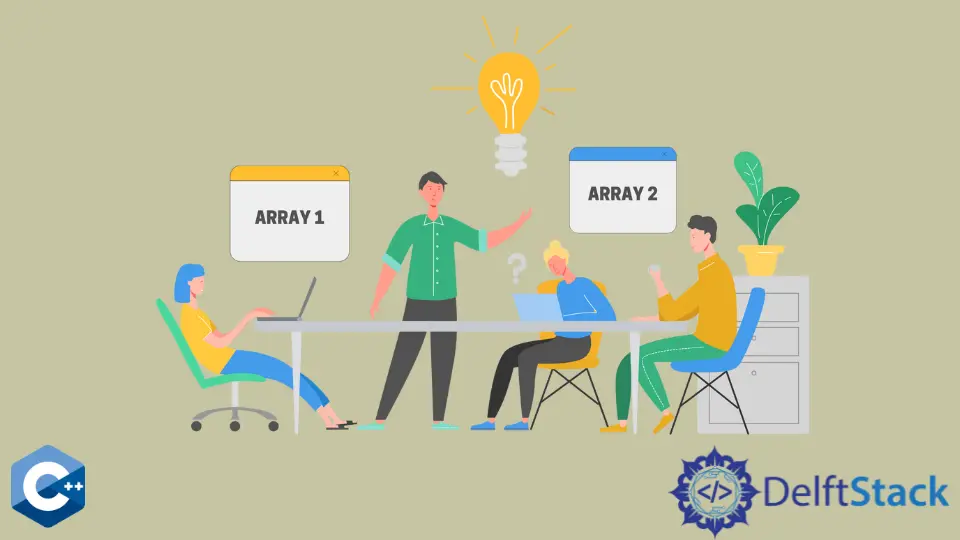
In C++, array comparison is a fundamental operation frequently encountered in various programming tasks. Whether validating data integrity, debugging, or implementing specific logic, having an understanding of different methods for comparing arrays is important.
This article explores six distinct approaches in C++ to compare arrays, each offering its unique advantages. From traditional for
loop statements to the standard library with std::equal
, std::memcmp
, and std::mismatch
, to the std::vector
comparisons and the customization of user-defined functions, we will delve into the syntax, workings, and practical applications of each method.
Compare Arrays in C++ Using a for
Loop Statement
One straightforward method to compare array contents is by using the for
loop statement.
The basic structure of using a for
loop to compare arrays involves iterating through each element of both arrays and checking for equality. Here’s a simple overview of the syntax:
for (int i = 0; i < size; ++i) {
// Compare array elements at index i
// If elements are not equal, arrays are not the same
}
In this structure, size
represents the size of the arrays. The i
is the loop variable that iterates from 0 to size-1
.
Inside the loop, you compare elements at index i
of both arrays.
Let’s dive into a complete working code example demonstrating the comparison of arrays using the for
loop statement:
#include <iostream>
using namespace std;
bool compareArrays(const int arr1[], const int arr2[], size_t size) {
for (size_t i = 0; i < size; ++i) {
if (arr1[i] != arr2[i]) {
return false; // Arrays are not the same
}
}
return true; // Arrays are the same
}
int main() {
const size_t arraySize = 5;
int array1[arraySize] = {1, 2, 3, 4, 5};
int array2[arraySize] = {1, 2, 3, 4, 5};
int array3[arraySize] = {1, 2, 3, 4, 6};
if (compareArrays(array1, array2, arraySize)) {
cout << "Arrays array1 and array2 are the same." << endl;
} else {
cout << "Arrays array1 and array2 are not the same." << endl;
}
if (compareArrays(array1, array3, arraySize)) {
cout << "Arrays array1 and array3 are the same." << endl;
} else {
cout << "Arrays array1 and array3 are not the same." << endl;
}
return 0;
}
In the above example, the compareArrays
function takes two arrays (arr1
and arr2
) along with their size as parameters. Inside the function, the for
loop iterates through each element, comparing them.
If any elements are not equal, the function returns false
, indicating that the arrays are not the same. If the loop completes without finding any unequal elements, the function returns true
, signifying that the arrays are identical.
Code Output:
This demonstrates how the for
loop statement is effectively used to compare arrays in C++, providing a clear understanding of the syntax and implementation.
Compare Arrays in C++ Using Custom Defined Function
Comparing arrays in C++ can be made more versatile by using a custom-defined function. This approach allows for flexibility in handling different types of arrays and facilitates customization if additional functionality or specific comparisons are needed.
The syntax for a custom-defined function to compare arrays typically involves creating a function that takes two array parameters and returns a Boolean value indicating whether the arrays are equal. Here’s a simplified example:
template <typename T>
bool compareArrays(const T arr1[], const T arr2[], size_t size) {
// Comparison logic
}
In this structure, T
is a template parameter representing the data type of the arrays.
The arr1
and arr2
are the arrays to be compared. The size
is the size of the arrays.
Let’s explore a complete working example of comparing arrays using a custom-defined function:
#include <iostream>
#include <vector>
using namespace std;
template <typename T>
bool compareArrays(const T arr1[], const T arr2[], size_t size) {
for (size_t i = 0; i < size; ++i) {
if (arr1[i] != arr2[i]) {
return false; // Arrays are not the same
}
}
return true; // Arrays are the same
}
int main() {
const size_t arraySize = 5;
int array1[arraySize] = {1, 2, 3, 4, 5};
int array2[arraySize] = {1, 2, 3, 4, 5};
int array3[arraySize] = {1, 2, 3, 4, 6};
if (compareArrays(array1, array2, arraySize)) {
cout << "Arrays array1 and array2 are the same." << endl;
} else {
cout << "Arrays array1 and array2 are not the same." << endl;
}
if (compareArrays(array1, array3, arraySize)) {
cout << "Arrays array1 and array3 are the same." << endl;
} else {
cout << "Arrays array1 and array3 are not the same." << endl;
}
return 0;
}
In this code example, the compareArrays
function is a template function that takes the same arrays (arr1
and arr2
) along with their size as parameters. The function uses a for
loop to iterate through each element, comparing them.
Similar to the previous example, if any elements are not equal, the function returns false
, indicating that the arrays are not the same. If the loop completes without finding any unequal elements, the function returns true
, signifying that the arrays are identical.
Code Output:
This showcases how a custom-defined function can be employed to compare arrays in C++, providing a clear and modular approach for array comparisons.
Compare Arrays in C++ Using the std::equal
Algorithm
Comparing arrays in C++ can be streamlined by leveraging the std::equal
algorithm from the <algorithm>
header. This algorithm provides a concise and efficient way to compare the contents of two arrays.
The syntax for using std::equal
involves passing the iterators representing the beginning and end of the ranges to be compared. Here’s a basic overview:
#include <algorithm>
bool isEqual = std::equal(first1, last1, first2, last2);
In this structure, first1
and last1
define the range of the first array.
The first2
and last2
define the range of the second array. The isEqual
is a Boolean variable indicating whether the ranges are equal.
Let’s explore a complete working example of comparing arrays using std::equal
:
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main() {
const size_t arraySize = 5;
int array1[arraySize] = {1, 2, 3, 4, 5};
int array2[arraySize] = {1, 2, 3, 4, 5};
int array3[arraySize] = {1, 2, 3, 4, 6};
bool isEqual1 =
std::equal(array1, array1 + arraySize, array2, array2 + arraySize);
bool isEqual2 =
std::equal(array1, array1 + arraySize, array3, array3 + arraySize);
if (isEqual1) {
cout << "Arrays array1 and array2 are the same." << endl;
} else {
cout << "Arrays array1 and array2 are not the same." << endl;
}
if (isEqual2) {
cout << "Arrays array1 and array3 are the same." << endl;
} else {
cout << "Arrays array1 and array3 are not the same." << endl;
}
return 0;
}
In this code example, std::equal
is used to compare the elements of two arrays. The first range is defined by the iterators array1
and array1 + arraySize
.
The second range is defined by the iterators array2
and array2 + arraySize
. The result is stored in isEqual1
.
The same process is repeated for comparing array1
and array3
, with the result stored in isEqual2
. Boolean values are then used to determine if the arrays are equal, and appropriate messages are printed to the console.
Code Output:
This illustrates how std::equal
provides a concise and effective way to compare arrays in C++, allowing for clear and concise code.
Compare Arrays in C++ Using the std::memcmp
Function
When it comes to comparing arrays in C++, the std::memcmp
function from the <cstring>
header provides another efficient solution. Unlike element-wise comparisons, std::memcmp
performs a memory comparison of the arrays.
The syntax for using std::memcmp
involves passing the pointers to the beginning of the arrays and their respective sizes. Here’s a basic overview:
#include <cstring>
int result = std::memcmp(ptr1, ptr2, size);
In this structure, ptr1
and ptr2
are pointers to the beginning of the first and second arrays. The size
is the size of the arrays in bytes.
The result
is an integer that indicates the relationship between the memory contents. The return value of std::memcmp
is zero if the memory contents are equal, less than zero if the first array is lexicographically less than the second, and greater than zero if the first array is lexicographically greater than the second.
Let’s explore a complete working example of comparing arrays using std::memcmp
:
#include <cstring>
#include <iostream>
using namespace std;
int main() {
const size_t arraySize = 5;
int array1[arraySize] = {1, 2, 3, 4, 5};
int array2[arraySize] = {1, 2, 3, 4, 5};
int array3[arraySize] = {1, 2, 3, 4, 6};
int result1 = std::memcmp(array1, array2, arraySize * sizeof(int));
int result2 = std::memcmp(array1, array3, arraySize * sizeof(int));
if (result1 == 0) {
cout << "Arrays array1 and array2 are the same." << endl;
} else {
cout << "Arrays array1 and array2 are not the same." << endl;
}
if (result2 == 0) {
cout << "Arrays array1 and array3 are the same." << endl;
} else {
cout << "Arrays array1 and array3 are not the same." << endl;
}
return 0;
}
In the code example above, std::memcmp
is used to perform a memory comparison of the arrays. The result is stored in result1
for comparing array1
and array2
and in result2
for comparing array1
and array3
.
The size of the arrays is provided in bytes by multiplying arraySize
with sizeof(int)
. The results are then checked, and appropriate messages are printed to the console.
Code Output:
This demonstrates how std::memcmp
provides an efficient way to compare arrays by examining their memory contents, offering a powerful alternative to element-wise comparisons.
Compare Arrays in C++ Using the std::vector
Comparison
In C++, when working with arrays, the std::vector
container offers a convenient way to compare arrays directly. This approach simplifies the comparison process, especially when dealing with dynamic array sizes.
Using std::vector
for array comparison involves utilizing the built-in ==
operator provided by the std::vector
class. The syntax is straightforward:
array1 == array2
In this structure, ==
is the equality operator for std::vector
.
The array1
and array2
are std::vector
instances. The comparison is performed directly using the ==
operator.
Let’s explore a complete working example of comparing arrays using std::vector
:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> array1 = {1, 2, 3, 4, 5};
vector<int> array2 = {1, 2, 3, 4, 5};
vector<int> array3 = {1, 2, 3, 4, 6};
if (array1 == array2) {
cout << "Arrays array1 and array2 are the same." << endl;
} else {
cout << "Arrays array1 and array2 are not the same." << endl;
}
if (array1 == array3) {
cout << "Arrays array1 and array3 are the same." << endl;
} else {
cout << "Arrays array1 and array3 are not the same." << endl;
}
return 0;
}
Here, we declare three std::vector<int>
instances: array1
, array2
, and array3
. The ==
operator is used to directly compare the contents of array1
and array2
, as well as array1
and array3
.
Messages are then printed to the console based on the comparison results.
Keep in mind that the ==
operator checks for element-wise equality, meaning both vectors must have the same size, and corresponding elements must be equal.
If the order of elements matters, this is the correct approach. If the order doesn’t matter, you might need to sort the vectors first or use other methods depending on your specific requirements.
Code Output:
This demonstrates how using std::vector
for array comparison in C++ simplifies the code and provides a straightforward approach to checking array equality.
Compare Arrays in C++ Using the std::mismatch
Algorithm
The <algorithm>
header provides the std::mismatch
algorithm, which offers another versatile way to compare arrays element-wise. This algorithm identifies the first mismatched pair of elements in two arrays, providing insights into their differences.
The syntax for using std::mismatch
involves providing the iterators representing the beginning and end of the ranges to be compared. The syntax for std::mismatch
is as follows:
cppCopy codetemplate<class InputIt1, class InputIt2>
std::pair<InputIt1, InputIt2> mismatch(InputIt1 first1, InputIt1 last1,
InputIt2 first2);
Parameters:
first1
: Iterator pointing to the beginning of the first range.last1
: Iterator pointing to the end of the first range.first2
: Iterator pointing to the beginning of the second range.
The function returns a std::pair
of iterators. The first
member of the pair points to the first element in the first range where a difference is found, and the second
member of the pair points to the corresponding element in the second range.
If no differences are found, both iterators will be equal to their respective last
positions.
Keep in mind that the ranges must be of the same length for a meaningful comparison. If the ranges are of different lengths, the behavior is undefined.
Let’s explore a complete working example of comparing arrays using std::mismatch
:
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> array1 = {1, 2, 3, 4, 5};
vector<int> array2 = {1, 2, 3, 4, 6};
auto mismatchPair =
std::mismatch(array1.begin(), array1.end(), array2.begin());
if (mismatchPair.first == array1.end()) {
cout << "Arrays array1 and array2 are the same." << endl;
} else {
cout << "Arrays array1 and array2 are not the same." << endl;
cout << "First mismatch at position "
<< distance(array1.begin(), mismatchPair.first) << endl;
}
return 0;
}
In this example, we declare two std::vector<int>
instances: array1
and array2
. std::mismatch
is used to find the first mismatched pair between the two arrays.
The result is stored in mismatchPair
, which is a pair of iterators. If mismatchPair.first
equals array1.end()
, it means the arrays are the same. Otherwise, we print a message indicating the first mismatch and its position.
Code Output:
This demonstrates how std::mismatch
in C++ can be utilized to pinpoint the first difference between two arrays, providing valuable information for debugging and analysis.
Conclusion
C++ offers multiple methods for comparing arrays, each suited to different scenarios and preferences. The for
loop statement provides a simple and direct approach, while custom-defined functions allow for flexibility and customization.
Leveraging the standard library, std::equal
and std::memcmp
offer concise solutions, with std::mismatch
providing detailed insights into differences. Additionally, using the std::vector
class streamlines array comparison, especially when dynamic sizes are involved.
Depending on the specific needs of the task at hand, you can choose the most suitable method, combining clarity, efficiency, and adaptability in their array comparison endeavors.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook