Confronta gli array in C++
-
Usa l’istruzione del cicli
for
per confrontare gli array in C++ - Usa la funzione definita personalizzata per confrontare gli array in C++
-
Usa l’algoritmo
std::equal
per confrontare gli array in C++
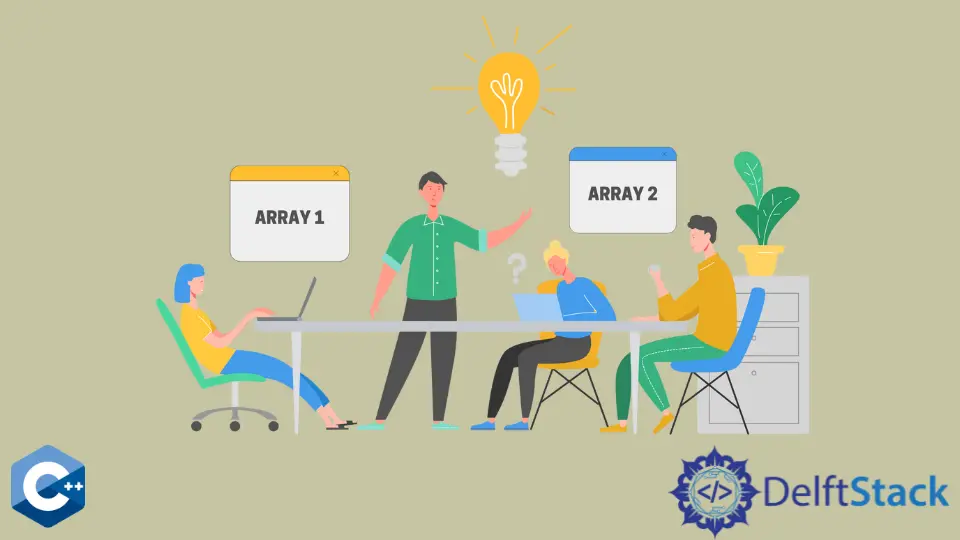
In questo articolo verranno illustrati più metodi per confrontare gli array in C++.
Usa l’istruzione del cicli for
per confrontare gli array in C++
In questi esempi, utilizzeremo un contenitore di array di variabili - std::vector
. Questa classe ha un operatore incorporato ==
che possiamo usare per confrontare i contenuti dei due vettori dati. In questo caso, non dobbiamo preoccuparci delle diverse lunghezze dei vettori, poiché sono gestite internamente dal metodo. Il valore restituito è booleano con true
, che implica l’uguaglianza di due vettori.
Nota che valutiamo l’espressione con ? :
l’istruzione condizionale e stampa i messaggi di stringa corrispondenti nella console.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> i_vec1 = {12, 32, 43, 53, 23, 65};
vector<int> i_vec2 = {12, 32, 43, 53, 23, 25};
vector<int> i_vec3 = {12, 32, 43, 53, 23, 65};
i_vec1 == i_vec2
? cout << "Vectors i_vec1 and i_vec2 are the same" << endl
: cout << "Vectors i_vec1 and i_vec2 are not the same" << endl;
i_vec1 == i_vec3
? cout << "Vectors i_vec1 and i_vec3 are the same" << endl
: cout << "Vectors i_vec1 and i_vec3 are not the same" << endl;
return EXIT_SUCCESS;
}
Produzione:
Vectors i_vec1 and i_vec2 are not the same
Vectors i_vec1 and i_vec3 are the same
Usa la funzione definita personalizzata per confrontare gli array in C++
Il metodo precedente può essere generalizzato nella funzione modello dell’utente e, se necessario, è possibile aggiungere codici di ritorno personalizzati e gestione delle eccezioni. In questo esempio, abbiamo implementato la funzione compareVectorContents
che accetta due riferimenti vettoriali
e valuta la condizione di uguaglianza utilizzando la struttura if
.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
template <typename T>
bool compareVectorContents(vector<T> &vec1, vector<T> &vec2) {
if (vec1 == vec2) {
return true;
} else {
return false;
}
}
int main() {
vector<int> i_vec1 = {12, 32, 43, 53, 23, 65};
vector<int> i_vec3 = {12, 32, 43, 53, 23, 65};
vector<int> i_vec4 = {12, 32, 43};
compareVectorContents(i_vec1, i_vec3)
? cout << "Vectors i_vec1 and i_vec3 are the same" << endl
: cout << "Vectors i_vec1 and i_vec3 are not the same" << endl;
compareVectorContents(i_vec1, i_vec4)
? cout << "Vectors i_vec1 and i_vec4 are the same" << endl
: cout << "Vectors i_vec1 and i_vec4 are not the same" << endl;
return EXIT_SUCCESS;
}
Vectors i_vec1 and i_vec3 are the same
Vectors i_vec1 and i_vec4 are not the same
Usa l’algoritmo std::equal
per confrontare gli array in C++
Un altro metodo per confrontare i contenuti di due vettori
è l’algoritmo std::equal
dalla libreria standard C++, definito nel file di intestazione <algorithm>
. Il metodo equal
prende 4 parametri che rappresentano 2 intervalli separati che devono essere confrontati, fornendo così un’interfaccia più generica per gestire i confronti. Questo metodo può essere impiegato su intervalli formati con iteratori da contenitori non ordinati, vale a dire - std::unordered_set
, std::unordered_map
ecc. Il valore di ritorno equal
è booleano e restituisce true
se gli elementi sono gli stessi in due intervalli. Inoltre, la funzione restituirà false
se gli intervalli passati differiscono in lunghezza.
#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::equal;
using std::string;
using std::vector;
int main() {
vector<int> i_vec1 = {12, 32, 43, 53, 23, 65};
vector<int> i_vec2 = {12, 32, 43, 53, 23, 65};
vector<int> i_vec3 = {12, 32, 43};
equal(i_vec1.begin(), i_vec1.end(), i_vec2.begin(), i_vec2.end())
? cout << "Vectors i_vec1 and i_vec2 are the same" << endl
: cout << "Vectors i_vec1 and i_vec2 are not the same" << endl;
equal(i_vec1.begin(), i_vec1.end(), i_vec3.begin(), i_vec3.end())
? cout << "Vectors i_vec1 and i_vec3 are the same" << endl
: cout << "Vectors i_vec1 and i_vec3 are not the same" << endl;
return EXIT_SUCCESS;
}
Produzione:
Vectors i_vec1 and i_vec2 are the same
Vectors i_vec1 and i_vec3 are not the same
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook