How to Compare Two Strings Ignoring the Case in C++
-
Use the
strcasecmp
Function to Compare Two Strings Ignoring the Case -
Use the
strncasecmp
Function to Compare Two Strings Ignoring the Case -
Use Custom
toLower
Function and==
Operator to Compare Two Strings Ignoring the Case -
Use the
std::transform
Withstd::equal
Function to Compare Two Strings Ignoring the Case - Conclusion
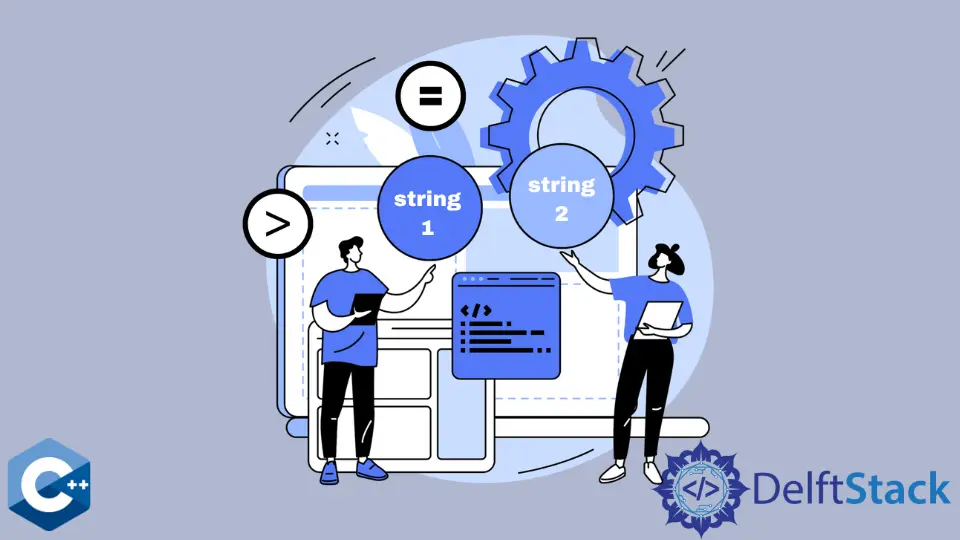
In C++, comparing two strings while disregarding the distinction between uppercase and lowercase characters is a common necessity. This need arises in situations where case differences should not affect the equality of strings, such as user inputs or file processing.
To address this, we’ll demonstrate multiple methods of how to compare two strings while ignoring the case of letters in C++.
Use the strcasecmp
Function to Compare Two Strings Ignoring the Case
The strcasecmp
function is a standard C library function designed for case-insensitive string comparison. It directly compares two strings without the need for custom logic, providing a straightforward and efficient approach.
Code Example:
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text1 = "Hey! Mr. Tambourine man, play a song for me";
string text2 = "hey! Mr. Tambourine man, PLAY a song for me";
string text3 = "Hey! Mrs. Tambourine man, play a song for me";
if (strcasecmp(text1.c_str(), text2.c_str()) == 0) {
cout << "strings: text1 and text2 match." << endl;
} else {
cout << "strings: text1 and text2 don't match!" << endl;
}
if (strcasecmp(text1.c_str(), text3.c_str()) == 0) {
cout << "strings: text1 and text3 match." << endl;
} else {
cout << "strings: text1 and text3 don't match!" << endl;
}
return EXIT_SUCCESS;
}
In this code, we compare three strings using the strcasecmp
function, a standard C library function for case-insensitive string comparison.
In the first conditional statement, we compare text1
and text2
. In the second conditional statement, we compare text1
and text3
.
Output:
strings: text1 and text2 match.
strings: text1 and text3 don't match!
In the output, we can see that the strings text1
and text2
match, as indicated above, "strings: text1 and text2 match."
. However, due to the case difference between "Mr."
and "Mrs."
in text1
and text3
, the comparison results in a mismatch, leading to the output, "strings: text1 and text3 don't match!"
.
Use the strncasecmp
Function to Compare Two Strings Ignoring the Case
Similar to strcasecmp
, the strncasecmp
function performs a case-insensitive comparison. It allows specifying the number of characters to compare, offering flexibility for partial string comparisons.
Code Example:
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
constexpr int BYTES_TO_COMPARE = 5;
int main() {
string text1 = "Hey! Mr. Tambourine man, play a song for me";
string text3 = "hey! Mrs. Tambourine man, PLAY a song for me";
if (strncasecmp(text1.c_str(), text3.c_str(), BYTES_TO_COMPARE) == 0) {
printf("The first %d characters of strings: text1 and text3 match.\n",
BYTES_TO_COMPARE);
}
return EXIT_SUCCESS;
}
In this code, we use the strncasecmp
function to compare the first five characters of two strings, text1
and text3
. These strings contain variations of the lyrics "Hey! Mr. Tambourine man, play a song for me"
and "hey! Mrs. Tambourine man, PLAY a song for me"
.
The if
statement checks if the first 5 characters of both strings match, disregarding case differences.
Output:
The first 5 characters of strings: text1 and text3 match.
The code outputs a message "The first 5 characters of strings: text1 and text3 match."
indicating that the initial characters of text1
and text3
match.
Use Custom toLower
Function and ==
Operator to Compare Two Strings Ignoring the Case
This method involves a custom toLower
function to convert characters to lowercase before comparison using the ==
operator. It provides explicit control over the comparison logic, offering customization when needed.
Code Example:
#include <algorithm>
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
std::string toLower(std::string s) {
std::transform(s.begin(), s.end(), s.begin(),
[](unsigned char c) { return std::tolower(c); });
return s;
}
int main() {
string text1 = "Hey! Mr. Tambourine man, play a song for me";
string text2 = "Hey! Mr. Tambourine man, play a song for me";
string text3 = "Hey! Mrs. Tambourine man, play a song for me";
if (toLower(text1) == toLower(text2)) {
cout << "strings: text1 and text2 match." << endl;
} else {
cout << "strings: text1 and text2 don't match!" << endl;
}
if (toLower(text1) == toLower(text3)) {
cout << "strings: text1 and text3 match." << endl;
} else {
cout << "strings: text1 and text3 don't match!" << endl;
}
return EXIT_SUCCESS;
}
In this code, we define a toLower
function that transforms a given string to lowercase using std::transform
. The main function initializes three strings with variations of the lyrics "Hey! Mr. Tambourine man, play a song for me"
.
The code then utilizes the toLower
function to convert the strings to lowercase and performs case-insensitive comparisons.
Output:
strings: text1 and text2 match.
strings: text1 and text3 don't match!
The output messages indicate whether the first two strings (text1
and text2
) match and whether text1
and text3
match, providing clear information about the case-insensitive matching status between the pairs of strings.
Use the std::transform
With std::equal
Function to Compare Two Strings Ignoring the Case
By using the std::transform
algorithm along with std::equal
, this method achieves case-insensitive comparison. A lambda function is employed to convert characters to lowercase before comparing them, providing a concise and readable solution.
Code Example:
#include <algorithm>
#include <cctype>
#include <iostream>
#include <string>
bool compareIgnoreCase1(const std::string& str1, const std::string& str2) {
if (str1.size() != str2.size()) {
return false;
}
return std::equal(str1.begin(), str1.end(), str2.begin(), [](char a, char b) {
return std::tolower(a) == std::tolower(b);
});
}
int main() {
std::string text1 = "Hey! Mr. Tambourine man, play a song for me";
std::string text2 = "hey! Mr. Tambourine man, PLAY a song for me";
if (compareIgnoreCase1(text1, text2)) {
std::cout << "Method 1: Strings are equal (ignoring case)." << std::endl;
} else {
std::cout << "Method 1: Strings are not equal (ignoring case)."
<< std::endl;
}
return 0;
}
In this code, we’ve made a way to compare two sentences while ignoring whether the letters are big or small. We created a method called compareIgnoreCase1
.
It checks if two sentences are the same, even if the letters are different cases.
Output:
Method 1: Strings are equal (ignoring case).
The code outputs a message "Method 1: Strings are equal (ignoring case)."
indicating the success of our method in making a case-insensitive comparison.
Conclusion
In this article, we delve into the task of comparing two strings in C++ while disregarding differences in uppercase and lowercase characters. The necessity for such comparisons is eloquently established, particularly in scenarios like user inputs or file processing where case distinctions should not influence string equality.
The article presents four methods for achieving case-insensitive string comparison, showcasing their implementations with code examples and the corresponding output. The methods range from using standard C library functions like strcasecmp
and strncasecmp
for simplicity and efficiency to more customized approaches like employing a custom toLower
function combined with the ==
operator or leveraging std::transform
with std::equal
for a concise and readable solution.
Each method is thoughtfully explained, offering developers a diverse set of tools to address their specific needs. In essence, the article empowers C++ developers with a comprehensive guide to navigate and choose the most suitable method for their unique case-insensitive string comparison requirements.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++