Confronta due stringhe ignorando il caso in C++
-
Usa la funzione
strcasecmp
per confrontare due stringhe ignorando il caso -
Usa la funzione
strncasecmp
per confrontare due stringhe ignorando il caso -
Usa la funzione personalizzata
toLower
e l’operatore==
per confrontare due stringhe ignorando il caso
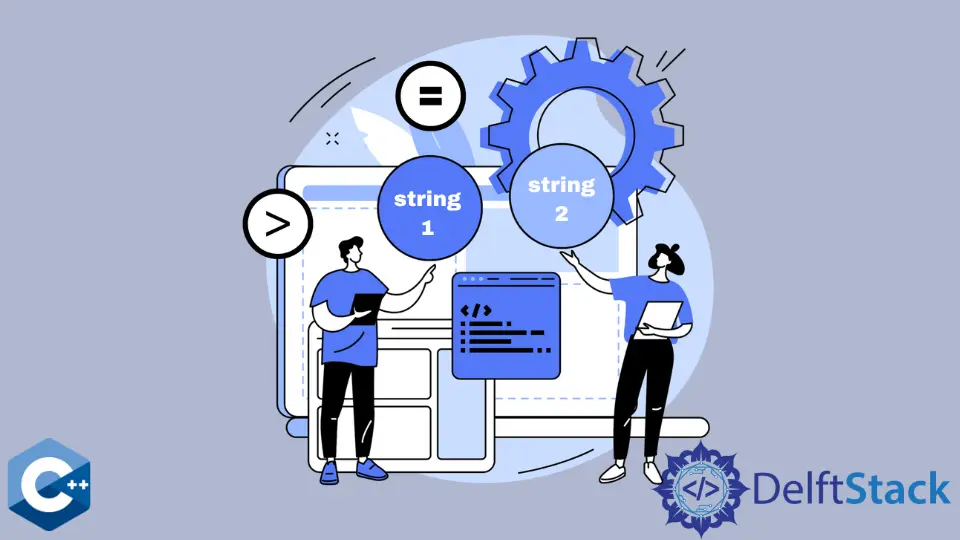
Questo articolo illustrerà più metodi su come confrontare due stringhe ignorando le maiuscole e minuscole in C++.
Usa la funzione strcasecmp
per confrontare due stringhe ignorando il caso
strcasecmp
è la funzione della libreria standard C che può essere inclusa nel file sorgente C++ usando l’intestazione <cstring>
. La funzione stessa opera byte per byte e restituisce un numero intero minore o uguale o maggiore di 0, come valutano le stringhe corrispondenti.
Vale a dire, se le due stringhe ignorate sono uguali, il valore restituito è zero. In altri scenari, quando vengono trovati i primi caratteri diversi, vengono confrontati con i valori nella loro posizione alfabetica e viene restituito il risultato corrispondente.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text1 = "Hey! Mr. Tambourine man, play a song for me";
string text2 = "hey! Mr. Tambourine man, PLAY a song for me";
string text3 = "Hey! Mrs. Tambourine man, play a song for me";
if (strcasecmp(text1.c_str(), text2.c_str()) == 0) {
cout << "strings: text1 and text2 match." << endl;
} else {
cout << "strings: text1 and text2 don't match!" << endl;
}
if (strcasecmp(text1.c_str(), text3.c_str()) == 0) {
cout << "strings: text1 and text3 match." << endl;
} else {
cout << "strings: text1 and text3 don't match!" << endl;
}
return EXIT_SUCCESS;
}
Produzione:
strings: text1 and text2 match.
strings: text1 and text3 don't match!
Usa la funzione strncasecmp
per confrontare due stringhe ignorando il caso
strncasecmp
è un’altra variazione della funzione precedente che può essere utilizzata per confrontare un dato numero di caratteri da due stringhe ignorando il caso. La funzione prende il valore intero per il numero massimo di caratteri che devono essere confrontati dal primo char
. L’esempio seguente mostra come confrontare i primi 5 caratteri di due stringhe ignorando il caso.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
constexpr int BYTES_TO_COMPARE = 5;
int main() {
string text1 = "Hey! Mr. Tambourine man, play a song for me";
string text3 = "hey! Mrs. Tambourine man, PLAY a song for me";
if (strncasecmp(text1.c_str(), text3.c_str(), BYTES_TO_COMPARE) == 0) {
printf("The first %d characters of strings: text1 and text3 match.\n",
BYTES_TO_COMPARE);
}
return EXIT_SUCCESS;
}
Produzione:
The first 5 characters of strings: text1 and text3 match.
Usa la funzione personalizzata toLower
e l’operatore ==
per confrontare due stringhe ignorando il caso
In alternativa, possiamo convertire le stringhe nello stesso caso e quindi utilizzare un semplice operatore ==
per il confronto. Come scelta arbitraria, questo esempio offre l’abbassamento di entrambe le stringhe con una funzione definita dall’utente, che restituisce l’oggetto stringa
. Infine, l’istruzione if
può contenere l’espressione di confronto.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
std::string toLower(std::string s) {
std::transform(s.begin(), s.end(), s.begin(),
[](unsigned char c) { return std::tolower(c); });
return s;
}
int main() {
string text1 = "Hey! Mr. Tambourine man, play a song for me";
string text2 = "Hey! Mr. Tambourine man, play a song for me";
string text3 = "Hey! Mrs. Tambourine man, play a song for me";
if (toLower(text1) == toLower(text2)) {
cout << "strings: text1 and text2 match." << endl;
} else {
cout << "strings: text1 and text2 don't match!" << endl;
}
if (toLower(text1) == toLower(text3)) {
cout << "strings: text1 and text3 match." << endl;
} else {
cout << "strings: text1 and text3 don't match!" << endl;
}
return EXIT_SUCCESS;
}
Produzione:
strings: text1 and text2 match.
strings: text1 and text3 don't match!
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook