C++ で大文字小文字を無視して 2つの文字列を比較する
胡金庫
2023年10月12日
-
大文字小文字を無視して 2つの文字列を比較するには
strcasecmp
関数を使用する -
大文字小文字を無視した 2つの文字列を比較するには
strncasecmp
関数を使用する -
カスタムの
toLower
関数と==
演算子を用いて大文字小文字を無視して 2つの文字列を比較する
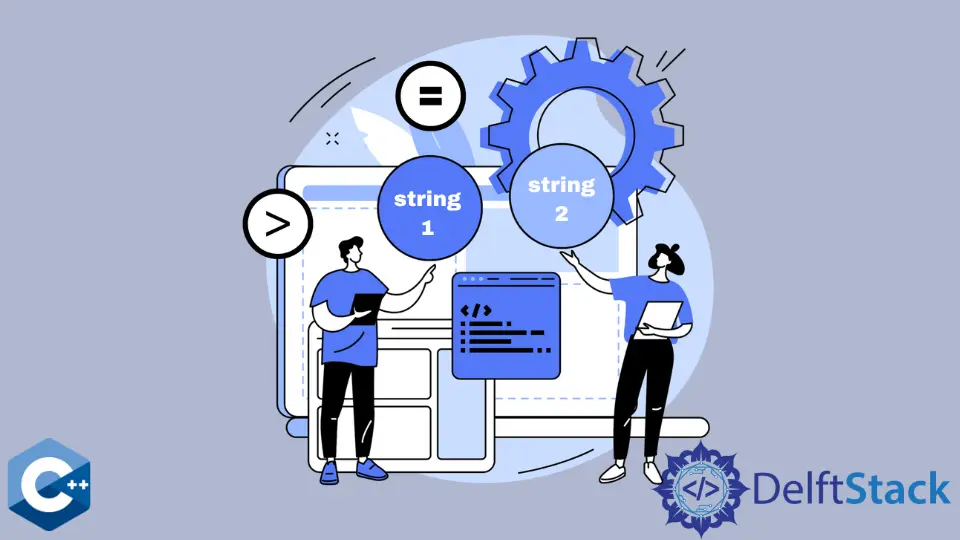
この記事では、C++ で文字の大文字小文字を無視して 2つの文字列を比較する方法について、複数の方法を紹介します。
大文字小文字を無視して 2つの文字列を比較するには strcasecmp
関数を使用する
strcasecmp
は C 標準ライブラリ関数であり、<cstring>
ヘッダを用いて C++ のソースファイルに含めることができます。この関数自体はバイト単位で動作し、対応する文字列の評価に応じて 0 以下か 0 以上の整数を返します。
つまり、無視された 2つの文字列が等しい場合、戻り値は 0
です。他のシナリオでは、最初の異なる文字が見つかった場合、そのアルファベットの位置まで値と比較され、対応する結果が返されます。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text1 = "Hey! Mr. Tambourine man, play a song for me";
string text2 = "hey! Mr. Tambourine man, PLAY a song for me";
string text3 = "Hey! Mrs. Tambourine man, play a song for me";
if (strcasecmp(text1.c_str(), text2.c_str()) == 0) {
cout << "strings: text1 and text2 match." << endl;
} else {
cout << "strings: text1 and text2 don't match!" << endl;
}
if (strcasecmp(text1.c_str(), text3.c_str()) == 0) {
cout << "strings: text1 and text3 match." << endl;
} else {
cout << "strings: text1 and text3 don't match!" << endl;
}
return EXIT_SUCCESS;
}
出力:
strings: text1 and text2 match.
strings: text1 and text3 don't match!
大文字小文字を無視した 2つの文字列を比較するには strncasecmp
関数を使用する
strncasecmp
は上記の関数の別のバリエーションであり、大文字小文字を無視して 2つの文字列から指定された数の文字を比較するために利用することができます。この関数は、最初の char
から比較する必要がある最大文字数の整数値を取ります。次の例は、大文字小文字を無視して 2つの文字列の最初の 5 文字を比較する方法を示しています。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
constexpr int BYTES_TO_COMPARE = 5;
int main() {
string text1 = "Hey! Mr. Tambourine man, play a song for me";
string text3 = "hey! Mrs. Tambourine man, PLAY a song for me";
if (strncasecmp(text1.c_str(), text3.c_str(), BYTES_TO_COMPARE) == 0) {
printf("The first %d characters of strings: text1 and text3 match.\n",
BYTES_TO_COMPARE);
}
return EXIT_SUCCESS;
}
出力:
The first 5 characters of strings: text1 and text3 match.
カスタムの toLower
関数と ==
演算子を用いて大文字小文字を無視して 2つの文字列を比較する
あるいは、文字列を同じケースに変換して、単純な ==
演算子を使って比較することもできます。任意の選択肢として、この例ではユーザ定義の関数を用いて両方の文字列を下げることができます。最後に、if
文には比較式を含めることができます。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
std::string toLower(std::string s) {
std::transform(s.begin(), s.end(), s.begin(),
[](unsigned char c) { return std::tolower(c); });
return s;
}
int main() {
string text1 = "Hey! Mr. Tambourine man, play a song for me";
string text2 = "Hey! Mr. Tambourine man, play a song for me";
string text3 = "Hey! Mrs. Tambourine man, play a song for me";
if (toLower(text1) == toLower(text2)) {
cout << "strings: text1 and text2 match." << endl;
} else {
cout << "strings: text1 and text2 don't match!" << endl;
}
if (toLower(text1) == toLower(text3)) {
cout << "strings: text1 and text3 match." << endl;
} else {
cout << "strings: text1 and text3 don't match!" << endl;
}
return EXIT_SUCCESS;
}
出力:
strings: text1 and text2 match.
strings: text1 and text3 don't match!
著者: 胡金庫