Arithmetic Operations on Fractions Using Constructor in C++
- Arithmetic Operations on Fractions Using Constructor in C++
- Declare a Constructor and Initialize its Data Members in C++
- Implement the Member Methods to Perform Operations in C++
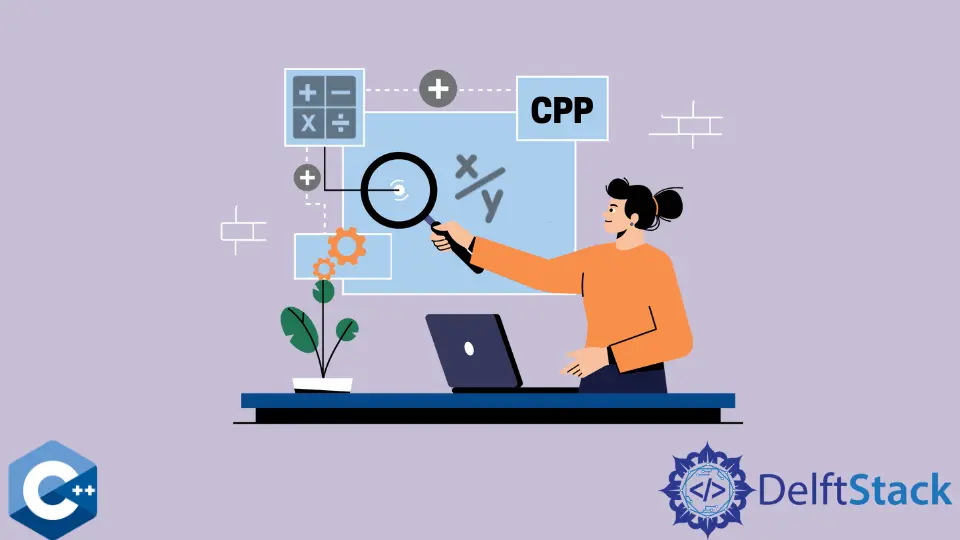
This article will discuss how to create fractions using constructors, find GCD to reduce fraction multiples, and perform computations on them.
Arithmetic Operations on Fractions Using Constructor in C++
The reader must understand the prerequisites of the program. To create a fraction using constructor,
- the denominator must not be zero;
- if the numerator and denominator are both divisible with each other, then it must be reduced to its lowest multiple;
- the program must be designed so that the result is stored and displayed through a new data member, while pre-existing variables must not change.
These conditions will make the program feasible and capable of computing fractions correctly.
Declare a Constructor and Initialize its Data Members in C++
The constructor interacts with different member methods and passes values to each other. The first step for any constructor program is to load the import packages and declare default constructors and parameterized constructors.
-
Import package.
#include <iostream>
-
The member method
reduce()
will be used to reduce the fraction to its lowest multiple.int reduce(int m, int n);
-
The
namespace std
is used for theiostream
package.using namespace std;
-
The class name must have the same name as member methods.
class computefraction
-
The default constructor
private
: has two data members for the numerator and denominator of the fraction.{ private: int topline; int bottomline;
-
The parameterized constructor
public:
is declared after that. Data members of the default constructor are initialized here with two new variables with the same data type(int tl = 0, int bl = 1)
.
The constructor class has five-member methods. The first one. computefraction()
, shares the same name as the class name and stores the values of fractions both before and after reduction.
The reduction of fractions needs to occur before the values are passed to other member methods. Four member methods are declared for 4 operator computations - addition, subtraction, multiplication, and division.
Two other member methods declared read the fractions and print them.
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
Below is the representation of the first part of the program.
#include <iostream>
int reduce(int m, int n);
using namespace std;
class computefraction {
private:
int topline;
int bottomline;
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
};
Implement the Member Methods to Perform Operations in C++
Member methods that perform arithmetic operations on fractions in C++ are implemented in this part of the article.
Fraction Class Operator Overloading in C++
In this program, operators are overloaded in every member method to fit the values of two fractions into a single object. Overloading is done when a user-defined data type is used instead of an in-built data type, and its return type is needed to be changed.
This is achieved by the ::
scope resolution operator. It accesses the local variables of a constructor from a particular class.
The values of the fractions are stored in the constructor computefraction
. The program will pass these values to the other member methods to perform arithmetic operations on them, but first, the fraction needs to be reduced before it can be given to other member methods.
The syntax computefraction::computefraction(int tl, int bl)
is used to access the local variables of constructor computefraction
.
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl)
Similarly, in the member method to add two fractions, operator sum
is overloaded to fit the values of two fractions.
Syntax:
return_method class_name::operator(argument)
This syntax accesses the operator sum
from the class computefraction
, overloads it with copy object b2
, and returns the final result into constructor computefraction
.
computefraction computefraction::sum(computefraction b2) const
Member Method to Find GCD of the Fraction in C++
The function gcd
finds the GCD to reduce fraction multiples. The numerator and denominator are the two data members int m
and int n
.
The function at first checks if the value is negative or positive. If either numerator or denominator has a negative value, it becomes positive using m = (m < 0) ? -m : m;
.
The function compares the higher value among variables m
and n
to find GCD for reducing fraction multiples. If the larger value is stored in variable n
, it swaps with m
.
The function is designed so that the higher value will always get stored in variable m
. The function subtracts the lower value from the higher value until m
is reduced to zero.
Lastly, the value left in variable n
is the GCD, and it is returned.
int gcd(int m, int n) {
m = (m < 0) ? -m : m;
n = (n < 0) ? -n : n;
while (m > 0) {
if (m < n) {
int bin = m;
m = n;
n = bin;
}
m -= n;
}
return n;
}
Member method to Reduce Numerator and Denominator of A Fraction in C++
Fractions are reduced by dividing numerator and denominator by its GCD.
Here two variables are initialized, topline
for the numerator and bottomline
for the denominator.
An if-else
condition checks the variable bottomline
values. If the values are zero, the program exits with an error message.
An integer variable reduce
is initialized to store the GCD of the two numbers returned from method gcd
.
Then, the value stored in reduce
is divided from both topline
and bottomline
, and the result is stored back to the same variables.
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl) {
if (bl == 0) {
cout << "You cannot put 0 as a denominator" << endl;
exit(0); // program gets terminated
} else
bottomline = bl;
int reduce = gcd(topline, bottomline);
topline /= reduce;
bottomline /= reduce;
}
Create Member Method to Add Two Fractions in C++
All the methods used for computation operations create a copy object like a copy constructor. That is done by the syntax computefraction computefraction::sum(bounty b2) const
.
The keyword bounty b2
creates a copy object b2
. Making a copy object is to reuse objects for the second fraction without initializing extra variables.
The equation to add two fractions a/b and c/d is,
Variable int tl
stores the result of the numerator, while int bl
stores the result of the denominator. Finally, tl
and bl
are passed.
computefraction computefraction::sum(bounty b2) const {
int tl = topline * b2.bottomline + b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
The other member methods are created in the same way. To check, the reader can go to the final program in this article.
How to Implement the Main Function Using Constructor in C++
Once all the member methods are created, the main()
function needs to be written so that the program can return the passed values and display them.
This program must be written in a way that the end-user is given choices and responses in this order:
- Option to choose the operator, i.e., addition, subtraction, etc.
- Throw an exception if the choice is out of order and the program reloads.
- When given a correct choice, the user is asked to enter the numerator and denominator of the first fraction, followed by the second fraction.
- Throws error exception if a zero is inserted in the denominator and exits.
- If inputs are correct, the program gives the result as a final response.
- The program reloads back to step 1.
Initialization of Local Variables and Constructor Objects for Main Function
As a menu-driven program, a char variable ch
is declared. Three objects are declared: up
to pass and store the first fraction, down
to hold the second fraction, and final
to display the result of the two fractions.
int main() {
char ch;
computefraction up;
computefraction down;
computefraction final;
}
Implement Menu-Driven Conditions Using a Do While
Loop
The program uses 5 menu-driven cases and a default case under a do-while
loop. The first four cases perform computation operations, while the fifth one is reserved for an exit option.
The default
will be defined for out-of-scope choices. The program runs in a loop until the user chooses the fifth case to break out of it and end the program.
do {
switch (ch) {
case '1':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
... case '5':
break;
default : cerr << "Choice is out of scope" << ch << endl;
break;
}
} while (ch != '5');
Complete Code to Compute Fractions Using Constructor in C++
// import package
#include <iostream>
// member method to find gcd, with two data members
int gcd(int m, int n);
using namespace std;
class computefraction {
// Default constructor
private:
int topline;
int bottomline;
// Parameterized Constructor
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
};
// Member methods of class type bounty.
// In constructors, the class and constructor names must be the same.
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl) {
if (bl == 0) {
cout << "You cannot put 0 as a denominator" << endl;
exit(0); // program gets terminated
} else
bottomline = bl;
// Below codes reduce the fractions using gcd
int reduce = gcd(topline, bottomline);
topline /= multiple;
bottomline /= multiple;
}
// Constructor to add fractions
computefraction computefraction::sum(computefraction b2) const {
int tl = topline * b2.bottomline + b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to subtract fractions
computefraction computefraction::minus(computefraction b2) const {
int tl = topline * b2.bottomline - b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to multiply fractions
computefraction computefraction::mult(computefraction b2) const {
int tl = topline * b2.topline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to divide fractions
computefraction computefraction::rem(computefraction b2) const {
int tl = topline * b2.bottomline;
int bl = bottomline * b2.topline;
return computefraction(tl, bl);
}
// Method to print output
void computefraction::show() const {
cout << endl << topline << "/" << bottomline << endl;
}
// Method to read input
void computefraction::see() {
cout << "Type the Numerator ";
cin >> topline;
cout << "Type the denominator ";
cin >> bottomline;
}
// GCD is calculated in this method
int gcd(int m, int n) {
m = (m < 0) ? -m : m;
n = (n < 0) ? -n : n;
while (m > 0) {
if (m < n) {
int bin = m;
m = n;
n = bin;
}
m -= n;
}
return n;
}
// Main Function
int main() {
char ch;
computefraction up;
computefraction down;
computefraction final;
do {
cout << " Choice 1\t Sum\n";
cout << " Choice 2\t Minus\n";
cout << " Choice 3\t multiply\n";
cout << " Choice 4\t Divide\n";
cout << " Choice 5\t Close\n";
cout << " \nEnter your choice: ";
cin >> ch;
cin.ignore();
switch (ch) {
case '1':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
case '2':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.minus(down);
final.show();
break;
case '3':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
case '4':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.mult(down);
final.show();
break;
case '5':
break; // exits program.
default:
cerr << "Choice is out of scope" << ch << endl;
break;
}
} while (ch != '5'); // program stops reloading when choice 5 is selected
return 0;
}
Output:
Choice 1 Sum
Choice 2 Minus
Choice 3 multiply
Choice 4 Divide
Choice 5 Close
Enter your choice: 2
first fraction: Type the Numerator 15
Type the denominator 3
Second fraction: Type the Numerator 14
Type the denominator 7
3/1
Choice 1 Sum
Choice 2 Minus
Choice 3 multiply
Choice 4 Divide
Choice 5 Close
Enter your choice: 3
first fraction: Type the Numerator 5
Type the denominator 0
Second fraction: Type the Numerator 6
Type the denominator 8
You cannot put 0 as a denominator
--------------------------------
Process exited after 47.24 seconds with return value 0
Press any key to continue . . .