How to Calculate Exponent Without Using pow() Function in C++
-
Calculate Exponent Without Using the
pow()
Function in C++ -
Use the
for
Loop to Calculate the Exponent Without Using thepow()
Function in C++ -
Use the
while
Loop to Calculate the Exponent Without Using thepow()
Function in C++ -
Use Recursion to Calculate Exponent Without Using the
pow()
Function in C++ - Conclusion
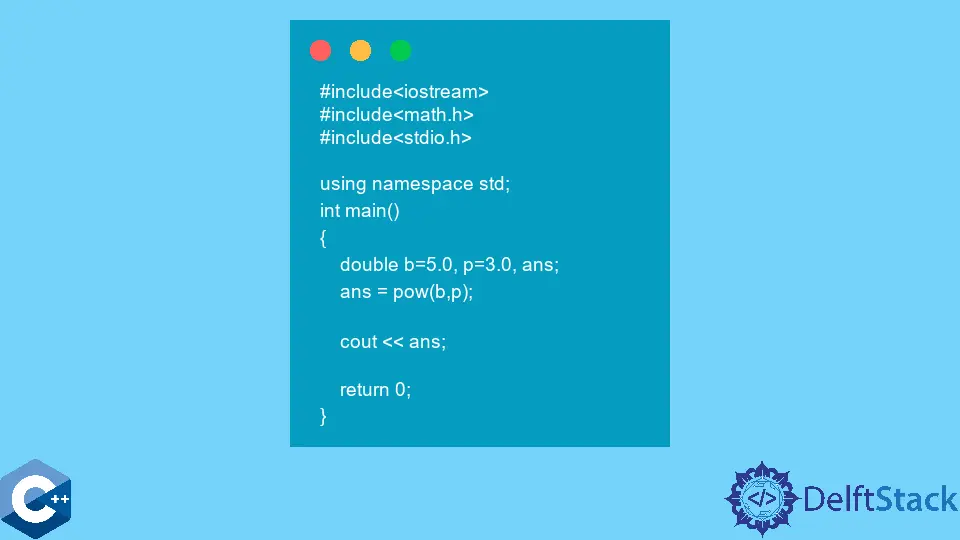
C++, like many other programming languages, C++ comes with an in-built library containing functions and modules to make tasks easier. The pow()
function is one in-built function that lets us easily calculate the power of a number.
However, there might be a situation, like on coding platforms, where you have to find the power of a number without using any in-built functions. Any guesses on how you will do that?
In this article, we will discuss the various ways we can calculate the exponent of a number without using the pow()
function.
Calculate Exponent Without Using the pow()
Function in C++
Before we go on to the alternatives of using the pow()
function, let us first see how the pow()
function works in C++.
the pow()
Function in C++
As the name says, the pow()
or power
function calculates a number’s power. This function takes two values as arguments and requires the use of the header file as well.
The first value that we pass in the pow()
function acts as the base, and the second value is the exponent to which the base has to be raised. Here is a program that calculates the value of 5^3
with the help of the pow()
function.
#include <math.h>
#include <stdio.h>
#include <iostream>
using namespace std;
int main() {
double b = 5.0, p = 3.0, ans;
ans = pow(b, p);
cout << ans;
return 0;
}
Output:
125
Note that the pow()
function takes double
values and not int
as arguments. But this does not mean that the pow()
function can’t be used with integers.
However, sometimes the use of int
with the pow()
function might give absurd outputs in some compilers. For example, pow(4,2)
might give the output as 15
with some compilers.
So, using the pow()
function with double
data types is advisable.
Now that you know the basics of the pow()
function, let us discuss some other ways to calculate the exponent of a number without using the pow()
function in C++.
Use the for
Loop to Calculate the Exponent Without Using the pow()
Function in C++
We know that while calculating the exponent of a number, we use repeated multiplication. The number of times this multiplication is repeated depends on the exponent or the power to which the base is raised.
Here is an example of the same.
5^4 = 5 * 5 * 5 * 5 = 625
We can also apply this concept in programming using the for
loop. Here is the code for the same.
#include <math.h>
#include <stdio.h>
#include <iostream>
using namespace std;
int main() {
int i, e, b, ans = 1;
cout << "Enter the base: " << endl;
cin >> b;
cout << "Enter the exponent: " << endl;
cin >> e;
/*logic to calculate power*/
for (i = 1; i <= e; ++i) {
ans = ans * b;
}
cout << "The solution is: " << ans;
return 0;
}
Output:
Enter the base:
5
Enter the exponent:
4
The solution is: 625
So what’s happening in this code?
The variables b
and e
refer to the base and exponent, respectively. Additionally, the variable ans
holds the value 1
, and the variable i
defines the number of times the for
loop runs.
Now understand that the logic that works with the pow()
function here starts from the for
block in this code. So let us break down what’s happening inside the for
block.
You can see that i
is initialized to 1
, and it goes up to the value of the exponent, which is 4
here. Also, the statement inside the loop multiplies b
with ans
and stores the result in the variable ans
itself.
Here is what the variable ans
contains at each iteration.
i=1
ans = 1*5
ans = 5
i=2
ans = 5*5
ans = 25
i=3
ans = 25*5
ans = 125
i=4
ans = 125*5
ans = 625
And obviously, the final value of the variable ans
is returned as the output when the loop terminates. This is essentially how the for
loop can be used to calculate the exponent of a number without the pow()
function.
Let us now use a while
loop to perform the same.
Use the while
Loop to Calculate the Exponent Without Using the pow()
Function in C++
The basic idea behind using the while
loop to calculate the exponent of a number remains the same as that of the for
loop. The only thing that changes is the method of counting the number of repetitions.
Look at the code given below.
Here in the while
block, the initial value of e
is what the user inputs as the exponent, and with each step, the value decreases by one. In the end, the loop terminates when e
reduces to 0
.
#include <math.h>
#include <stdio.h>
#include <iostream>
using namespace std;
int main() {
int i, e, b, ans = 1;
cout << "Enter the base: " << endl;
cin >> b;
cout << "Enter the exponent: " << endl;
cin >> e;
/*logic to calculate power*/
while (e != 0) {
ans = ans * b;
--e;
}
cout << "The solution is: " << ans;
return 0;
}
Output:
Enter the base:
5
Enter the exponent:
4
The solution is: 625
You can see that the statement inside the while
loop multiplies b
with ans
and stores the result in the variable ans
itself in the same way as was done in the case of the for
loop.
Here is what the variable ans
contains at each iteration.
e = 4
ans = 1 * 5
ans = 5
e = 3
ans = 5 * 5
ans = 25
e = 2
ans = 25 * 5
ans = 125
e = 1
ans = 125 * 5
ans = 625
So this is how you can use the while
loop to calculate the exponent of a number without using the pow()
function.
If you further want to perform any other operation on the output, you can do so as shown in the example below.
#include <math.h>
#include <stdio.h>
#include <iostream>
using namespace std;
int main() {
int i, e, b, ans = 1;
cout << "Enter the base: " << endl;
cin >> b;
cout << "Enter the exponent: " << endl;
cin >> e;
/*logic to calculate power*/
while (e != 0) {
ans = ans * b;
--e;
}
/*adding 500 to the result*/
ans = ans + 500;
cout << "The solution after addition is: " << ans;
return 0;
}
Output:
Enter the base:
5
Enter the exponent:
4
The solution after addition is: 1125
Note that it is advisable to use these methods for calculating the exponent when the base and exponent are ints
. For float
values, use the pow()
function.
The complexity of both these methods is O(n)
, n
being the exponent.
So far, we have discussed the usual looping methods to find the power of a number. Let us now discuss a very interesting recursive solution to do the same.
Use Recursion to Calculate Exponent Without Using the pow()
Function in C++
Look at the code given below.
#include <math.h>
#include <stdio.h>
#include <iostream>
using namespace std;
int product(int a, int b) {
if (b)
return (a + product(a, b - 1));
else
return 0;
}
int calculate_power(int x, int y) {
if (y)
return product(x, calculate_power(x, y - 1));
else
return 1;
}
int main() {
cout << calculate_power(5, 2);
getchar();
return 0;
}
Output:
25
This code uses two functions, product
for repeated multiplication and calculate_power
to find out x^y
.
When the main
block runs, the control goes to the calculate_power
function first. Inside this function, if the value of the exponent y
is 0
, the control goes to the if
block, and the function returns 1
.
Otherwise, the control goes to the if
block, where the function calls the product
function, which recursively calls the calculate_power
function. Also, inside the product
function, when the control goes to the if
block, the product
function is called again recursively.
Here is an illustration that will help you better understand the working of this code.
Coming down: calculate_pow(5,2)
↓
product(5, calculate_pow(5,1))
↓
product(5, calculate_pow(5, 0)
↓
return 1
Going back, Step 1: product(5, 1)
↓
return 5 + product(5, 0)
↓
return 0
↓
return 5+0 = 5
Going back, Step 2: product(5, 5)
↓
5 + product(5, 4)
↓
5 + 5 + product(5, 3)
↓
5 + 5 + 5 + product(5, 2)
↓
5 + 5 + 5 + 5 + product(5, 1)
↓
5 + 5 + 5 + 5 + 5
↓
25
This is how recursion calculates the power of a number, the time complexity being O(n)
where n
is the exponent.
Conclusion
In this article, we discussed how we could calculate the exponent of a number without using the pow()
function in C++. We used for
and while
loops to do this and also saw how we can further perform other calculations once we get the power of a number.
Most interestingly, we discussed a recursive solution to calculate the power of a number and also saw the working of that code with a detailed flowchart.