C++ Cube Root
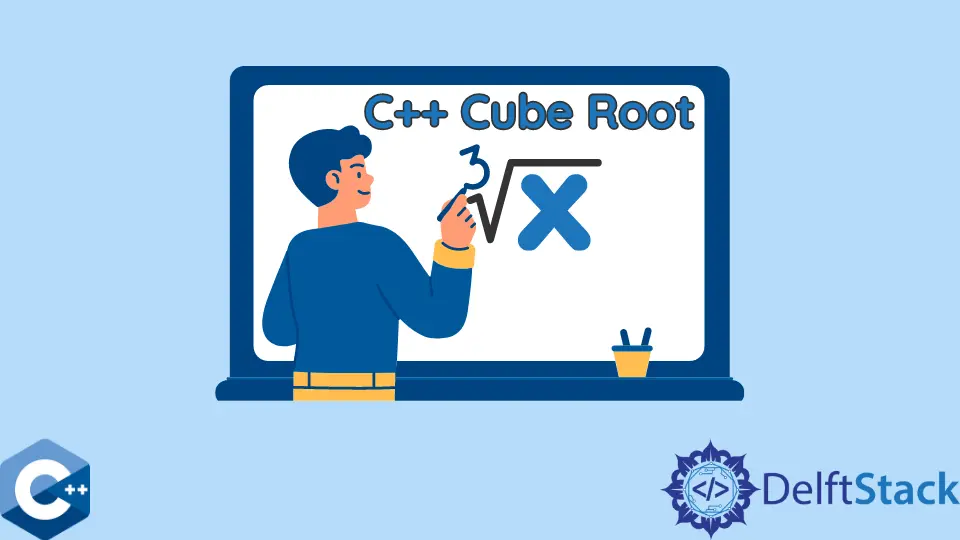
Calculating the cube root of a number is a fundamental operation in mathematics and programming. In C++, you can easily compute the cube root using the pow()
or cbrt()
function from the cmath
library. Whether you’re a beginner looking to grasp the basics of C++ or an experienced programmer seeking to refine your skills, understanding how to compute cube roots is essential.
In this article, we will explore both methods in detail, providing clear examples and explanations to ensure you have a solid grasp of the concepts. Let’s dive into the world of C++ and learn how to efficiently compute cube roots!
Understanding Cube Roots
Before we jump into the methods, let’s clarify what a cube root is. The cube root of a number x
is a value y
such that when y
is multiplied by itself three times (y * y * y), it equals x
. Mathematically, this is expressed as:
y = x^(1/3) or y = cbrt(x)
In C++, you have two main options for calculating the cube root: using the pow()
function or the cbrt()
function. Both methods are effective, but they have different syntaxes and may offer varying performance in specific scenarios.
Using the pow() Function
The pow()
function is a versatile tool for performing exponentiation in C++. To compute the cube root using pow()
, you can raise the number to the power of 1/3
. Here’s how you can do it:
#include <iostream>
#include <cmath>
int main() {
double number = 27.0;
double cubeRoot = pow(number, 1.0 / 3.0);
std::cout << "The cube root of " << number << " is " << cubeRoot << std::endl;
return 0;
}
Output:
The cube root of 27 is 3
In this example, we included the necessary header file, cmath
, to access the pow()
function. We defined a variable number
with a value of 27, which is the number for which we want to find the cube root. Using pow(number, 1.0 / 3.0)
, we calculated the cube root and stored it in the variable cubeRoot
. Finally, we printed the result to the console.
The pow()
function is flexible and can handle various base and exponent values. However, it may be slightly less efficient than the cbrt()
function for cube root calculations specifically, as it performs general exponentiation.
Using the cbrt()
Function
The cbrt()
function, introduced in C++11, is specifically designed for calculating cube roots. This function is straightforward to use and can provide better performance for this specific operation. Here’s an example:
#include <iostream>
#include <cmath>
int main() {
double number = 64.0;
double cubeRoot = cbrt(number);
std::cout << "The cube root of " << number << " is " << cubeRoot << std::endl;
return 0;
}
Output:
The cube root of 64 is 4
In this code snippet, we again included the cmath
header for mathematical functions. We defined a variable number
with a value of 64 and used the cbrt()
function to calculate the cube root, storing it in the cubeRoot
variable. Finally, we printed the result.
Using cbrt()
is often more efficient than using pow()
for cube root calculations, as it is optimized for this specific operation. This can be particularly beneficial in performance-critical applications where you need to compute cube roots frequently.
Conclusion
In summary, calculating the cube root in C++ can be accomplished using either the pow()
or cbrt()
function from the cmath
library. While both methods are effective, the cbrt()
function is more specialized and may offer better performance for cube root calculations. As you continue to explore C++, understanding these mathematical functions will enhance your programming skills and enable you to tackle more complex problems with confidence. Happy coding!
FAQ
-
How do I include the cmath library in my C++ program?
To include the cmath library, simply add#include <cmath>
at the beginning of your program. -
What is the difference between pow() and cbrt() in C++?
Thepow()
function is a general-purpose exponentiation function, whilecbrt()
is specifically designed for calculating cube roots, offering better performance for this operation. -
Can I compute the cube root of negative numbers in C++?
Yes, you can compute the cube root of negative numbers in C++. The result will also be negative, as cube roots of negative numbers yield negative results. -
Is there a performance difference between pow() and cbrt()?
Yes,cbrt()
is generally more efficient for computing cube roots compared topow()
, as it is optimized for that specific calculation.
- Can I use these functions with integers in C++?
Yes, bothpow()
andcbrt()
can be used with integer values, but the result will be returned as a double. If you need an integer result, you can cast the output accordingly.