在 C++ 中使用建構函式對分數進行算術運算
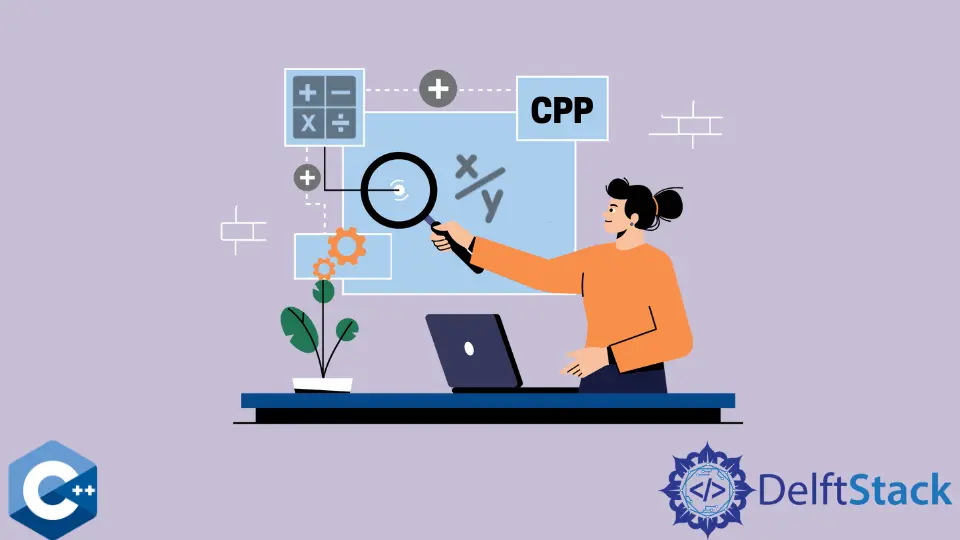
本文將討論如何使用建構函式建立分數,找到 GCD 以減少分數倍數,並對它們執行計算。
在 C++ 中使用建構函式對分數進行算術運算
讀者必須瞭解程式的先決條件。要使用建構函式建立分數,
- 分母不能為零;
- 如果分子和分母都可整除,則必須將其約簡到其最小倍數;
- 程式必須設計成通過新的資料成員儲存和顯示結果,而預先存在的變數不得改變。
這些條件將使程式可行並能夠正確計算分數。
在 C++ 中宣告建構函式並初始化其資料成員
建構函式與不同的成員方法互動並相互傳遞值。任何建構函式程式的第一步是載入匯入包並宣告預設建構函式和引數化建構函式。
-
匯入包。
#include <iostream>
-
成員方法
reduce()
將用於將分數減少到其最低倍數。int reduce(int m, int n);
-
namespace std
用於iostream
包。using namespace std;
-
類名必須與成員方法同名。
class computefraction
-
預設建構函式
private
:具有分數的分子和分母的兩個資料成員。{ private: int topline; int bottomline;
-
引數化建構函式
public:
之後宣告。預設建構函式的資料成員在此處使用兩個具有相同資料型別(int tl = 0, int bl = 1)
的新變數進行初始化。
建構函式類有五個成員方法。第一個。computefraction()
,與類名共享相同的名稱,並儲存減少前後的分數值。
在將值傳遞給其他成員方法之前,需要減少分數。為 4 種運算子計算宣告瞭四個成員方法 - 加法、減法、乘法和除法。
宣告的另外兩個成員方法讀取分數並列印它們。
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
下面是程式第一部分的表示。
#include <iostream>
int reduce(int m, int n);
using namespace std;
class computefraction {
private:
int topline;
int bottomline;
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
};
實現成員方法以在 C++ 中執行操作
本文的這一部分實現了在 C++ 中對分數執行算術運算的成員方法。
C++ 中的分數類運算子過載
在這個程式中,運算子在每個成員方法中都被過載,以將兩個分數的值放入一個物件中。當使用使用者定義的資料型別而不是內建的資料型別時會進行過載,並且需要更改其返回型別。
這是通過::
範圍解析運算子實現的。它從特定類訪問建構函式的區域性變數。
分數的值儲存在建構函式 computefraction
中。程式會將這些值傳遞給其他成員方法以對它們執行算術運算,但首先,需要減少分數才能將其提供給其他成員方法。
語法 computefraction::computefraction(int tl, int bl)
用於訪問建構函式 computefraction
的區域性變數。
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl)
類似地,在新增兩個分數的成員方法中,運算子 sum
被過載以適合兩個分數的值。
語法:
return_method class_name::operator(argument)
該語法從類 computefraction
訪問運算子 sum
,用複製物件 b2
過載它,並將最終結果返回到建構函式 computefraction
。
computefraction computefraction::sum(computefraction b2) const
C++ 中查詢分數的 GCD 的成員方法
函式 gcd
找到 GCD 以減少分數倍數。分子和分母是兩個資料成員 int m
和 int n
。
該函式首先檢查該值是負數還是正數。如果分子或分母中的任何一個為負值,則使用 m = (m < 0) ? -m : m;
來變成正值。
該函式比較變數 m
和 n
之間的較高值以找到用於減少分數倍數的 GCD。如果較大的值儲存在變數 n
中,它與 m
交換。
該函式的設計使得較高的值將始終儲存在變數 m
中。該函式從較高的值中減去較低的值,直到 m
減少到零。
最後,變數 n
中留下的值是 GCD,它被返回。
int gcd(int m, int n) {
m = (m < 0) ? -m : m;
n = (n < 0) ? -n : n;
while (m > 0) {
if (m < n) {
int bin = m;
m = n;
n = bin;
}
m -= n;
}
return n;
}
C++ 中一個分數的分子和分母減少的成員方法
通過將分子和分母除以其 GCD 來減少分數。
這裡初始化了兩個變數,分子的 topline
和分母的 bottomline
。
if-else
條件檢查變數 bottomline
值。如果值為零,則程式退出並顯示錯誤訊息。
一個整數變數 reduce
被初始化以儲存從方法 gcd
返回的兩個數字的 GCD。
然後,儲存在 reduce
中的值從 topline
和 bottomline
中分離出來,並將結果儲存回相同的變數。
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl) {
if (bl == 0) {
cout << "You cannot put 0 as a denominator" << endl;
exit(0); // program gets terminated
} else
bottomline = bl;
int reduce = gcd(topline, bottomline);
topline /= reduce;
bottomline /= reduce;
}
建立成員方法以在 C++ 中新增兩個分數
用於計算操作的所有方法都建立了一個複製物件,如複製建構函式。這是通過語法 computefraction computefraction::sum(bounty b2) const
完成的。
關鍵字 bounty b2
建立了一個複製物件 b2
。製作複製物件是在不初始化額外變數的情況下重用第二部分的物件。
新增兩個分數 a/b 和 c/d 的方程式是,
變數 int tl
儲存分子的結果,而 int bl
儲存分母的結果。最後,通過了 tl
和 bl
。
computefraction computefraction::sum(bounty b2) const {
int tl = topline * b2.bottomline + b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
其他成員方法以相同的方式建立。要檢查,讀者可以到本文的最終程式。
如何在 C++ 中使用建構函式實現主函式
一旦建立了所有成員方法,就需要編寫 main()
函式,以便程式可以返回傳遞的值並顯示它們。
該程式的編寫方式必須使終端使用者按以下順序獲得選擇和響應:
- 選擇運算子的選項,即加法、減法等。
- 如果選擇無序並且程式重新載入,則丟擲異常。
- 當給出正確的選擇時,要求使用者輸入第一部分的分子和分母,然後是第二部分。
- 如果在分母中插入零並退出,則丟擲錯誤異常。
- 如果輸入正確,程式將結果作為最終響應。
- 程式重新載入到步驟 1。
Main 函式的區域性變數和建構函式物件的初始化
作為一個選單驅動程式,宣告瞭一個字元變數 ch
。宣告瞭三個物件:up
傳遞和儲存第一個分數,down
儲存第二個分數,final
顯示兩個分數的結果。
int main() {
char ch;
computefraction up;
computefraction down;
computefraction final;
}
使用 Do While
迴圈實現選單驅動條件
該程式使用 5 個選單驅動案例和一個 do-while
迴圈下的預設案例。前四種情況執行計算操作,而第五種情況保留用於退出選項。
default
將被定義為超出範圍的選擇。程式迴圈執行,直到使用者選擇第 5 種情況跳出並結束程式。
do {
switch (ch) {
case '1':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
... case '5':
break;
default : cerr << "Choice is out of scope" << ch << endl;
break;
}
} while (ch != '5');
使用 C++ 中的建構函式計算分數的完整程式碼
// import package
#include <iostream>
// member method to find gcd, with two data members
int gcd(int m, int n);
using namespace std;
class computefraction {
// Default constructor
private:
int topline;
int bottomline;
// Parameterized Constructor
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
};
// Member methods of class type bounty.
// In constructors, the class and constructor names must be the same.
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl) {
if (bl == 0) {
cout << "You cannot put 0 as a denominator" << endl;
exit(0); // program gets terminated
} else
bottomline = bl;
// Below codes reduce the fractions using gcd
int reduce = gcd(topline, bottomline);
topline /= multiple;
bottomline /= multiple;
}
// Constructor to add fractions
computefraction computefraction::sum(computefraction b2) const {
int tl = topline * b2.bottomline + b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to subtract fractions
computefraction computefraction::minus(computefraction b2) const {
int tl = topline * b2.bottomline - b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to multiply fractions
computefraction computefraction::mult(computefraction b2) const {
int tl = topline * b2.topline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to divide fractions
computefraction computefraction::rem(computefraction b2) const {
int tl = topline * b2.bottomline;
int bl = bottomline * b2.topline;
return computefraction(tl, bl);
}
// Method to print output
void computefraction::show() const {
cout << endl << topline << "/" << bottomline << endl;
}
// Method to read input
void computefraction::see() {
cout << "Type the Numerator ";
cin >> topline;
cout << "Type the denominator ";
cin >> bottomline;
}
// GCD is calculated in this method
int gcd(int m, int n) {
m = (m < 0) ? -m : m;
n = (n < 0) ? -n : n;
while (m > 0) {
if (m < n) {
int bin = m;
m = n;
n = bin;
}
m -= n;
}
return n;
}
// Main Function
int main() {
char ch;
computefraction up;
computefraction down;
computefraction final;
do {
cout << " Choice 1\t Sum\n";
cout << " Choice 2\t Minus\n";
cout << " Choice 3\t multiply\n";
cout << " Choice 4\t Divide\n";
cout << " Choice 5\t Close\n";
cout << " \nEnter your choice: ";
cin >> ch;
cin.ignore();
switch (ch) {
case '1':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
case '2':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.minus(down);
final.show();
break;
case '3':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
case '4':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.mult(down);
final.show();
break;
case '5':
break; // exits program.
default:
cerr << "Choice is out of scope" << ch << endl;
break;
}
} while (ch != '5'); // program stops reloading when choice 5 is selected
return 0;
}
輸出:
Choice 1 Sum
Choice 2 Minus
Choice 3 multiply
Choice 4 Divide
Choice 5 Close
Enter your choice: 2
first fraction: Type the Numerator 15
Type the denominator 3
Second fraction: Type the Numerator 14
Type the denominator 7
3/1
Choice 1 Sum
Choice 2 Minus
Choice 3 multiply
Choice 4 Divide
Choice 5 Close
Enter your choice: 3
first fraction: Type the Numerator 5
Type the denominator 0
Second fraction: Type the Numerator 6
Type the denominator 8
You cannot put 0 as a denominator
--------------------------------
Process exited after 47.24 seconds with return value 0
Press any key to continue . . .