C++ でコンストラクタを使用した分数の算術演算
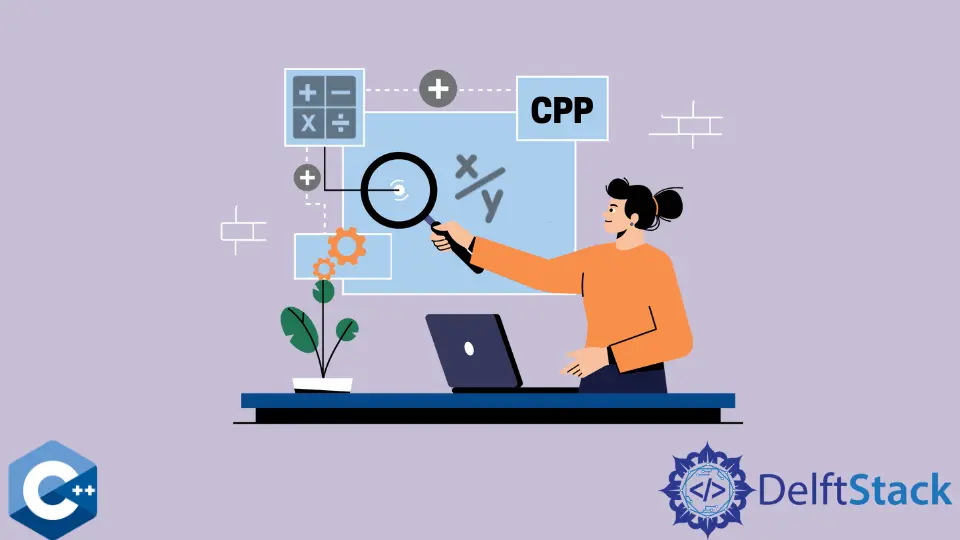
この記事では、コンストラクターを使用して分数を作成する方法、分数の倍数を減らすための GCD を見つけて、それらに対して計算を実行する方法について説明します。
C++ でコンストラクタを使用した分数の算術演算
読者は、プログラムの前提条件を理解する必要があります。コンストラクターを使用して分数を作成するには、
- 分母はゼロであってはなりません。
- 分子と分母の両方が互いに割り切れる場合は、最小の倍数に減らす必要があります。
- プログラムは、結果が新しいデータメンバーを介して保存および表示されるように設計する必要がありますが、既存の変数は変更しないでください。
これらの条件により、プログラムは実行可能になり、分数を正しく計算できるようになります。
コンストラクターを宣言し、C++ でそのデータメンバーを初期化する
コンストラクターはさまざまなメンバーメソッドと対話し、相互に値を渡します。コンストラクタープログラムの最初のステップは、インポートパッケージをロードし、デフォルトのコンストラクターとパラメーター化されたコンストラクターを宣言することです。
-
パッケージをインポートします。
#include <iostream>
-
メンバーメソッド
reduce()
を使用して、分数を最小の倍数に減らします。int reduce(int m, int n);
-
namespace std
はiostream
パッケージに使用されます。using namespace std;
-
クラス名は、メンバーメソッドと同じ名前である必要があります。
class computefraction
-
デフォルトのコンストラクター
private
:分数の分子と分母の 2つのデータメンバーがあります。{ private: int topline; int bottomline;
-
その後、パラメーター化されたコンストラクター
public:
が宣言されます。デフォルトのコンストラクターのデータメンバーは、同じデータ型(int tl = 0, int bl = 1)
の 2つの新しい変数で初期化されます。
コンストラクタークラスには 5つのメンバーからなるメソッドがあります。最初の 1つ。computefraction()
は、クラス名と同じ名前を共有し、削減前と削減後の両方の分数の値を格納します。
値が他のメンバーメソッドに渡される前に、分数の削減を行う必要があります。4つの演算子の計算(加算、減算、乗算、除算)に対して 4つのメンバーメソッドが宣言されています。
宣言された他の 2つのメンバーメソッドは、小数部を読み取り、それらを出力します。
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
以下は、プログラムの最初の部分の表現です。
#include <iostream>
int reduce(int m, int n);
using namespace std;
class computefraction {
private:
int topline;
int bottomline;
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
};
C++ で操作を実行するためのメンバーメソッドを実装する
この記事のこの部分では、C++ で分数の算術演算を実行するメンバーメソッドが実装されています。
C++ での分数クラス演算子のオーバーロード
このプログラムでは、演算子はすべてのメンバーメソッドでオーバーロードされ、2つの小数部の値を 1つのオブジェクトに適合させます。オーバーロードは、組み込みデータ型の代わりにユーザー定義データ型が使用され、その戻り型を変更する必要がある場合に実行されます。
これは、::
スコープ解決演算子によって実現されます。特定のクラスのコンストラクターのローカル変数にアクセスします。
小数部の値は、コンストラクターcomputefraction
に格納されます。プログラムはこれらの値を他のメンバーメソッドに渡して算術演算を実行しますが、最初に、他のメンバーメソッドに渡す前に小数部を減らす必要があります。
構文 computefraction::computefraction(int tl, int bl)
は、コンストラクターcomputefraction
のローカル変数にアクセスするために使用されます。
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl)
同様に、2つの小数部を追加するメンバーメソッドでは、演算子 sum
が 2つの小数部の値に合うようにオーバーロードされます。
構文:
return_method class_name::operator(argument)
この構文は、クラス computefraction
から演算子 sum
にアクセスし、コピーオブジェクト b2
でオーバーロードし、最終結果をコンストラクタ computefraction
に返します。
computefraction computefraction::sum(computefraction b2) const
C++ で分数の GCD を求めるメンバメソッド
関数 gcd
は、分数の倍数を減らすために GCD を求めます。分子と分母は、int m
と int n
の 2つのデータメンバーです。
この関数は、最初に値が負か正かをチェックします。分子または分母のいずれかが負の場合は、m = (m < 0) ? -m : m;
を使用して正にします。
この関数は、変数 m
と n
の高い値を比較して、小数倍数を減らすための GCD を見つけます。大きい方の値が変数 n
に格納されている場合、それは m
と交換されます。
この関数は、高い値が常に変数 m
に格納されるように設計されています。この関数は、m
がゼロになるまで、高い値から低い値を減算します。
最後に、変数 n
に残っている値が GCD であり、それが返されます。
int gcd(int m, int n) {
m = (m < 0) ? -m : m;
n = (n < 0) ? -n : n;
while (m > 0) {
if (m < n) {
int bin = m;
m = n;
n = bin;
}
m -= n;
}
return n;
}
C++ で分数の分子と分母を減らすためのメンバーメソッド
分母は、分子と分母をその GCD で割ることによって削減されます。
ここでは、分子の topline
と分母の bottomline
の 2つの変数が初期化されます。
if-else
条件は、変数 bottomline
値をチェックします。値がゼロの場合、プログラムはエラーメッセージを表示して終了します。
整数変数 reduce
は、メソッド gcd
から返された 2つの数値の GCD を格納するために初期化されます。
次に、reduce
に格納されている値が topline
と bottomline
の両方から除算され、結果が同じ変数に格納されます。
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl) {
if (bl == 0) {
cout << "You cannot put 0 as a denominator" << endl;
exit(0); // program gets terminated
} else
bottomline = bl;
int reduce = gcd(topline, bottomline);
topline /= reduce;
bottomline /= reduce;
}
C++ で 2つの分数を追加するためのメンバーメソッドを作成する
計算操作に使用されるすべてのメソッドは、コピーコンストラクターのようなコピーオブジェクトを作成します。これは、構文 computefraction computefraction::sum(bounty b2) const
によって行われます。
キーワード bounty b2
は、コピーオブジェクト b2
を作成します。コピーオブジェクトを作成することは、余分な変数を初期化することなく、2 番目の部分のオブジェクトを再利用することです。
2つの分数 a/b と c/d を加算する式は次のとおりです。
変数 int tl
は分子の結果を格納し、int bl
は分母の結果を格納します。最後に、tl
と bl
が渡されます。
computefraction computefraction::sum(bounty b2) const {
int tl = topline * b2.bottomline + b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
他のメンバーメソッドも同じ方法で作成されます。確認するには、読者はこの記事の最後のプログラムに進むことができます。
C++ でコンストラクターを使用してメイン関数を実装する方法
すべてのメンバーメソッドが作成されたら、プログラムが渡された値を返し、それらを表示できるように、main()
関数を作成する必要があります。
このプログラムは、エンドユーザーに次の順序で選択肢と応答が与えられるように作成する必要があります。
- 演算子を選択するオプション、つまり、加算、減算など。
- 選択が正しくなく、プログラムがリロードされた場合は、例外をスローします。
- 正しい選択が与えられると、ユーザーは最初の分数の分子と分母を入力し、続いて 2 番目の分数を入力するように求められます。
- 分母にゼロが挿入されて終了すると、エラー例外がスローされます。
- 入力が正しければ、プログラムは結果を最終応答として返します。
- プログラムはステップ 1 にリロードします。
メイン関数のローカル変数とコンストラクターオブジェクトの初期化
メニュー方式のプログラムとして、char 変数 ch
が宣言されています。3つのオブジェクトが宣言されています。最初の分数を渡して保存する up
、2 番目の分数を保持する down
、2つの分数の結果を表示する final
です。
int main() {
char ch;
computefraction up;
computefraction down;
computefraction final;
}
do while
ループを使用してメニュー駆動型条件を実装する
プログラムは、do-while
ループの下で 5つのメニュー方式のケースとデフォルトのケースを使用します。最初の 4つのケースは計算操作を実行し、5 番目のケースは終了オプション用に予約されています。
デフォルト
は、範囲外の選択に対して定義されます。プログラムは、ユーザーが 5 番目のケースを選択してプログラムを終了し、プログラムを終了するまで、ループで実行されます。
do {
switch (ch) {
case '1':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
... case '5':
break;
default : cerr << "Choice is out of scope" << ch << endl;
break;
}
} while (ch != '5');
C++ でコンストラクターを使用して分数を計算するための完全なコード
// import package
#include <iostream>
// member method to find gcd, with two data members
int gcd(int m, int n);
using namespace std;
class computefraction {
// Default constructor
private:
int topline;
int bottomline;
// Parameterized Constructor
public:
computefraction(int tl = 0, int bl = 1);
computefraction sum(computefraction b2) const;
computefraction minus(computefraction b2) const;
computefraction mult(computefraction b2) const;
computefraction rem(computefraction b2) const;
void show() const;
void see();
};
// Member methods of class type bounty.
// In constructors, the class and constructor names must be the same.
computefraction::computefraction(int tl, int bl) : topline(tl), bottomline(bl) {
if (bl == 0) {
cout << "You cannot put 0 as a denominator" << endl;
exit(0); // program gets terminated
} else
bottomline = bl;
// Below codes reduce the fractions using gcd
int reduce = gcd(topline, bottomline);
topline /= multiple;
bottomline /= multiple;
}
// Constructor to add fractions
computefraction computefraction::sum(computefraction b2) const {
int tl = topline * b2.bottomline + b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to subtract fractions
computefraction computefraction::minus(computefraction b2) const {
int tl = topline * b2.bottomline - b2.topline * bottomline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to multiply fractions
computefraction computefraction::mult(computefraction b2) const {
int tl = topline * b2.topline;
int bl = bottomline * b2.bottomline;
return computefraction(tl, bl);
}
// Constructor to divide fractions
computefraction computefraction::rem(computefraction b2) const {
int tl = topline * b2.bottomline;
int bl = bottomline * b2.topline;
return computefraction(tl, bl);
}
// Method to print output
void computefraction::show() const {
cout << endl << topline << "/" << bottomline << endl;
}
// Method to read input
void computefraction::see() {
cout << "Type the Numerator ";
cin >> topline;
cout << "Type the denominator ";
cin >> bottomline;
}
// GCD is calculated in this method
int gcd(int m, int n) {
m = (m < 0) ? -m : m;
n = (n < 0) ? -n : n;
while (m > 0) {
if (m < n) {
int bin = m;
m = n;
n = bin;
}
m -= n;
}
return n;
}
// Main Function
int main() {
char ch;
computefraction up;
computefraction down;
computefraction final;
do {
cout << " Choice 1\t Sum\n";
cout << " Choice 2\t Minus\n";
cout << " Choice 3\t multiply\n";
cout << " Choice 4\t Divide\n";
cout << " Choice 5\t Close\n";
cout << " \nEnter your choice: ";
cin >> ch;
cin.ignore();
switch (ch) {
case '1':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
case '2':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.minus(down);
final.show();
break;
case '3':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.sum(down);
final.show();
break;
case '4':
cout << "first fraction: ";
up.see();
cout << "Second fraction: ";
down.see();
final = up.mult(down);
final.show();
break;
case '5':
break; // exits program.
default:
cerr << "Choice is out of scope" << ch << endl;
break;
}
} while (ch != '5'); // program stops reloading when choice 5 is selected
return 0;
}
出力:
Choice 1 Sum
Choice 2 Minus
Choice 3 multiply
Choice 4 Divide
Choice 5 Close
Enter your choice: 2
first fraction: Type the Numerator 15
Type the denominator 3
Second fraction: Type the Numerator 14
Type the denominator 7
3/1
Choice 1 Sum
Choice 2 Minus
Choice 3 multiply
Choice 4 Divide
Choice 5 Close
Enter your choice: 3
first fraction: Type the Numerator 5
Type the denominator 0
Second fraction: Type the Numerator 6
Type the denominator 8
You cannot put 0 as a denominator
--------------------------------
Process exited after 47.24 seconds with return value 0
Press any key to continue . . .