Default Constructor and Default Keyword in C++
- What is a Default Constructor?
- The Role of the Default Keyword
- Benefits of Using Default Constructor and Default Keyword
- Practical Applications of Default Constructors
- Conclusion
- FAQ
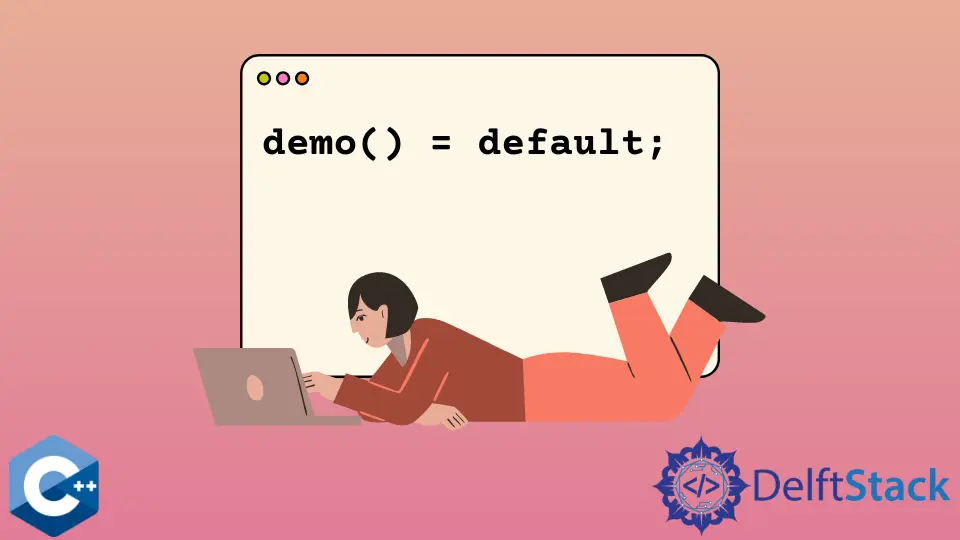
Understanding C++ can be a challenging yet rewarding journey for programmers. Among the many concepts in this powerful language, the default constructor and the default keyword are fundamental building blocks that every C++ developer should grasp.
In this article, we will delve into what a default constructor is, how it operates, and the role of the default keyword in simplifying object creation. Whether you’re a novice or an experienced programmer, this guide will provide valuable insights into these concepts, helping you write cleaner and more efficient code. Let’s explore the world of C++ constructors and keywords together!
What is a Default Constructor?
A default constructor in C++ is a special type of constructor that initializes an object without requiring any parameters. If no constructors are defined in a class, the compiler automatically provides a default constructor. This constructor can be either implicit or explicit, depending on how it is defined.
Let’s look at a simple example to illustrate this concept:
#include <iostream>
class MyClass {
public:
MyClass() {
std::cout << "Default constructor called!" << std::endl;
}
};
int main() {
MyClass obj;
return 0;
}
When you create an instance of MyClass
, the default constructor is invoked automatically. In this case, the message “Default constructor called!” will be printed to the console.
Output:
Default constructor called!
In this example, MyClass
has a default constructor that outputs a message when an object is created. This shows how default constructors can be used to initialize class members and perform setup tasks.
The Role of the Default Keyword
The default keyword in C++ is used to indicate that the compiler should generate a default implementation of a constructor, assignment operator, or destructor. This is particularly useful when you want to explicitly declare a default constructor in a class that already has other constructors defined.
Here’s a practical example:
#include <iostream>
class MyClass {
public:
MyClass(int x) {
std::cout << "Parameterized constructor called with value: " << x << std::endl;
}
MyClass() = default; // Explicitly declare default constructor
};
int main() {
MyClass obj1(10); // Calls parameterized constructor
MyClass obj2; // Calls default constructor
return 0;
}
In this code, we have both a parameterized constructor and a default constructor. The default constructor is explicitly declared using the default
keyword.
Output:
Parameterized constructor called with value: 10
Default constructor called!
By using the default keyword, you can ensure that your class has a default constructor even when other constructors are present. This gives you more control over object initialization and enhances code clarity.
Benefits of Using Default Constructor and Default Keyword
The default constructor and the default keyword in C++ provide several benefits. First and foremost, they simplify object creation. When you have a default constructor, you can create objects without needing to pass any parameters, making your code cleaner and easier to read.
Additionally, using the default keyword allows you to maintain default behavior in classes that have multiple constructors. This can be particularly useful in complex applications where you need to manage various object states without cluttering your code with multiple constructor definitions.
Moreover, default constructors can help prevent errors. By ensuring that objects are automatically initialized, you reduce the risk of uninitialized variables, which can lead to unpredictable behavior in your programs.
Practical Applications of Default Constructors
Default constructors are widely used in various applications, especially when dealing with collections of objects, such as arrays or vectors. When you create a collection of objects, having a default constructor allows you to easily instantiate all the objects in the collection without needing to provide specific values.
Consider the following example where we create an array of objects:
#include <iostream>
#include <array>
class MyClass {
public:
MyClass() {
std::cout << "Default constructor called!" << std::endl;
}
};
int main() {
std::array<MyClass, 3> myArray; // Creates an array of 3 MyClass objects
return 0;
}
In this case, the array myArray
will have three instances of MyClass
, each initialized by the default constructor.
Output:
Default constructor called!
Default constructor called!
Default constructor called!
This example illustrates how default constructors facilitate the creation of multiple objects seamlessly. By leveraging default constructors, developers can focus on building robust applications without worrying about the intricacies of object initialization.
Conclusion
In summary, the default constructor and the default keyword are essential concepts in C++. They simplify object creation, enhance code clarity, and help prevent errors associated with uninitialized variables. By mastering these concepts, you can write cleaner and more efficient C++ code. Whether you are developing small applications or large systems, understanding how to use default constructors and the default keyword will empower you to create better software solutions.
FAQ
-
What is a default constructor in C++?
A default constructor is a constructor that can be called with no arguments. It initializes an object of a class without requiring any parameters. -
How do I declare a default constructor?
You can declare a default constructor by defining a constructor without parameters or by using the default keyword in C++. -
What is the purpose of the default keyword?
The default keyword instructs the compiler to generate a default implementation of a constructor, assignment operator, or destructor when needed. -
Can a class have multiple constructors?
Yes, a class can have multiple constructors, including default, parameterized, and copy constructors, allowing for flexible object initialization. -
Why is it important to use default constructors?
Default constructors simplify object creation, help prevent uninitialized variables, and enhance code readability, making them vital for robust C++ programming.