Struct Constructors in C++
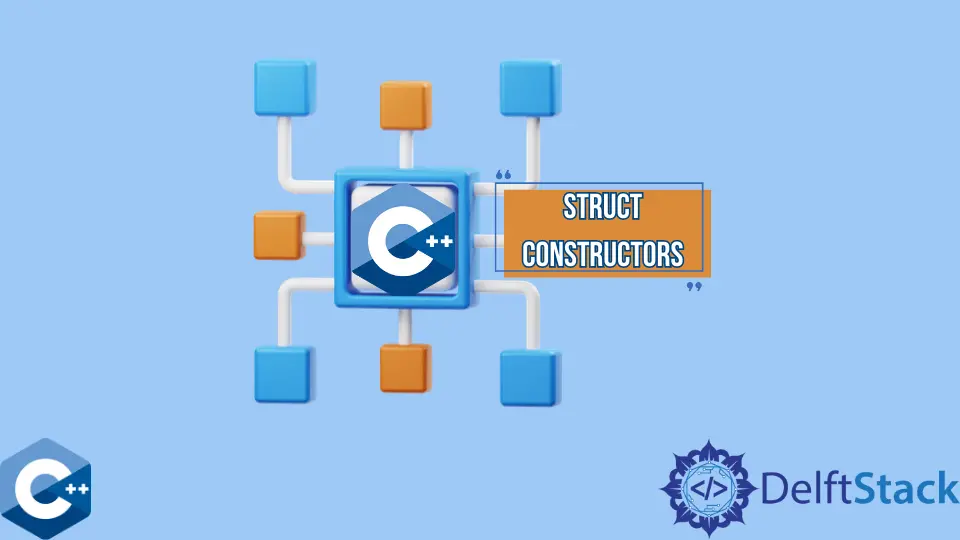
When it comes to C++, understanding how to use constructors within structures is fundamental for effective programming. Constructors are special member functions that are automatically invoked when an object of a structure is created. They allow you to initialize your structure’s data members, ensuring that your objects start life in a valid state. In this short tutorial, we’ll explore how to implement constructors in C++ structures, providing you with clear examples and explanations. Whether you’re a beginner or looking to refresh your knowledge, this guide will help you grasp the concept in no time.
What is a Constructor?
A constructor in C++ is a member function that initializes objects of a class or structure. Unlike regular functions, constructors have the same name as the structure or class and do not have a return type. They can be overloaded, allowing multiple constructors to exist with different parameter lists. This feature provides flexibility in object creation.
Basic Constructor Example
Let’s start with a simple example of a structure with a constructor. Here, we define a structure called Person
, which has two data members: name
and age
. We also define a constructor to initialize these members.
#include <iostream>
#include <string>
struct Person {
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
};
int main() {
Person person1("Alice", 30);
std::cout << "Name: " << person1.name << ", Age: " << person1.age << std::endl;
return 0;
}
Output:
Name: Alice, Age: 30
In this example, we declare a constructor that takes two parameters: a string for the name and an integer for the age. The constructor uses an initializer list to set the values of name
and age
. When we create an instance of Person
, we pass the values directly, initializing the object in one go. This ensures that every Person
object is created with valid data.
Default Constructor
A default constructor is a constructor that can be called without any arguments. It’s useful for creating objects with default values. If you don’t define any constructors, C++ automatically generates a default constructor for you. However, if you define any constructor, you’ll need to explicitly define a default constructor if you want one.
Default Constructor Example
Let’s enhance our Person
structure with a default constructor.
#include <iostream>
#include <string>
struct Person {
std::string name;
int age;
Person() : name("Unknown"), age(0) {}
Person(std::string n, int a) : name(n), age(a) {}
};
int main() {
Person person1;
Person person2("Bob", 25);
std::cout << "Person 1: " << person1.name << ", Age: " << person1.age << std::endl;
std::cout << "Person 2: " << person2.name << ", Age: " << person2.age << std::endl;
return 0;
}
Output:
Person 1: Unknown, Age: 0
Person 2: Bob, Age: 25
In this modified version, we introduced a default constructor that initializes name
to “Unknown” and age
to 0. When we create person1
without arguments, it calls the default constructor, while person2
uses the parameterized constructor. This flexibility allows us to create objects with specific or default values as needed.
Copy Constructor
A copy constructor is a special type of constructor that initializes an object using another object of the same type. It’s particularly useful when you want to create a new object as a copy of an existing object. C++ provides a default copy constructor if you don’t define one, but it performs a shallow copy, which may not always be desirable.
Copy Constructor Example
Let’s see how to implement a copy constructor in our Person
structure.
#include <iostream>
#include <string>
struct Person {
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
Person(const Person &p) : name(p.name), age(p.age) {}
};
int main() {
Person person1("Charlie", 28);
Person person2 = person1; // Invokes the copy constructor
std::cout << "Person 1: " << person1.name << ", Age: " << person1.age << std::endl;
std::cout << "Person 2: " << person2.name << ", Age: " << person2.age << std::endl;
return 0;
}
Output:
Person 1: Charlie, Age: 28
Person 2: Charlie, Age: 28
In this example, we defined a copy constructor that takes a reference to another Person
object. When we create person2
as a copy of person1
, the copy constructor is invoked, and person2
receives the same values as person1
. This is particularly useful when managing resources or when the structure contains dynamically allocated memory.
Destructor
While constructors initialize objects, destructors are used to clean up when an object goes out of scope. A destructor has the same name as the structure or class but is prefixed with a tilde (~). It’s called automatically when an object is destroyed.
Destructor Example
Let’s add a destructor to our Person
structure to illustrate its use.
#include <iostream>
#include <string>
struct Person {
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
~Person() {
std::cout << "Destructor called for " << name << std::endl;
}
};
int main() {
Person person1("Diana", 35);
return 0;
}
Output:
Destructor called for Diana
In this example, we defined a destructor that outputs a message when an object is destroyed. When person1
goes out of scope at the end of the main
function, the destructor is called automatically, demonstrating how destructors can be used for cleanup tasks, such as releasing resources.
Conclusion
Constructors are a powerful feature in C++ that allow you to initialize objects of structures effectively. By understanding the different types of constructors—default, copy, and destructors—you can ensure that your objects are always in a valid state and manage resources properly. Whether you’re creating simple data structures or complex classes, mastering constructors will enhance your programming skills and lead to more robust, maintainable code.
FAQ
-
What are constructors in C++?
Constructors are special member functions that initialize objects of a class or structure. -
Can constructors be overloaded?
Yes, constructors can be overloaded, allowing multiple constructors with different parameter lists.
-
What is a default constructor?
A default constructor is one that can be called without any arguments, initializing members with default values. -
What is a copy constructor?
A copy constructor initializes a new object using another object of the same type, allowing for deep or shallow copies. -
Why do we need destructors?
Destructors are used to clean up resources when an object goes out of scope, ensuring proper resource management.