How to Copy Constructor for Singly Linked List in C++
- Importance of Copy Constructors for Singly Linked List
- Iterative Copying in Singly Linked List Copy Constructors
- Recursive Copying in Singly Linked List Copy Constructors
- Copy-And-Swap Idiom in Singly Linked List Copy Constructors
- Standard Containers in Singly Linked List Copy Constructors
- Conclusion
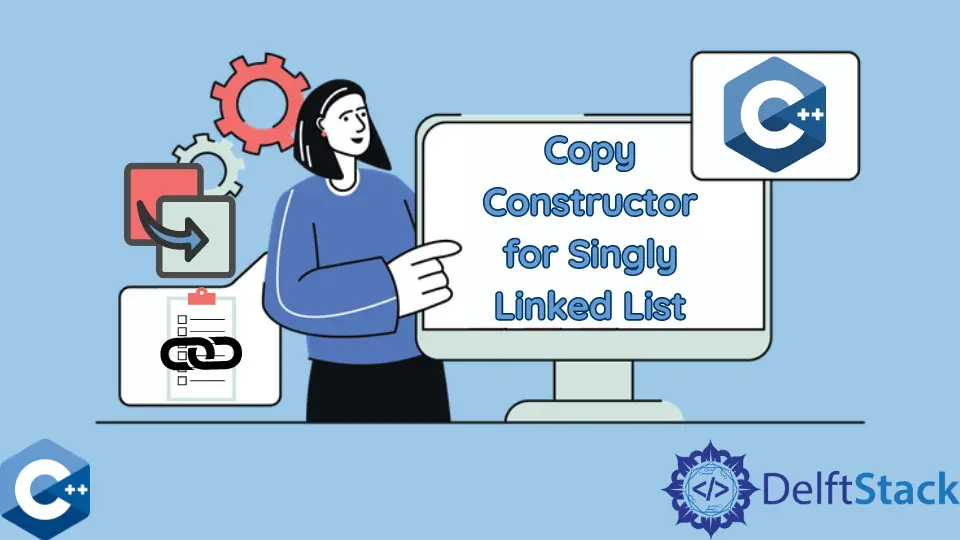
This article explores the significance of copy constructors in singly linked lists and various methods for their implementation.
Importance of Copy Constructors for Singly Linked List
Copy constructors for singly linked lists are essential for creating independent copies of the list, enabling data replication without sharing memory references. This ensures that modifications to one instance of the list do not affect others, promoting data integrity and preventing unintended side effects.
Copy constructors are particularly crucial in scenarios where distinct instances of the linked list are needed, such as in function parameter passing, class object initialization, or any situation requiring separate, identical copies. They contribute to code reliability by maintaining data encapsulation and avoiding unintended data modifications, enhancing the overall robustness of programs that involve singly linked lists.
Iterative Copying in Singly Linked List Copy Constructors
Iterative copying in singly linked list copy constructors is crucial for simplicity and efficiency. Unlike recursive approaches, iterative methods avoid the risk of stack overflow, making them more suitable for large lists.
Additionally, iterative copying allows for better control over memory resources and avoids potential issues with deep recursion. It is a straightforward and resource-efficient technique, making it an essential choice for developers seeking a pragmatic and scalable solution in situations where the size of the linked list might be a concern.
Code Example:
#include <iostream>
template <typename T>
class Node {
public:
T data;
Node* next;
Node(T value) : data(value), next(nullptr) {}
};
template <typename T>
class SinglyLinkedList {
private:
Node<T>* head;
public:
SinglyLinkedList() : head(nullptr) {}
SinglyLinkedList(const SinglyLinkedList& other) {
head = nullptr;
Node<T>* current = other.head;
Node<T>* tail = nullptr;
while (current != nullptr) {
Node<T>* newNode = new Node<T>(current->data);
if (head == nullptr) {
head = newNode;
} else {
tail->next = newNode;
}
tail = newNode;
current = current->next;
}
}
void display() {
Node<T>* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
void push(T value) {
Node<T>* newNode = new Node<T>(value);
newNode->next = head;
head = newNode;
}
~SinglyLinkedList() {
Node<T>* current = head;
Node<T>* nextNode;
while (current != nullptr) {
nextNode = current->next;
delete current;
current = nextNode;
}
}
};
int main() {
SinglyLinkedList<int> originalList;
originalList.push(3);
originalList.push(2);
originalList.push(1);
std::cout << "Original List: ";
originalList.display();
SinglyLinkedList<int> copiedList(originalList);
std::cout << "Copied List: ";
copiedList.display();
return 0;
}
The SinglyLinkedList
class manages the linked list, featuring a default constructor, a copy constructor, a display
method for printing elements, a push
method to add elements to the front, and a destructor for memory cleanup. The copy constructor initializes the new list (copiedList
) with a null head and iterates through the original list (other
) using a while
loop.
For each node in the original list, a new node with the same data is created and linked to the new list, maintaining the end with the tail
pointer. The display
method prints list elements, the push
method adds to the front, and the destructor ensures proper memory cleanup by deleting each node.
Output:
The iterative copying method for copy constructors in singly linked lists provides a straightforward and efficient approach to duplicate the structure and content of a list. Understanding the intricacies of this method not only enhances one’s grasp of C++ programming but also lays a solid foundation for working with more complex data structures and memory management tasks.
Recursive Copying in Singly Linked List Copy Constructors
Recursive copying in singly linked list copy constructors is important for its simplicity and elegance. It provides a clear and intuitive way to handle the copying process by breaking it down into smaller, self-contained steps.
Recursive methods are often favored for their readability, making the code more straightforward and easier to understand. While recursive approaches may introduce a slight overhead due to function calls, the trade-off in terms of code clarity and conciseness can be valuable, especially when dealing with smaller to moderately sized linked lists.
Code Example:
#include <iostream>
template <typename T>
class Node {
public:
T data;
Node* next;
Node(T value) : data(value), next(nullptr) {}
};
template <typename T>
class SinglyLinkedList {
private:
Node<T>* head;
public:
SinglyLinkedList() : head(nullptr) {}
SinglyLinkedList(const SinglyLinkedList& other) {
head = copyNodes(other.head);
}
Node<T>* copyNodes(Node<T>* original) {
if (original == nullptr) {
return nullptr;
}
Node<T>* newNode = new Node<T>(original->data);
newNode->next = copyNodes(original->next);
return newNode;
}
void display() {
Node<T>* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
void push(T value) {
Node<T>* newNode = new Node<T>(value);
newNode->next = head;
head = newNode;
}
~SinglyLinkedList() {
Node<T>* current = head;
Node<T>* nextNode;
while (current != nullptr) {
nextNode = current->next;
delete current;
current = nextNode;
}
}
};
int main() {
SinglyLinkedList<int> originalList;
originalList.push(3);
originalList.push(2);
originalList.push(1);
std::cout << "Original List: ";
originalList.display();
SinglyLinkedList<int> copiedList(originalList);
std::cout << "Copied List: ";
copiedList.display();
return 0;
}
Defining a Node
class encapsulating linked list elements, each node holding data and a pointer to the next node. The SinglyLinkedList
class manages the list, offering methods for copy construction, display, and memory cleanup.
The recursive copy constructor initializes the new list (copiedList
) by employing the copyNodes
helper function. In the recursive process, copyNodes
takes a pointer to the original list, returning nullptr
if empty, and creates a new node for each original node, calling the function recursively for the subsequent node.
The display
method prints list elements, the push
method adds to the front, and the destructor ensures proper memory cleanup by deleting each node.
Output:
The recursive copying method for copy constructors in singly linked lists adds a touch of elegance to the process of duplicating a list. By harnessing the power of recursion, this technique provides a concise and expressive way to replicate the structure and content of a linked list.
Copy-And-Swap Idiom in Singly Linked List Copy Constructors
The Copy and Swap Idiom in singly linked list copy constructors is vital for its simplicity and exception safety. This technique ensures efficient memory management by leveraging the standard assignment operator and a temporary object.
It eliminates the need for manual resource management, making the code concise and readable. Moreover, the idiom guarantees transactional behavior, safeguarding against potential errors during the copy process.
This approach simplifies code maintenance and adheres to best practices, providing a robust and straightforward solution for creating copies of singly linked lists.
Code Example:
#include <iostream>
#include <utility>
template <typename T>
class Node {
public:
T data;
Node* next;
Node(T value) : data(value), next(nullptr) {}
};
template <typename T>
class SinglyLinkedList {
private:
Node<T>* head;
public:
SinglyLinkedList() : head(nullptr) {}
// Copy constructor using the Copy-and-Swap Idiom
SinglyLinkedList(const SinglyLinkedList& other) : head(nullptr) {
Node<T>* current = other.head;
Node<T>* tail = nullptr;
while (current != nullptr) {
Node<T>* newNode = new Node<T>(current->data);
if (head == nullptr) {
head = newNode;
} else {
tail->next = newNode;
}
tail = newNode;
current = current->next;
}
}
void display() const {
Node<T>* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
void push(T value) {
Node<T>* newNode = new Node<T>(value);
newNode->next = head;
head = newNode;
}
~SinglyLinkedList() {
Node<T>* current = head;
Node<T>* nextNode;
while (current != nullptr) {
nextNode = current->next;
delete current;
current = nextNode;
}
}
};
int main() {
SinglyLinkedList<int> originalList;
originalList.push(3);
originalList.push(2);
originalList.push(1);
std::cout << "Original List: ";
originalList.display();
SinglyLinkedList<int> copiedList(originalList);
std::cout << "Copied List: ";
copiedList.display();
return 0;
}
In the context of a singly linked list copy constructor, the Node
class defines elements, with each node containing data and a pointer to the next node. The SinglyLinkedList
class manages the list, offering methods for copy construction, display, and memory cleanup.
The copy constructor implements the Copy-and-Swap Idiom, creating a temporary copy (temp
) through a while
loop and subsequently swapping content with the original object. The display
method prints list elements, the push
method adds to the front, and the destructor ensures proper memory cleanup by deleting each node.
This application of the Copy-and-Swap Idiom enhances code clarity and adheres to best practices for creating linked list copies.
Output:
The Copy-and-Swap Idiom simplifies the implementation of copy constructors, providing a concise and robust way to duplicate objects, especially in the realm of C++ programming. This technique not only improves code readability but also ensures exception safety and efficient resource management.
Standard Containers in Singly Linked List Copy Constructors
Utilizing standard containers in singly linked list copy constructors, such as std::vector
, is crucial for its simplicity and efficiency. Standard containers offer a convenient mechanism for managing dynamic memory, eliminating manual memory allocation and deallocation.
This approach enhances code readability, reduces the risk of memory leaks, and simplifies the overall implementation. By leveraging established container functionalities, developers benefit from well-tested and optimized code, resulting in a more maintainable solution.
Standard containers provide a robust foundation, ensuring a streamlined and error-resistant process for creating copies of singly linked lists.
Code Example:
#include <iostream>
#include <vector>
template <typename T>
class Node {
public:
T data;
Node* next;
Node(T value) : data(value), next(nullptr) {}
};
template <typename T>
class SinglyLinkedList {
private:
Node<T>* head;
public:
SinglyLinkedList() : head(nullptr) {}
// Copy constructor using the Standard Containers Idiom
SinglyLinkedList(const SinglyLinkedList& other) {
head = nullptr; // Initialize the head of the new list
std::vector<T> tempVector;
Node<T>* current = other.head;
// Copy elements from the original list to the vector
while (current != nullptr) {
tempVector.push_back(current->data);
current = current->next;
}
// Rebuild the new list from the vector
for (auto it = tempVector.rbegin(); it != tempVector.rend(); ++it) {
push(*it);
}
}
void display() const {
Node<T>* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
void push(T value) {
Node<T>* newNode = new Node<T>(value);
newNode->next = head;
head = newNode;
}
~SinglyLinkedList() {
Node<T>* current = head;
Node<T>* nextNode;
while (current != nullptr) {
nextNode = current->next;
delete current;
current = nextNode;
}
}
};
int main() {
SinglyLinkedList<int> originalList;
originalList.push(3);
originalList.push(2);
originalList.push(1);
std::cout << "Original List: ";
originalList.display();
SinglyLinkedList<int> copiedList(originalList);
std::cout << "Copied List: ";
copiedList.display();
return 0;
}
The Standard Containers Idiom is intricately implemented in the provided code for a singly linked list. The Node
class establishes the fundamental building block, encapsulating data and a pointer to the next node.
Within the SinglyLinkedList
class, responsible for managing the list, the copy constructor employs the Standard Containers Idiom. The head of the new list initializes to nullptr
for a clean start.
The copy process involves a std::vector
named tempVector
temporarily storing elements from the original list.
A while
loop iterates through the original list, pushing elements into the vector. Subsequently, a for
loop reconstructs the new list in reverse order from the vector, effectively creating a copy.
The display
method prints list elements, the push
method adds to the front, and the destructor ensures proper memory cleanup by deleting each node.
Output:
The Standard Containers Idiom provides a concise and efficient method for implementing copy constructors in singly linked lists. By leveraging the capabilities of standard containers like std::vector
, this approach not only simplifies the code but also enhances its readability.
This method showcases the adaptability and elegance inherent in C++, offering a powerful toolset for developers aiming to write clean, efficient, and expressive code.
Conclusion
Understanding the importance of copy constructors for singly linked lists is fundamental in ensuring the integrity and independence of data structures. Copy constructors play a pivotal role in creating duplicate instances of a linked list, preventing unintended side effects, and maintaining data encapsulation.
Throughout this article, we explored various methods of implementing copy constructors, including iterative copying, recursive copying, the Copy-and-Swap idiom, and utilizing standard containers. Each method offers distinct advantages, providing developers with flexible options based on specific needs and preferences.
By comprehending the significance of copy constructors and employing appropriate techniques, programmers can enhance code reliability, foster data consistency, and build robust applications involving singly linked lists.