How to Empty Constructors in C++
- Understanding Empty Constructors
- Creating an Empty Constructor in C++
- When to Use Empty Constructors
- Advantages of Using Empty Constructors
- Conclusion
- FAQ
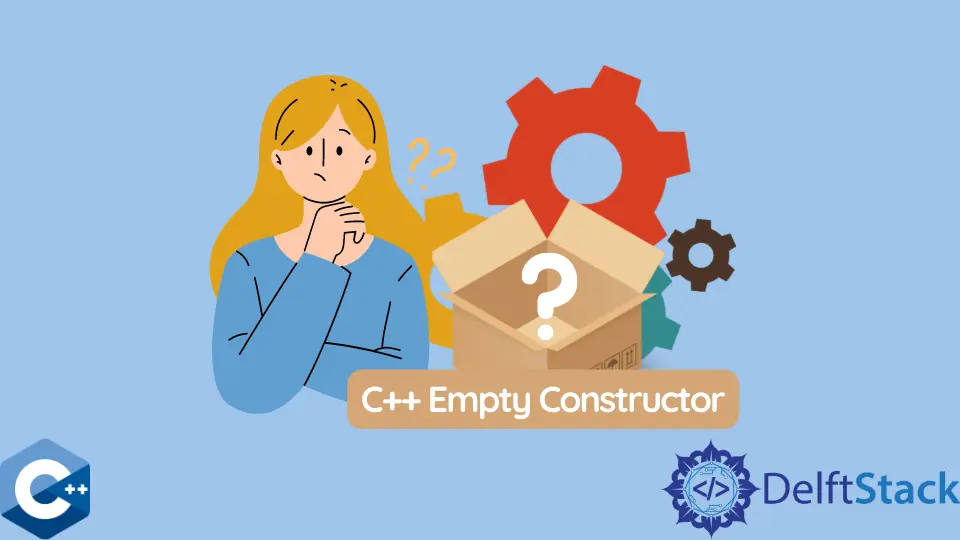
When diving into the world of C++, one concept that often surfaces is the empty constructor. This is particularly relevant when a class requires default initialization without any specific parameters. An empty constructor is a simple yet powerful tool that can streamline your code and enhance its readability. Whether you’re a seasoned developer or a beginner, understanding how to effectively use empty constructors can significantly improve your coding practices.
In this article, we will explore what empty constructors are, how to implement them in C++, and the scenarios in which they are most beneficial. Let’s get started!
Understanding Empty Constructors
An empty constructor in C++ is a constructor that does not take any parameters and does not contain any code. Its primary purpose is to provide a default way to instantiate objects of a class. This can be particularly useful when you want to create an object without needing to provide any initial values.
For example, consider a class named Car
. If you want to create a car object without specifying any attributes like color or model, an empty constructor allows you to do just that. It sets up the object in a state that you can modify later, making your code more flexible and easier to manage.
Here’s a simple example of an empty constructor:
class Car {
public:
Car() {
// Empty constructor
}
};
The constructor Car()
is defined but does not execute any code when a Car
object is instantiated. This is the essence of an empty constructor.
Creating an Empty Constructor in C++
Creating an empty constructor is straightforward in C++. You simply declare a constructor within your class definition without any parameters. This constructor can be used to initialize member variables to their default values or leave them uninitialized.
Here’s how you can define a class with an empty constructor:
class Dog {
public:
Dog() {
// Empty constructor
}
};
Output:
No output, as there are no operations performed.
In this example, the Dog
class has an empty constructor. When you create an object of the Dog
class, the constructor is called, but since it does nothing, there are no side effects. This allows you to create a Dog
object easily without worrying about initial conditions.
This technique is particularly useful when working with arrays of objects or when you need to create a collection of objects that will be initialized later. It simplifies the process of object creation, allowing you to focus on the logic of your application rather than the intricacies of initialization.
When to Use Empty Constructors
Empty constructors are particularly useful in several scenarios. One common use case is when you are working with classes that will eventually have their member variables set through other methods or functions. For instance, if you’re developing a game, you might have a Player
class where the player’s attributes are set after the object is created.
Here’s an example of how an empty constructor can be beneficial:
class Player {
private:
std::string name;
int score;
public:
Player() {
// Empty constructor
}
void setName(std::string playerName) {
name = playerName;
}
void setScore(int playerScore) {
score = playerScore;
}
};
In this Player
class, the empty constructor allows you to create a Player
object without needing to set the name or score immediately. You can call setName
and setScore
later, providing flexibility in how you manage the object’s state.
Another scenario where empty constructors shine is during the creation of dynamic arrays or collections of objects. When you need to create multiple instances of a class, having an empty constructor allows you to instantiate these objects easily, and you can populate them later as needed.
Advantages of Using Empty Constructors
Using empty constructors offers several advantages that can enhance your coding experience in C++. Here are a few key benefits:
- Simplicity: Empty constructors simplify the instantiation of objects, making your code cleaner and easier to read.
- Flexibility: They provide flexibility in how and when you initialize member variables, allowing for a more dynamic approach to object management.
- Ease of Use: You can create objects without needing to worry about passing parameters, which is particularly useful in complex applications.
- Integration with Other Code: Empty constructors work well with other classes and functions, making them a versatile choice in object-oriented programming.
Let’s illustrate these advantages with a simple example:
class Book {
private:
std::string title;
std::string author;
public:
Book() {
// Empty constructor
}
void setDetails(std::string bookTitle, std::string bookAuthor) {
title = bookTitle;
author = bookAuthor;
}
};
In this Book
class, the empty constructor allows you to create Book
objects without having to specify the title and author right away. This can be incredibly useful in scenarios where you may not have all the information at the time of object creation.
Conclusion
In conclusion, empty constructors in C++ provide a valuable tool for developers looking to simplify their code and enhance flexibility. By allowing for default initialization without parameters, empty constructors enable easier object management and cleaner code structures. Whether you’re creating a single object or a collection, understanding how to implement and utilize empty constructors can significantly streamline your coding process. So, the next time you find yourself needing a default initialization, consider using an empty constructor to make your life easier!
FAQ
-
What is an empty constructor in C++?
An empty constructor is a constructor that does not take any parameters and does not contain any code, used for default initialization of class objects. -
When should I use an empty constructor?
You should use an empty constructor when you want to create objects without needing to provide initial values, allowing for later initialization. -
Can an empty constructor initialize member variables?
While an empty constructor does not initialize member variables by default, you can add code to do so if needed. -
How does an empty constructor improve code readability?
An empty constructor simplifies object creation, making the code cleaner and easier to understand, especially in complex applications. -
Are there any downsides to using empty constructors?
The main downside is that if you forget to initialize member variables later, it may lead to undefined behavior, so it’s important to ensure proper initialization at some point.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook