How to Add Int to String in C++
-
Use the
+=
Operator andstd::to_string
Function to Append Int to String -
Use
std::stringstream
to Add Int to String -
Use the
append()
Method to Add Int to String -
Use the
sprintf
Method to Add Int to String -
Use Boost’s
lexical_cast
to Add Int to String - Conclusion
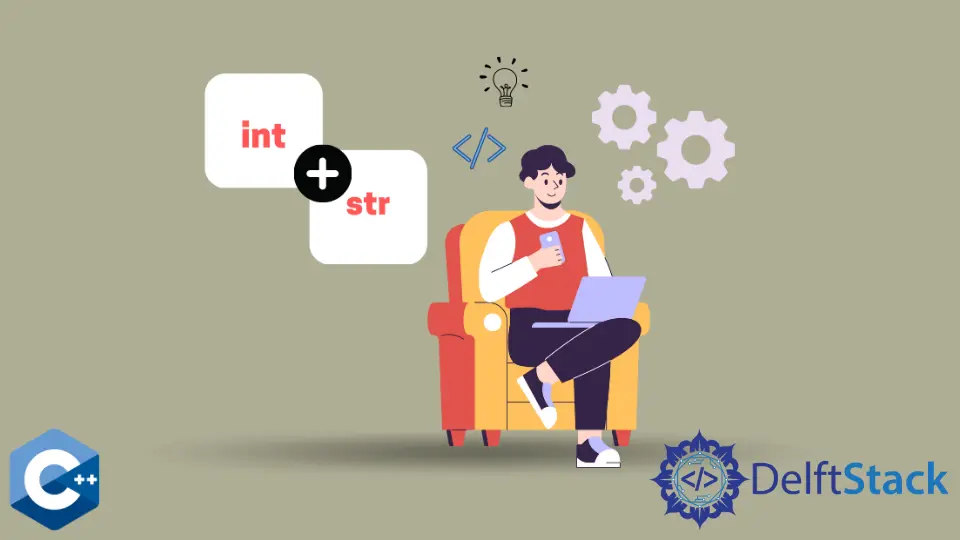
Appending an integer to a string is a fundamental operation in programming, often required for creating formatted output or constructing messages. In C++, there are several approaches to achieve this.
In this article, we will explore five different methods to add or append an integer to a string, each with its advantages and use cases.
Use the +=
Operator and std::to_string
Function to Append Int to String
In C++, the std::string
class provides robust support for concatenation using core operators such as +
and +=
. Among these, the +=
operator stands out as an elegant and efficient solution for appending content to an existing string.
In scenarios where an integer or a floating-point number needs to be appended to a string, the std::to_string
function comes in handy to convert the numerical value into a string before concatenation.
This function can convert various numerical types, such as integers, floats, doubles, and longs, into their string representations. This conversion allows for easy manipulation and incorporation of numerical data into strings.
Syntax:
std::string to_string( int value );
The std::to_string()
function takes a numerical value as an argument and returns a string representing that value.
Let’s explore a practical demonstration of this approach:
#include <iostream>
#include <string>
int main() {
std::string app_str = "This string will be appended to ";
int number = 12345;
app_str += std::to_string(number);
std::cout << app_str << std::endl;
return 0;
}
In this example, we begin with a string, app_str
, initialized with a base text.
We then concatenate an integer, number
, to the string using the +=
operator after converting it to a string using std::to_string(number)
. The result is displayed using std::cout
.
Output:
This string will be appended to 12345
This method is not limited to integers alone; it works with floating-point numbers as well. Here’s a similar example demonstrating concatenation with a floating-point number:
#include <iostream>
#include <string>
int main() {
std::string app_str = "This string will be appended to ";
float fnumber = 12.345;
app_str += std::to_string(fnumber);
std::cout << app_str << std::endl;
return 0;
}
Output:
This string will be appended to 12.345
This code is similar to the previous one but with a small difference in the type of the given variable. In the first code snippet, the numerical value is of type int
, whereas in the second code snippet, it is of type float
.
In both cases, the program converts a numerical value (int
or float
) to its string representation using std::to_string
. It then appends this string representation to an original string.
Finally, it prints the updated string to the standard output.
Use std::stringstream
to Add Int to String
Another way to append an integer to a string is by using std::stringstream
, a versatile stream class in C++ that is defined in the <sstream>
header.
It provides capabilities for input and output operations on strings as if they were standard input/output streams. This includes reading from a string, writing to a string, and formatting data into strings.
It’s particularly useful when you need to construct a string from different data types.
Let’s go through the important steps involved in using std::stringstream
to append an integer to a string:
-
To use
std::stringstream
, you first need to create an instance of thestd::stringstream
class. This object will serve as the intermediary buffer for the string operations.std::stringstream tmp_stream;
-
The
<<
operator is overloaded for thestd::stringstream
class, allowing you to easily insert data into the stringstream. This is similar to using<<
withstd::cout
.tmp_stream << data;
In our case, we want to insert an integer, so we use
<<
to insert the integer into thestd::stringstream
object. -
To retrieve the string representation of the integer, we use the
str()
member function of thestd::stringstream
. This function returns a string that represents the content currently stored in the stream.tmp_stream.str();
Here’s the complete example:
#include <iostream>
#include <string>
#include <sstream>
int main() {
std::string app_str = "This string will be appended to ";
int number = 12345;
std::stringstream tmp_stream;
tmp_stream << app_str << number;
std::cout << tmp_stream.str() << std::endl;
return 0;
}
Output:
This string will be appended to 12345
In this example, two variables are initialized: app_str
and number
. app_str
is a string holding the starting text, and number
is an integer set to 12345
.
A std::stringstream
object called tmp_stream
is created to facilitate string operations. The <<
operator, overloaded for std::stringstream
, is used to append the integer number
to the string app_str
.
This operation effectively concatenates the two values.
Subsequently, the str()
member function of the std::stringstream
object is employed to retrieve the concatenated string. Finally, the resulting concatenated string is displayed using std::cout
.
Use the append()
Method to Add Int to String
The append()
method provides another efficient way to append integers to strings. It is a member function of the std::basic_string
class, offering various forms of appending operations based on the parameters provided.
The append()
method, when invoked on a string object, allows for appending additional characters or strings to the existing string. Its functionality is versatile, accepting different types of parameters to facilitate various appending operations.
In the simplest form, when a single string argument is passed to append()
, it appends the provided string to the object from which the method is called. Alternatively, it can accept a single character and an integer count, appending the specified count of the given character.
Before we delve into the practical example, let’s outline the syntax of the append()
method:
string& append(const string& str); // Appends the string 'str' to the current string
string& append(const char* s); // Appends the C-style string 's' to the current string
string& append(size_t n, char c); // Appends 'n' occurrences of character 'c' to the current string
string& append(const string& str, size_t pos, size_t len); // Appends a substring of 'str' starting from index 'pos' and up to 'len' characters to the current string
Syntax:
string& append(const string& str);
string&
: This part of the syntax indicates thatappend()
returns a reference to a string. The&
symbol represents a reference, allowing the method to modify the original string it is called on. The returned reference is essential to enable chaining of multipleappend()
calls.const string& str
: Here,str
is a parameter of typeconst string&
, meaning it’s a constant reference to a string. It specifies the string that you want to append to the original string. Theconst
qualifier means that thestr
parameter cannot be modified within theappend()
method. This version ofappend()
is used to append the entire content of one string to another.
For a comprehensive list of parameters and options that append()
offers, refer to the C++ reference manual.
Let’s see an example that showcases the usage of the append()
method to concatenate an integer with a string:
#include <iostream>
#include <string>
int main() {
std::string app_str = "This string will be appended to ";
int number = 12345;
app_str.append(std::to_string(number));
std::cout << app_str << std::endl;
return 0;
}
Output:
This string will be appended to 12345
Here, a string app_str
is initialized with a starting text, and an integer number
is set to 12345
. The append()
method is then employed on app_str
, appending the string representation of the integer using std::to_string(number)
.
Finally, the updated string is displayed on the console using std::cout
.
Use the sprintf
Method to Add Int to String
The sprintf
function is a part of the C Standard Library, available in C++ as well, and is typically used for string formatting. It’s similar to printf
in C, but instead of printing to the console, it writes the formatted output into a string.
This makes it convenient for constructing strings with specific formats. The general syntax for sprintf
is as follows:
int sprintf(char* str, const char* format, ...);
char* str
: A pointer to the destination character array where the resulting string will be stored.const char* format
: A format string specifying how the subsequent arguments are formatted and inserted into the string....
: Optional arguments to be formatted based on the format string.
The format string typically consists of placeholders (format specifiers) for different types of data that will be inserted into the string. For example, %d
is a format specifier for an integer, %f
for a float, %s
for a string, etc.
These format specifiers are replaced by the corresponding arguments provided after the format string in the sprintf
call.
Let’s delve into the code to understand how sprintf
can be used to append an integer to a string:
#include <cstdio>
#include <iostream>
#include <string>
int main() {
std::string originalString = "This string will be appended to ";
int num = 12345;
char buffer[100];
sprintf(buffer, "%s%d", originalString.c_str(), num);
std::string result(buffer);
std::cout << result << std::endl;
return 0;
}
Output:
This string will be appended to 12345
In this example, we start by initializing a string originalString
with a predefined text. The integer num
is set to 12345
, representing the value we want to append to the string.
The sprintf
function is then employed to format and append the integer to the original string.
In this case, the format string is " %s%d"
, where %s
is a placeholder for a string (originalString.c_str()
) and %d
is a placeholder for an integer (num
). This results in the concatenation of the string and the integer, forming the final formatted string.
After formatting, the result is stored in a character buffer buffer
. To use it as a C++ string, we convert the buffer to a string using std::string result(buffer)
.
Finally, the concatenated string is displayed on the console using std::cout
.
Use Boost’s lexical_cast
to Add Int to String
boost::lexical_cast
is a versatile utility provided by the Boost library. It simplifies the process of converting one data type to another, making conversions easy and intuitive.
This utility is especially helpful when you need to convert between fundamental types, like integers to strings, and vice versa. lexical_cast
operates similarly to the standard library functions, such as std::to_string
, but offers a more flexible approach by handling various data types.
The code provided below demonstrates how to use boost::lexical_cast
to append an integer to a string. Let’s understand the key parts of the code:
#include <iostream>
#include <string>
#include <boost/lexical_cast.hpp>
int main() {
std::string originalString = "This string will be appended to ";
int num = 12345;
originalString += boost::lexical_cast<std::string>(num);
std::cout << originalString << std::endl;
return 0;
}
Output:
This string will be appended to 12345
In the provided code example, we begin by including essential headers, such as <iostream>
for input/output, <string>
for string manipulation, and <boost/lexical_cast.hpp>
for utilizing Boost’s lexical_cast
functionality.
We then declare a string variable named originalString
, which serves as the base string to which we intend to append an integer. Additionally, we initialize an integer variable num
with the value 12345
, representing the integer we want to append.
The key operation occurs where we use boost::lexical_cast<std::string>(num)
to convert the integer num
to its string representation. This string representation is then appended to the originalString
using the +=
operator.
Finally, we display the updated string by printing it to the console using std::cout
.
Conclusion
Each of the methods discussed in this article offers a unique way to append an integer to a string. Understanding and mastering these methods equips you with the flexibility to choose the most appropriate approach based on your specific requirements and preferences.
Using standard C++ functions like std::to_string()
, stream manipulation with std::stringstream
, or Boost’s versatile lexical_cast
, you now have a range of options to handle this operation effectively in your C++ projects.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++