如何在 C++ 中向字串新增整數
Jinku Hu
2023年10月12日
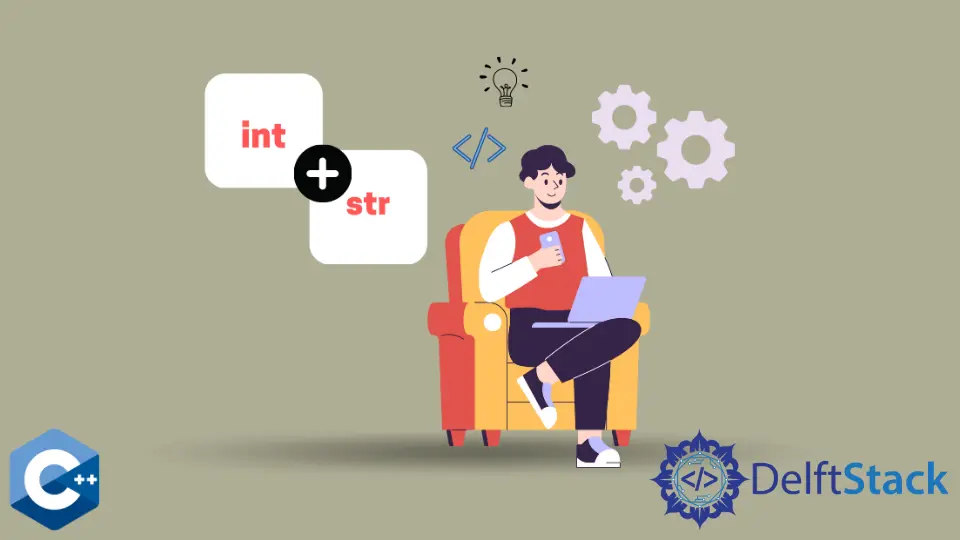
本文將講解幾種在 C++ 中向字串新增整數的方法。
使用+=
運算子和 std::to_string
函式將整型新增到字串中
std::string
類支援使用+
和+=
等核心運算子進行最常見的連線形式。在下面的例子中,我們演示了後一種,因為它是最佳解決方案。在將 int
值追加到字串的末尾之前,應該將 int
值轉換為相同的型別,這可以通過 std::to_string
函式呼叫來實現。
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
int main() {
string app_str = "This string will be appended to ";
int number = 12345;
cout << app_str << endl;
app_str += to_string(number);
cout << app_str << endl;
return EXIT_SUCCESS;
}
輸出:
This string will be appended to
This string will be appended to 12345
上述方法也與浮點數相容,如下面的程式碼示例所示。
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
int main() {
string app_str = "This string will be appended to ";
float fnumber = 12.345;
cout << app_str << endl;
app_str += to_string(fnumber);
cout << app_str << endl;
return EXIT_SUCCESS;
}
輸出:
This string will be appended to
This string will be appended to 12.345000
使用 std::stringstream
將整型新增到字串中
stringstream
可以將多種輸入型別並以字串格式儲存。它提供了易於使用的操作符和內建的方法來將構建的字串重定向到控制檯輸出。
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::to_string;
int main() {
string app_str = "This string will be appended to ";
int number = 12345;
stringstream tmp_stream;
cout << app_str << endl;
tmp_stream << app_str << number;
cout << tmp_stream.str() << endl;
return EXIT_SUCCESS;
}
輸出:
This string will be appended to
This string will be appended to 12345
使用 append()
方法將整型新增到字串中
append()
是 std::basic_string
類的一個成員函式,它可以根據引數的指定進行多種型別的追加操作。在最簡單的形式下,當傳遞一個字串引數時,它將追加到呼叫該方法的物件上。作為另一種形式,它可以接受一個單一的字元和整數,代表給定字元的追加計數。我們可以在手冊中看到完整的引數列表。
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
int main() {
string app_str = "This string will be appended to ";
int number = 12345;
cout << app_str << endl;
app_str.append(to_string(number));
cout << app_str;
return EXIT_SUCCESS;
}
輸出:
This string will be appended to
This string will be appended to 12345
作者: Jinku Hu