How to Initialize an Array to 0 in C
- Method 1: Using the Static Initialization
- Method 2: Using a Loop
-
Method 3: Using
memset
- Method 4: Using Compound Literals
- Conclusion
- FAQ
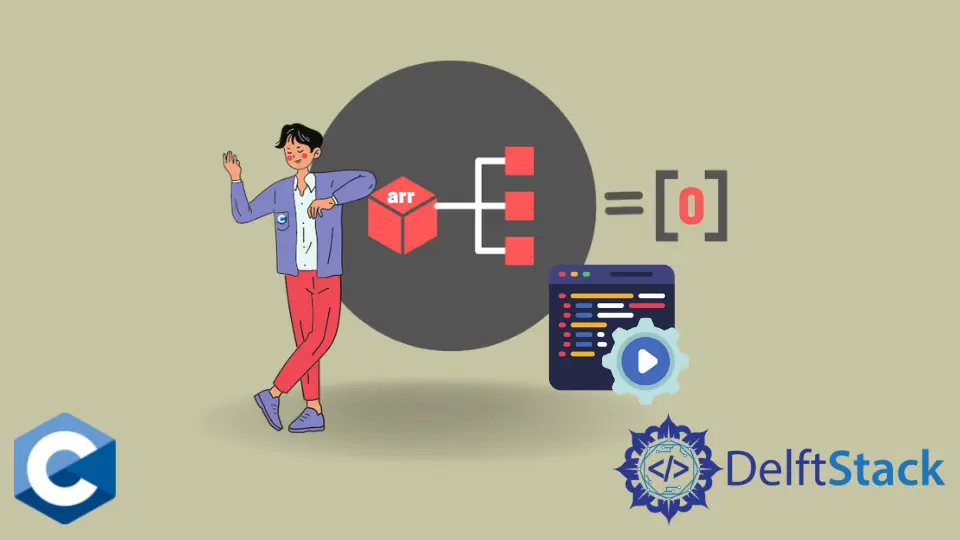
When programming in C, one common task is initializing arrays. Specifically, you might want to set all the elements of an array to zero. This is a fundamental operation that can be done in several ways, each with its own advantages and scenarios where it shines. Understanding how to initialize an array to zero is crucial for avoiding undefined behavior and ensuring your program runs smoothly.
In this tutorial, we will explore different methods to achieve this, providing clear examples and explanations. Whether you’re a beginner or looking to refresh your knowledge, this guide will help you master array initialization in C.
Method 1: Using the Static Initialization
One of the simplest ways to initialize an array to zero in C is through static initialization. When you declare an array and set it to zero during its declaration, all its elements are automatically initialized to zero.
#include <stdio.h>
int main() {
int arr[5] = {0};
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
return 0;
}
Output:
0 0 0 0 0
In this code, we declare an integer array arr
of size 5 and initialize it with {0}
. This tells the compiler to set the first element to zero, and since we didn’t specify values for the other elements, they are implicitly initialized to zero as well. This method is straightforward and effective for small arrays or when you know the size at compile time.
Method 2: Using a Loop
Another commonly used method is to initialize an array using a loop. This approach is particularly useful when you need to initialize an array dynamically or when working with larger arrays.
#include <stdio.h>
int main() {
int arr[5];
for (int i = 0; i < 5; i++) {
arr[i] = 0;
}
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
return 0;
}
Output:
0 0 0 0 0
In this example, we first declare the integer array arr
without initializing it. We then use a for
loop to iterate through each element of the array, setting it to zero. Finally, we print the array elements. This method gives you more control, allowing you to initialize arrays of any size or even conditionally set values based on specific criteria.
Method 3: Using memset
The memset
function from the C standard library is another effective way to initialize an array to zero. This method is particularly efficient for larger arrays, as it can set a block of memory to a specific value in a single function call.
#include <stdio.h>
#include <string.h>
int main() {
int arr[5];
memset(arr, 0, sizeof(arr));
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
return 0;
}
Output:
0 0 0 0 0
Here, we include the string.h
header to use memset
. The function takes three parameters: the pointer to the memory block (in this case, the array arr
), the value to set (0), and the number of bytes to set (using sizeof(arr)
). This method is particularly useful for larger arrays because it can initialize the entire array in a single call, saving time and effort compared to looping through each element.
Method 4: Using Compound Literals
C99 introduced compound literals, which allow you to initialize an array to zero in a more concise way. This method is handy when you want to create an array and initialize it at the same time.
#include <stdio.h>
int main() {
int *arr = (int[]){0, 0, 0, 0, 0};
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
return 0;
}
Output:
0 0 0 0 0
In this example, we create an array using a compound literal and initialize it to zero. The array is dynamically allocated on the stack, and we can access its elements just like a regular array. This method is particularly useful for temporary arrays or when you want to avoid the overhead of declaring a full array.
Conclusion
Initializing an array to zero in C is a fundamental skill that every programmer should master. Whether you choose static initialization, loops, memset
, or compound literals, each method has its advantages depending on the context of your program. By understanding these techniques, you can ensure that your arrays are properly initialized, avoiding potential bugs and undefined behavior. Remember that the method you choose can depend on the size of the array and your specific programming needs. Happy coding!
FAQ
-
What is the default value of an uninitialized array in C?
An uninitialized array in C contains garbage values, which can lead to unpredictable behavior in your program. -
Can I initialize a multi-dimensional array to zero in C?
Yes, you can initialize a multi-dimensional array to zero using similar methods, such as static initialization or loops. -
Is
memset
safe for all data types?
memset
is safe for initializing arrays of simple data types like integers or characters, but it should be used cautiously with complex data types. -
What happens if I only partially initialize an array?
If you partially initialize an array, the remaining elements will be set to zero automatically.
- Is there a performance difference between these methods?
Yes, usingmemset
can be more efficient for large arrays compared to looping through each element, especially for larger data types.