How to Compare Strings in C
- Understanding String Representation in C
- Using the strcmp Function
- Using the strncmp Function
-
Use
strcasecmp
andstrncasecmp
Functions to Compare Strings Ignoring the Case of Letters - Using Manual Comparison
- Conclusion
- FAQ
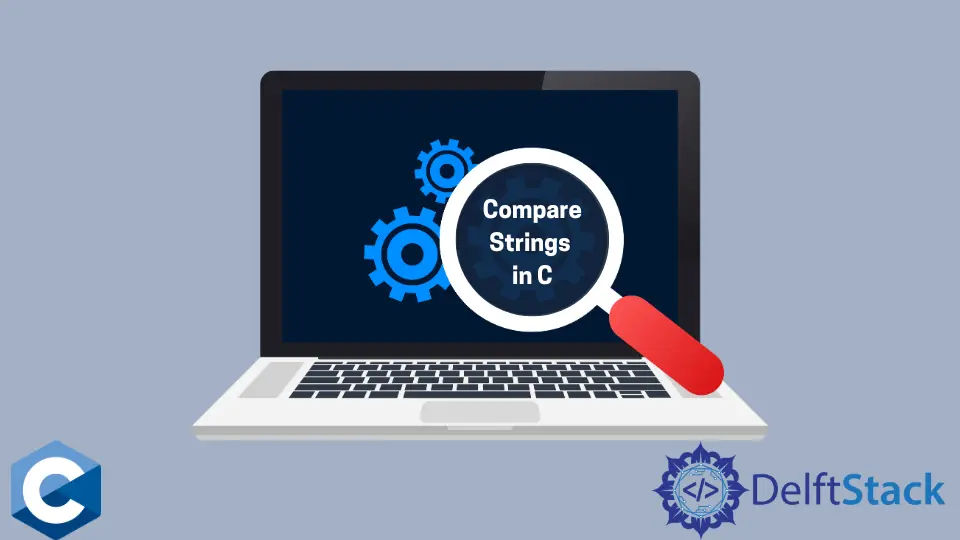
Comparing strings in C can be a bit tricky, especially for beginners. Unlike higher-level languages like Python, C does not have a built-in string type. Instead, strings in C are represented as arrays of characters terminated by a null character. This means that you need to use specific functions to compare these arrays effectively.
In this article, we will explore various methods to compare strings in C, including the use of standard library functions. By the end, you will have a solid understanding of how to handle string comparisons in your C programs, enabling you to write more efficient and effective code.
Understanding String Representation in C
Before diving into the methods for comparing strings, it’s essential to understand how strings are represented in C. A string in C is essentially an array of characters. Each character is stored in contiguous memory locations, and the end of the string is marked by a null character ('\0'
). This representation is crucial because it influences how we compare strings.
For instance, when comparing two strings, we need to ensure that we are comparing each character in the arrays until we reach the null terminator. This is different from languages like Python, where strings are first-class objects with built-in comparison operators.
Using the strcmp Function
One of the most common methods for comparing strings in C is using the strcmp
function from the C standard library. This function compares two strings lexicographically. It returns zero if the strings are equal, a negative value if the first string is less than the second, and a positive value if the first string is greater than the second.
Here’s how you can use strcmp
:
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "Hello";
char str2[] = "World";
int result = strcmp(str1, str2);
if (result == 0) {
printf("Strings are equal.\n");
} else if (result < 0) {
printf("'%s' is less than '%s'.\n", str1, str2);
} else {
printf("'%s' is greater than '%s'.\n", str1, str2);
}
return 0;
}
Output:
'Hello' is less than 'World'.
The strcmp
function takes two strings as arguments and performs a character-by-character comparison. If the strings match, it returns 0. If they differ, it returns a value based on the difference of the first non-matching character’s ASCII values. This makes it easy to determine not just equality but also the lexicographical order of the strings.
Using the strncmp Function
Sometimes, you may want to compare only a portion of two strings. This is where the strncmp
function comes in handy. It works similarly to strcmp
, but it allows you to specify the number of characters to compare. This can be particularly useful when you want to compare prefixes or when dealing with strings of different lengths.
Here’s an example of how to use strncmp
:
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "HelloWorld";
char str2[] = "HelloEveryone";
int result = strncmp(str1, str2, 5);
if (result == 0) {
printf("The first 5 characters are equal.\n");
} else {
printf("The first 5 characters are not equal.\n");
}
return 0;
}
Output:
The first 5 characters are equal.
In this example, we compare only the first five characters of str1
and str2
. The function returns 0, indicating that the substrings are equal. This is a powerful feature that allows for more granular control over string comparisons.
Use strcasecmp
and strncasecmp
Functions to Compare Strings Ignoring the Case of Letters
strcasecmp
function behaves similarly to the strcmp
function except that it ignores the letter case. This function is POSIX compliant and can be utilized on multiple operating systems along with strncasecmp
, which implements the case insensitive comparison on the certain number of characters in both strings. The latter parameter can be passed to the function with the third argument of type size_t
.
Notice that the return values of these functions can be used directly in conditional statements.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
const char* str1 = "hello there 2";
const char* str3 = "Hello there 2";
!strcasecmp(str1, str3) ? printf("strings are equal\n")
: printf("strings are not equal\n");
!strncasecmp(str1, str3, 5) ? printf("strings are equal\n")
: printf("strings are not equal\n");
exit(EXIT_SUCCESS);
}
Output:
strings are equal
strings are equal
In this example, we demonstrate case-insensitive string comparisons. First, strcasecmp
compares the entire strings str1
and str3
without considering case differences. The function returns 0, indicating that “hello there 2” and “Hello there 2” are considered equal when case is ignored, hence “strings are equal” is printed.
Next, we use strncasecmp
to compare only the first five characters of str1
and str3
, also ignoring case. The function again returns 0, signifying that the substrings “hello” and “Hello” are equal. This showcases the ability to perform case-insensitive comparisons while also limiting the comparison to a specific number of characters, providing flexibility and precision in string handling.
Using Manual Comparison
While using library functions is the most straightforward approach, you can also manually compare strings. This method involves iterating over each character of the strings and comparing them one by one. This technique can be useful for educational purposes or in situations where you want to avoid using standard library functions.
Here’s how you can implement a manual comparison:
#include <stdio.h>
int compareStrings(const char *str1, const char *str2) {
while (*str1 && (*str1 == *str2)) {
str1++;
str2++;
}
return *(unsigned char *)str1 - *(unsigned char *)str2;
}
int main() {
char str1[] = "Hello";
char str2[] = "HelloWorld";
int result = compareStrings(str1, str2);
if (result == 0) {
printf("Strings are equal.\n");
} else if (result < 0) {
printf("'%s' is less than '%s'.\n", str1, str2);
} else {
printf("'%s' is greater than '%s'.\n", str1, str2);
}
return 0;
}
Output:
'Hello' is less than 'HelloWorld'.
In this code, we define a function compareStrings
that takes two string pointers as arguments. It uses a loop to compare each character until it finds a mismatch or reaches the end of either string. This method gives you a clear understanding of how string comparison works under the hood.
Conclusion
Comparing strings in C is a fundamental skill that every programmer should master. Whether you choose to use built-in functions like strcmp
and strncmp
or implement your own comparison logic, understanding how strings work in C will enhance your coding capabilities. As you continue to develop your skills in C programming, keep these methods in mind to handle string comparisons effectively.
FAQ
-
What is the difference between
strcmp
andstrncmp
?
strcmp
compares two strings completely, whilestrncmp
compares only a specified number of characters. -
How do I compare strings of different lengths?
You can usestrcmp
orstrncmp
; they will handle differences in length by returning a non-zero value if the strings differ. -
Can I compare strings without using library functions?
Yes, you can manually compare strings by iterating through each character, as demonstrated in the manual comparison method. -
What happens if I compare uninitialized strings?
Comparing uninitialized strings can lead to undefined behavior, as their contents are unpredictable. Always ensure strings are initialized before comparison. -
Are there any performance implications when comparing long strings?
Yes, comparing longer strings may take more time, especially if using manual comparison. Using optimized library functions is generally more efficient.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook