C 語言中比較字串
Jinku Hu
2023年10月12日
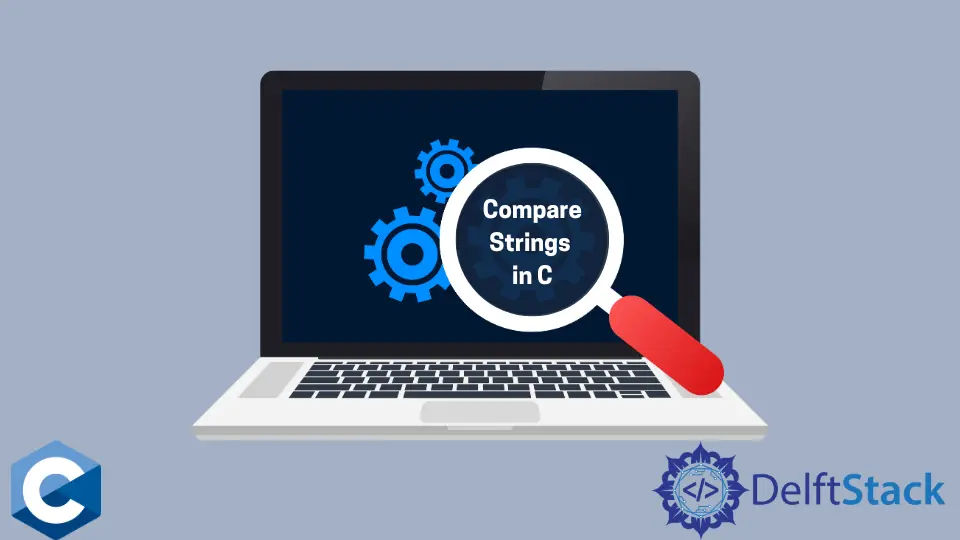
本文將介紹關於如何在 C 語言中比較字串的多種方法。
使用 strcmp
函式比較字串
strcmp
函式是定義在 <string.h>
頭的標準庫函式。C 風格的字串只是以 0
符號結束的字元序列,所以函式必須對每個字元進行迭代比較。
strcmp
接受兩個字元字串,並返回整數來表示比較的結果。如果第一個字串在詞法上小於第二個字串,則返回的數字為負數,如果後一個字串小於前一個字串,則返回的數字為正數,如果兩個字串相同,則返回的數字為 0
。
需要注意的是,在下面的例子中,我們將函式的返回值反轉,並將其插入到 ?:
條件語句中,列印相應的輸出到控制檯。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
const char* str1 = "hello there 1";
const char* str2 = "hello there 2";
const char* str3 = "Hello there 2";
!strcmp(str1, str2) ? printf("strings are equal\n")
: printf("strings are not equal\n");
!strcmp(str1, str3) ? printf("strings are equal\n")
: printf("strings are not equal\n");
exit(EXIT_SUCCESS);
}
輸出:
strings are not equal
strings are not equal
使用 strncmp
函式只比較部分字串
strncmp
是定義在 <string.h>
標頭檔案中的另一個有用的函式,它可以用來只比較字串開頭的幾個字元。
strncmp
的第三個引數為整數型別,用於指定兩個字串中要比較的字元數。該函式的返回值與 strcmp
返回的值類似。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
const char* str1 = "hello there 1";
const char* str2 = "hello there 2";
!strncmp(str1, str2, 5) ? printf("strings are equal\n")
: printf("strings are not equal\n");
exit(EXIT_SUCCESS);
}
輸出:
strings are equal
使用 strcasecmp
和 strncasecmp
函式比較忽略字母大小寫的字串
strcasecmp
函式的行為與 strcmp
函式類似,但它忽略字母大小寫。該函式與 POSIX 相容,可與 strncasecmp
一起在多個作業系統上使用,後者實現了對兩個字串中一定數量的字元進行不區分大小寫的比較。後者的引數可以和型別為 size_t
的第三個引數一起傳遞給函式。
注意,這些函式的返回值可以直接用於條件語句中。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
const char* str1 = "hello there 2";
const char* str3 = "Hello there 2";
!strcasecmp(str1, str3) ? printf("strings are equal\n")
: printf("strings are not equal\n");
!strncasecmp(str1, str3, 5) ? printf("strings are equal\n")
: printf("strings are not equal\n");
exit(EXIT_SUCCESS);
}
輸出:
strings are equal
strings are equal
作者: Jinku Hu