How to Convert Char to String in Arduino
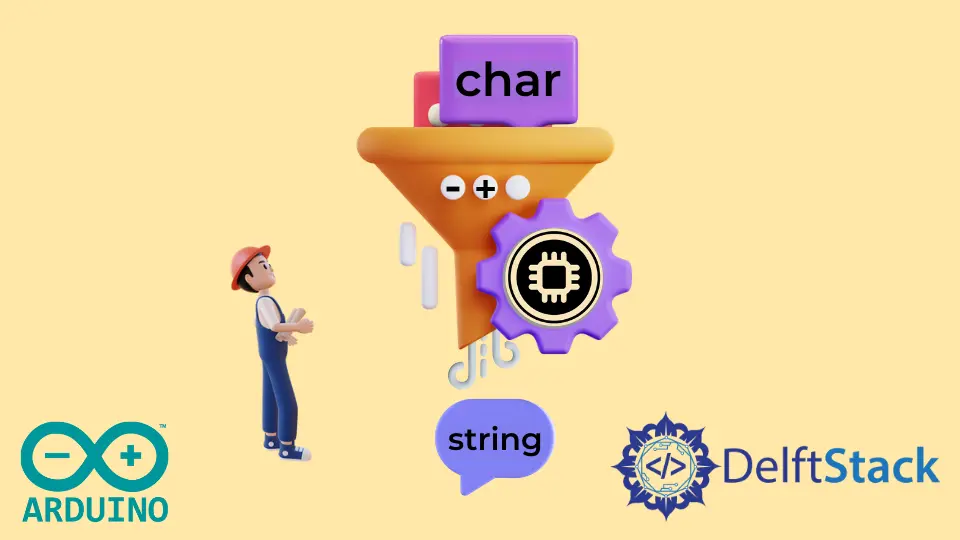
Converting a char to a string in Arduino is a common task that many developers encounter. Whether you are working on a simple project or a complex application, understanding how to manipulate character data is essential. In Arduino, the process is straightforward and can be accomplished using built-in functions like String()
and Serial.readString()
. These methods allow you to seamlessly convert individual characters into strings, making it easier to handle text data for display, storage, or communication.
In this article, we’ll delve into the various ways to convert char to string in Arduino, providing you with practical examples and clear explanations.
Method 1: Using the String()
Function
The String()
function in Arduino is a powerful tool for converting a character into a string. This function takes a single character as input and returns a string representation of that character. This method is particularly useful when you want to create a string from a single char variable.
Here’s how you can use the String()
function:
char myChar = 'A';
String myString = String(myChar);
Serial.begin(9600);
Serial.println(myString);
Output:
A
In this code snippet, we first declare a character variable myChar
and assign it the value ‘A’. We then use the String()
function to convert myChar
into a string and store it in the variable myString
. Finally, we initialize the serial communication and print the string to the serial monitor. The output will display the character ‘A’ as a string.
This method is straightforward and efficient for converting single characters. However, if you need to convert multiple characters or read characters from user input, you might want to consider other methods, such as using Serial.readString()
.
Method 2: Using Serial.readString() Function
The Serial.readString()
function is another effective way to convert characters to strings in Arduino. This function reads a string from the serial input until a timeout occurs or a newline character is received. It is particularly useful for capturing user input or data from sensors.
Let’s see how to implement this:
void setup() {
Serial.begin(9600);
}
void loop() {
if (Serial.available() > 0) {
String inputString = Serial.readString();
Serial.print("You entered: ");
Serial.println(inputString);
}
}
Output:
You entered: Hello
In this example, we first set up serial communication at a baud rate of 9600. The loop()
function checks if there is any data available on the serial port. If data is available, it reads the input using Serial.readString()
and stores it in the inputString
variable. The program then prints the entered string back to the serial monitor.
This method is particularly beneficial when you want to capture entire strings of text rather than just single characters. It allows for more flexibility in handling user input, making it ideal for interactive projects.
Conclusion
Converting char to string in Arduino can be done easily using the String()
function or the Serial.readString()
function. Each method serves different purposes, with String()
being perfect for single characters and Serial.readString()
for capturing user input. By mastering these techniques, you can enhance your Arduino projects and improve your ability to manipulate text data efficiently. With practice, you’ll find that handling strings becomes second nature in your programming journey.
FAQ
-
How do I convert multiple characters to a string in Arduino?
You can concatenate multiple characters using theString()
function or by using the+=
operator to build your string incrementally. -
What is the difference between char and String in Arduino?
A char is a single character data type, while String is an object that can hold a sequence of characters. Strings can be manipulated more easily than individual chars. -
Can I convert a string back to a char in Arduino?
Yes, you can access individual characters in a String object using indexing, likemyString[0]
, to retrieve the first character. -
Is using String() function memory efficient in Arduino?
TheString()
function can lead to memory fragmentation in environments with limited RAM, like Arduino. For critical applications, consider using char arrays instead. -
How can I clear a String variable in Arduino?
You can clear a String variable by using theStringVariable = "";
syntax or theStringVariable.clear();
method.