How to Convert String to Char in Arduino
- Understanding Strings and Char Arrays in Arduino
-
Using the
toCharArray()
Function - Converting Other Data Types Using the Append Operator
- Conclusion
- FAQ
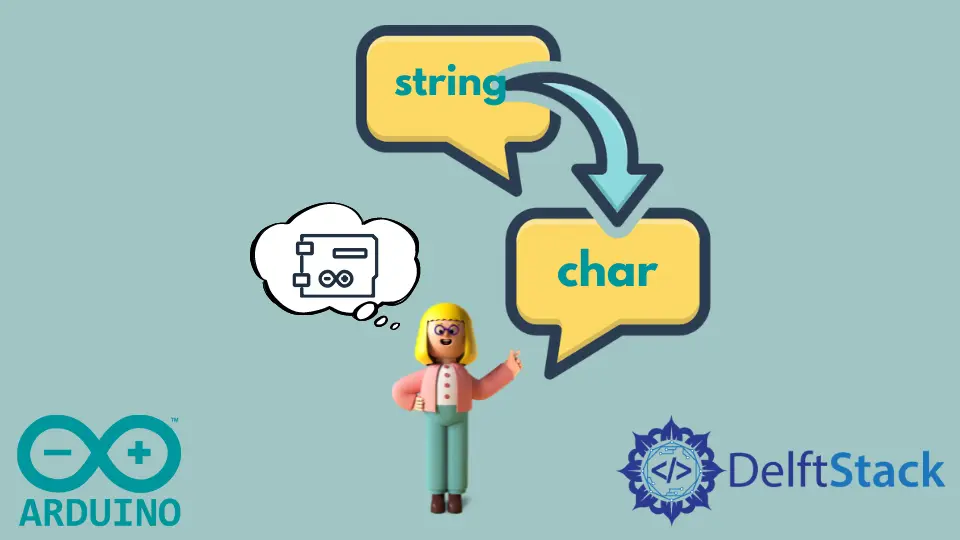
Converting a string to a character array in Arduino is a common task that many developers encounter. Whether you’re working on a simple project or a more complex application, understanding how to manipulate strings is crucial for effective programming. The primary way to convert a string to a char array in Arduino is by using the toCharArray()
function. Additionally, if you need to convert other data types to strings, the append operator is your best friend.
In this article, we’ll explore these methods in detail, provide clear examples, and help you grasp the fundamentals of string manipulation in Arduino programming.
Understanding Strings and Char Arrays in Arduino
In Arduino, a string is a sequence of characters that can be manipulated using various functions. Char arrays, on the other hand, are a lower-level representation of strings. They are often used for more efficient memory management and compatibility with C-style strings. When you need to pass data to functions that expect char arrays or when interfacing with hardware, converting strings to char arrays is essential.
The toCharArray()
function is specifically designed for this purpose. It takes a string and copies its contents into a specified char array, allowing you to work with the data in the format you need.
Using the toCharArray()
Function
The toCharArray()
function is straightforward to use. Here’s how you can implement it in your Arduino sketch.
String myString = "Hello, Arduino!";
char myCharArray[20];
myString.toCharArray(myCharArray, sizeof(myCharArray));
Serial.println(myCharArray);
Output:
Hello, Arduino!
In this example, we first declare a string variable myString
and initialize it with the text “Hello, Arduino!”. We then create a character array, myCharArray
, with a size of 20. The toCharArray()
function is called on myString
, passing in myCharArray
and its size as arguments. This copies the content of myString
into myCharArray
. Finally, we print the character array to the serial monitor.
Using toCharArray()
is efficient and ensures that you have a null-terminated string, which is crucial for many string manipulation functions in C and C++. This method is particularly useful when you need to send data over serial communication or when working with libraries that require char arrays.
Converting Other Data Types Using the Append Operator
Sometimes, you may need to convert other data types, such as integers or floats, into strings before converting them to char arrays. The append operator comes in handy here. Let’s see how you can achieve this.
String myString = "Temperature: ";
float temperature = 23.5;
myString += temperature;
char myCharArray[30];
myString.toCharArray(myCharArray, sizeof(myCharArray));
Serial.println(myCharArray);
Output:
Temperature: 23.5
In this code snippet, we start with a string myString
, initialized with “Temperature: “. We then declare a float variable temperature
and assign it a value of 23.5. Using the append operator (+=
), we concatenate the float value to the string. After that, we create a char array myCharArray
and use the toCharArray()
function to convert the string into a char array. The final output shows the complete message, including the temperature.
This method is beneficial when you are working with sensor data or any dynamic values that need to be displayed as strings. The append operator allows for seamless integration of multiple data types into a single string, which can then be easily converted to a char array.
Conclusion
In summary, converting strings to char arrays in Arduino is a fundamental skill that can enhance your programming capabilities. By using the toCharArray()
function, you can efficiently manage string data and ensure compatibility with various functions and libraries. Additionally, the append operator allows for the easy conversion of other data types into strings, making your code more versatile. Whether you’re a novice or an experienced programmer, mastering these techniques will undoubtedly improve your projects.
FAQ
-
What is the difference between String and char array in Arduino?
String is an object that provides various functions for string manipulation, while a char array is a lower-level representation of a string that requires manual memory management. -
Can I convert a number directly to a char array without using a string?
No, you typically convert a number to a string first and then usetoCharArray()
to convert that string to a char array. -
What happens if the char array size is smaller than the string length?
If the char array is smaller, it will not store the entire string, and you may encounter data loss or undefined behavior. -
Is using String in Arduino memory efficient?
Using String can lead to memory fragmentation in long-running applications, so it’s often recommended to use char arrays for better memory management. -
How can I clear a String in Arduino?
You can clear a String by using themyString = "";
syntax, which resets the String to an empty state.
Related Article - Arduino String
- Arduino strcmp Function
- Arduino Strcpy Function
- How to Concatenate Strings in Arduino
- How to Parse a String in Arduino
- How to Split String in Arduino
- How to Compare Strings in Arduino