How to Convert Float to String in Arduino
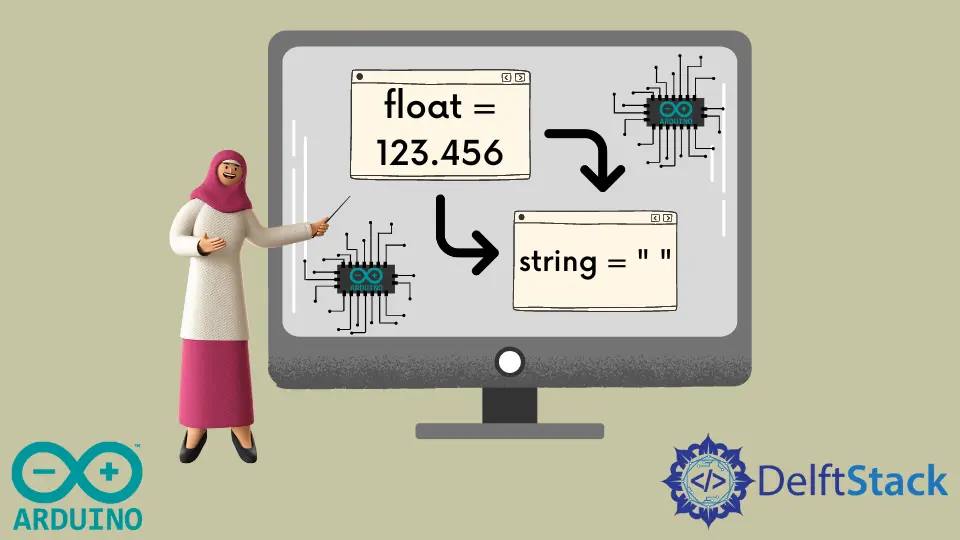
Converting a float to a string in Arduino can seem daunting at first, but with the right functions, it becomes a straightforward task. This process is essential for many applications, especially when you need to send data over a serial connection or display it on an LCD. In Arduino, the two primary methods to achieve this conversion are using the String()
function and the concat()
function. Understanding these methods will not only enhance your coding skills but also improve the efficiency of your projects.
In this article, we will delve into each method, providing clear examples and detailed explanations to ensure you can easily implement them in your own Arduino projects.
Method 1: Using the String()
Function
The String()
function is a simple and effective way to convert a float to a string in Arduino. This function takes a floating-point number as input and returns a string representation of that number. Here’s how you can use it:
void setup() {
Serial.begin(9600);
float myFloat = 3.14159;
String myString = String(myFloat);
Serial.println(myString);
}
void loop() {
}
Output:
3.14159
In this example, we start by initializing the serial communication at a baud rate of 9600. We define a float variable called myFloat
and assign it the value of Pi. The crucial part is the line where we convert the float to a string using String(myFloat)
. This creates a new string variable, myString
, that holds the string representation of the float. Finally, we print myString
to the serial monitor. This method is straightforward and works well for most cases, especially when you need to display the float value directly.
The String()
function is particularly useful because it automatically handles the conversion for you. However, keep in mind that excessive use of the String
class can lead to memory fragmentation in Arduino due to dynamic memory allocation. Therefore, it’s best used when necessary and in limited quantities.
Method 2: Using the concat()
Function
Another effective method to convert a float to a string in Arduino is by using the concat()
function. This function allows you to append a float to an existing string, which can be quite handy in certain situations. Here’s an example of how to use it:
void setup() {
Serial.begin(9600);
float myFloat = 2.71828;
String myString = "The value of e is: ";
myString.concat(myFloat);
Serial.println(myString);
}
void loop() {
}
Output:
The value of e is: 2.71828
In this code, we again start by initializing the serial communication. We define a float variable, myFloat
, assigned the value of Euler’s number. We also create a string variable, myString
, initialized with a message. The concat()
function is then used to append myFloat
to myString
. This results in the complete string, which we print to the serial monitor.
The concat()
method is particularly useful when you want to build a more complex string that includes both static text and dynamic float values. It provides flexibility in how you construct your output. However, similar to the String()
function, be cautious about using concat()
excessively, as it can lead to memory issues on microcontrollers with limited resources.
Conclusion
Converting floats to strings in Arduino using the String()
and concat()
functions is a crucial skill for any Arduino programmer. Both methods are straightforward and effective, allowing you to manipulate and display float values easily. While the String()
function is excellent for direct conversions, concat()
offers flexibility when constructing more complex strings. By understanding these techniques, you can enhance your Arduino projects, making them more interactive and user-friendly. Remember to use these methods judiciously to avoid memory issues, and you’ll be well on your way to mastering string manipulations in Arduino.
FAQ
-
How do I convert a float to a string in Arduino?
You can use theString()
function or theconcat()
function to convert a float to a string in Arduino. -
What is the difference between the
String()
function and concat()?
TheString()
function creates a new string from a float, while concat() appends a float to an existing string. -
Can I use these methods for other data types?
Yes, both methods can be used to convert various data types to strings in Arduino. -
Is there a memory concern when using String in Arduino?
Yes, excessive use of the String class can lead to memory fragmentation, so it’s best used sparingly. -
Can I format the float value before converting it to a string?
Yes, you can format float values using thedtostrf()
function before converting them to strings.