Python os.write() Method
-
Syntax of the
os.write()
Method -
Example 1: Use the
os.write()
Method to Write a Byte-String to a Given File Descriptor -
Example 2: Alternative Syntax of the
os.write()
Method -
Example 3: Use the
os.fdopen
Method With theos.write()
Method -
Example 4: Write to the Standard Output (
stdout
) Using theos.write()
Method - Conclusion
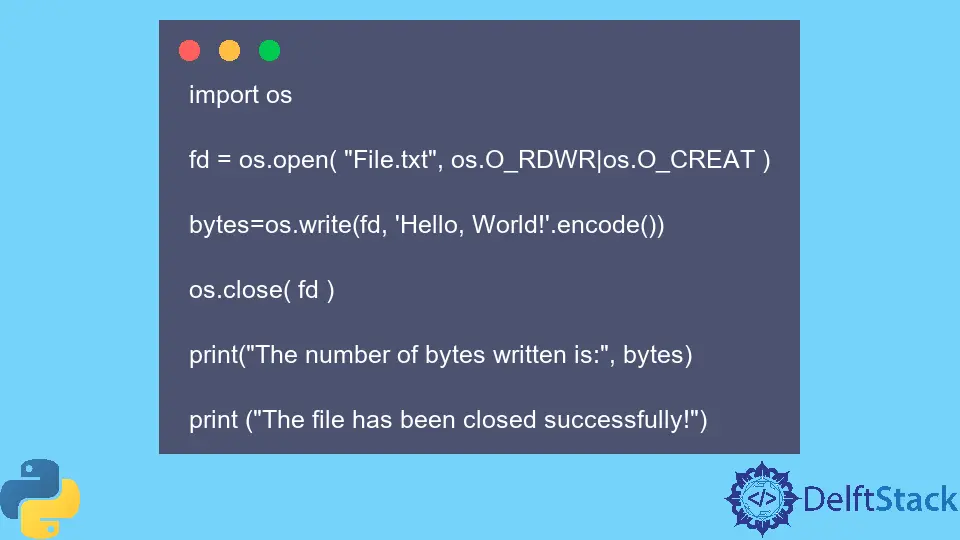
The Python os.write()
method is an efficient way of writing a byte of string to a specific file descriptor.
Syntax of the os.write()
Method
os.write(fd, string)
Parameters
Parameter | Description |
---|---|
fd |
It is an optional parameter representing a file descriptor referring to a path. |
string |
It is any byte of strings that needs to be written in the file. |
Return
The return type of this method is an integer value representing the number of bytes written successfully on a file.
Example 1: Use the os.write()
Method to Write a Byte-String to a Given File Descriptor
In the example code, we start by using the os.open()
function to open a file named "File.txt"
in both read and write mode (os.O_RDWR
) with the os.O_CREAT
flag, which creates the file if it doesn’t exist. We then encode the string "Hello, World!"
to bytes using .encode()
and write these bytes to the opened file descriptor (fd
) using os.write()
.
The number of bytes written, which is 13
, is stored in the variable bytes
. After writing the data, we close the file descriptor with os.close(fd)
to ensure proper cleanup.
import os
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
bytes = os.write(fd, "Hello, World!".encode())
os.close(fd)
print("The number of bytes written is:", bytes)
print("The file has been closed successfully!")
Output:
The number of bytes written is: 13
The file has been closed successfully!
The output of the code shows the number of bytes written, which is 13
, and confirms the successful closure of the file. This code effectively writes the "Hello, World!"
string to the File.txt
file and provides information about the number of bytes written and the closure status of the file.
The methods os.open()
and os.write()
go hand in hand because one has to open a file and write data. Data can’t be written into a file in another way.
Example 2: Alternative Syntax of the os.write()
Method
In this example code, we begin by opening a file named "File.txt"
in write mode ("w"
) using the open()
function. Then, we use the write()
method to write the "Hello, World!"
string to the file.
After writing, we close the file using file.close()
to ensure proper handling. Next, we reopen the same file, "File.txt"
, in read mode ("r"
) and assign it to the variable file
.
We then use the read()
method to read the content of the file. The content, Hello, World!
is printed on the console.
import os
file = open("File.txt", "w")
file.write("Hello, World!")
file.close()
file = open("File.txt", "r")
print(file.read())
Output:
Hello, World!
The output displays the content of the file, which is Hello, World!
. This code demonstrates a simple file write and read operation using standard Python file I/O functions, making it easy to create and read from a file.
It is a healthy practice for any programmer to close all the newly opened files using the os.close()
method.
Example 3: Use the os.fdopen
Method With the os.write()
Method
In this example code, we utilize the os.open()
function to open a file named "File.txt"
in both read and write mode with the os.O_CREAT
flag, which creates the file if it doesn’t exist, and os.O_RDWR
for read and write permissions. We then use os.fdopen()
to create a higher-level file object (fd
) from the file descriptor.
Next, we write the string "Hello, World!"
to the file using fd.write()
. The with
statement ensures that the file descriptor is automatically closed after the block’s execution.
After writing to the file, the code prints "The file descriptor has been closed successfully"
.
import os
with os.fdopen(os.open("File.txt", os.O_CREAT | os.O_RDWR), "w") as fd:
fd.write("Hello, World!")
print("The file descriptor has been closed successfully.")
Output:
The file descriptor has been closed successfully.
The output simply confirms the successful closure of the file descriptor, demonstrating proper resource management when working with low-level file descriptors in Python.
Example 4: Write to the Standard Output (stdout
) Using the os.write()
Method
import os
bytes_written = os.write(1, "Hello, World!".encode())
print("The number of bytes written is:", bytes_written)
print("The content has been written to stdout!")
Output
Hello, World!The number of bytes written is: 13
The content has been written to stdout!
The output is a combination of the content written and the print statements. It first displays "Hello, World!"
(the content written to stdout
), followed by "The number of bytes written is: 13"
, which indicates the number of bytes written to stdout.
Finally, it confirms that the content has been successfully written to stdout
, as specified in the second print statement.
Conclusion
The Python os.write()
method is a commonly used tool for low-level I/O operations and is often employed in conjunction with file descriptors obtained through os.open()
. The article presents several illustrative examples of its usage.
The first example demonstrates opening a file, writing data to it, and recording the number of bytes written while ensuring proper resource management. The second example employs standard open()
and write()
functions, which are more typical for everyday file I/O operations.
The third example introduces the os.fdopen()
method in combination with os.write()
, highlighting the importance of using the with
statement for resource cleanup. Lastly, the fourth example shows how os.write()
can be used to write data directly to the standard output (stdout
).
Python’s flexibility in providing both low-level and high-level I/O methods caters to diverse programming needs.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn