Python os.waitpid() Method
Musfirah Waseem
Jan 30, 2023
Python
Python OS
-
Syntax of Python
os.waitpid()
Method -
Example 1: Use the
os.waitpid()
Method in Python -
Example 2: Shorter Syntax of the
os.waitpid()
Method
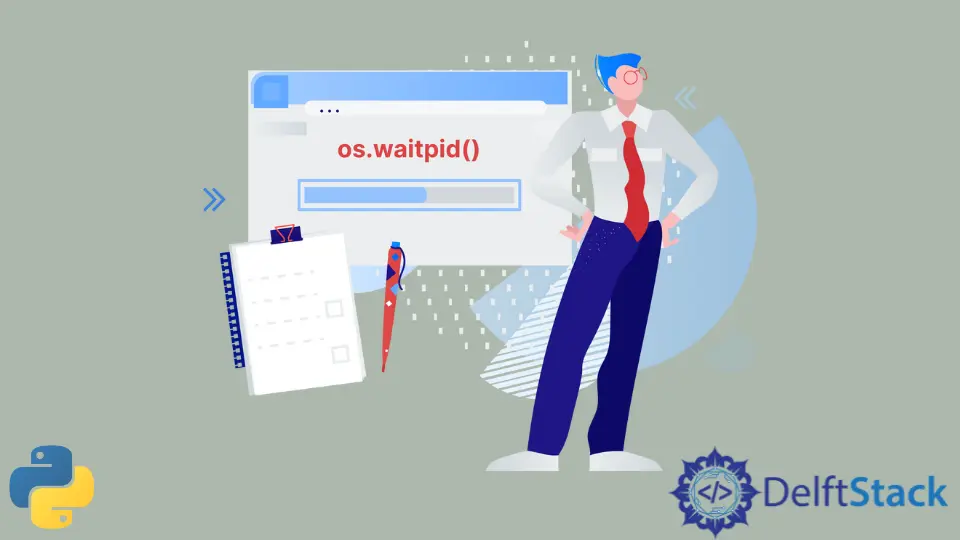
Python os.waitpid()
method is an efficient way for a process to wait for the completion of one or more of its child processes, as specified by the input parameter. The functionality of this method differs between UNIX and Windows OS.
Syntax of Python os.waitpid()
Method
os.waitpid(pid, options)
Parameters
pid |
It is an integer value representing the ID of the child process to wait for. On Windows OS, any pid less than or equal to 0 raises an exception. |
On the Windows system, the pid can refer to any process whose ID is available and not necessarily to the child process. However, on the UNIX system, the pid must refer to a child process. |
|
options |
It is an integer value representing what type of operations will be carried out on the process after it is completed. The default value is 0. |
Return
- On UNIX: The return type of this method is a tuple representing the process ID of the child process and the exit status.
- On Windows: The return type of this method is a tuple representing the process ID of any process, and the exit status is shifted to the left by 8 bits. It is shifted to the left so that cross-platform use of the function becomes easier.
Example 1: Use the os.waitpid()
Method in Python
import os
pid = os.fork()
if pid > 0:
process = os.waitpid(pid, 0)
print("\nParent process:")
print("Status of child process:", process)
else:
print("\nChild process:")
print("The child process ID:", os.getpid())
print("Hello, World!")
Output:
Child process:
The child process ID: 3186
Hello, World!
Parent process:
Status of child process: (3186, 0)
Note that the os.waitpid()
semantics are affected by the parameter options
value. In the above code, the integer value of options
is 0, which stands for normal operation.
Example 2: Shorter Syntax of the os.waitpid()
Method
import os
pid = os.fork()
if pid != 0:
print("Waiting for the child process to execute: ", os.waitpid(pid, 0))
Output:
Waiting for the child process to execute: (1505, 0)
On any Windows OS, when the process has exited, the id returned is the same ID, which is reusable.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn