Python os.umask() Method
-
Syntax of Python
os.umask()
Method -
What Is a
umask
in Python -
Example 1: Get the Current
umask
Using theos.umask()
Method in Python -
Example 2: Setting a New
umask
Using theos.umask()
Method in Python -
Example 3: Use Octal Representation in the
os.umask()
Method -
Example 4: Find the Permissions in the Current Working Directory Using the
os.umask()
Method - Use Cases
- Conclusion
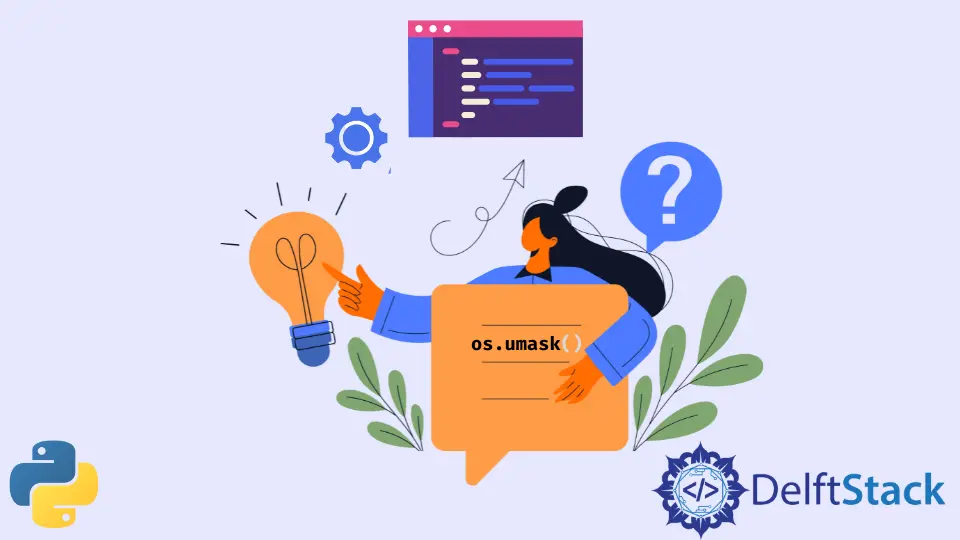
The os.umask()
method in Python is a function that sets or retrieves the current umask
. The umask
is a set of bits that determine the default permissions for new files or directories.
This method is part of the os
module, which provides a way to interact with the operating system.
Python’s os.umask()
method is an efficient way to assign the present numeric umask
value and retrieve the previous umask
value. Umask
determines the file permissions for any newly created folders or files.
Syntax of Python os.umask()
Method
The os.umask()
method is used to set or retrieve the current umask
value.
os.umask(mask)
Parameters
mask
: Optional. An integer representing the new umask
value to set.
Return Value
- If
mask
is specified, the method returns the previousumask
value. - If
mask
is not specified, it returns the currentumask
value without changing it.
What Is a umask
in Python
- The
umask
is a set of bits that determines the default permissions for newly created files or directories. - It works by subtracting the
umask
value from the maximum permissions (usually 777 for directories and 666 for files), resulting in the default permissions for new files and directories. - Each bit in the
umask
corresponds to specific permission: read (4
), write (2
), and execute (1
). - For instance, a
umask
of022
means that write permission (2
) will be removed for both groups and others, resulting in default permissions of 755 for directories and 644 for files.
Example 1: Get the Current umask
Using the os.umask()
Method in Python
This example uses the os.umask()
method to modify the current umask
setting and retrieve its value. The os.umask(0)
call sets the umask
to 0
temporarily and returns the previous umask
value, which is then printed using an f-string.
The umask()
function in Python sets the current umask
and returns the previous umask
. In this case, setting it to 0
temporarily sets the umask
to allow full permissions (read, write, execute) for the newly created files, and then it displays the previous umask
value before this change.
import os
current_umask = os.umask(0)
print(f"Current umask: {current_umask}")
Output:
Current umask: 18
Note that the input parameter of os.umask()
can be either an integer or an octal value.
Example 2: Setting a New umask
Using the os.umask()
Method in Python
This example showcases the use of os.umask()
to modify the umask
value. Specifically, it sets a new umask
value of 0o027
(octal notation), which denies write
permissions for the group and others.
Then, it retrieves and displays the previous umask
value before this modification.
import os
# Set a new umask of 0o027 (equivalent to 027 in octal)
new_umask = 0o027
previous_umask = os.umask(new_umask)
print(f"Previous umask: {previous_umask}")
Output:
Previous umask: 18
Example 3: Use Octal Representation in the os.umask()
Method
This example demonstrates the utilization of os.umask()
to set the umask
value to 0o777
(in octal notation). It then retrieves and displays both the current umask
value and the previous umask
value before the modification occurred.
import os
current_mask = 0o777
previous_umask = os.umask(current_mask)
print("The current umask value is:", current_mask)
print("The previous umask value is:", previous_umask)
Output:
The current umask value is: 511
The previous umask value is: 18
Note that the return value of os.umask()
will always be an integer, even if you enter an octal value as a parameter.
Example 4: Find the Permissions in the Current Working Directory Using the os.umask()
Method
This example sets the umask
value to 0o55
using os.umask()
, which changes the default permission settings for subsequently created files and directories within the program.
Then, it executes the shell command ls -alh > test
, which lists the directory contents in a long format and redirects the output to a file named test
.
Finally, it restores the previous umask
value using os.umask(previous_mask)
. This step reverts the umask
value back to what it was before changing it to 0o55
.
import os
previous_mask = os.umask(0o55)
os.system("ls -alh > test")
os.umask(previous_mask)
The above code doesn’t display output but opens a new file called test
in the terminal. That file shows the record of all the files in the current working directory, such as file size, permissions, file creation date, etc.
Use Cases
- File and Directory Creation: Control default permissions when creating files or directories in Python.
- Security and Permissions: Ensure specific default permissions for new files and directories in various applications.
Notes
- The umask set by
os.umask()
applies to the current process and its child processes but does not persist after the Python script ends. - The umask is applied when a file or directory is created using functions like
open()
oros.mkdir()
. - The umask value might vary based on the operating system and the user’s environment.
Conclusion
The os.umask()
method in Python offers control over default file and directory permissions by setting or retrieving the umask
value. Understanding and utilizing this method can be beneficial in scenarios where you need to manage security or customize default permissions for newly created files or directories within your Python programs.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn