Python os.times() Method
-
Syntax of the Python
os.times()
Method -
Example 1: Use the
os.times()
Method to Get the Current Global Process Time in Python -
Example 2: Retrieving Basic Timing Information With the
os.times()
Method in Python -
Example 3: Find the User Time of a Process Using the
os.times()
Method in Python -
Example 4: Monitoring CPU Usage in a Loop Using the
os.times()
Method in Python -
Example 5: Measuring the Execution Time of a Function Using the
os.times()
Method in Python - Use Cases
- Conclusion
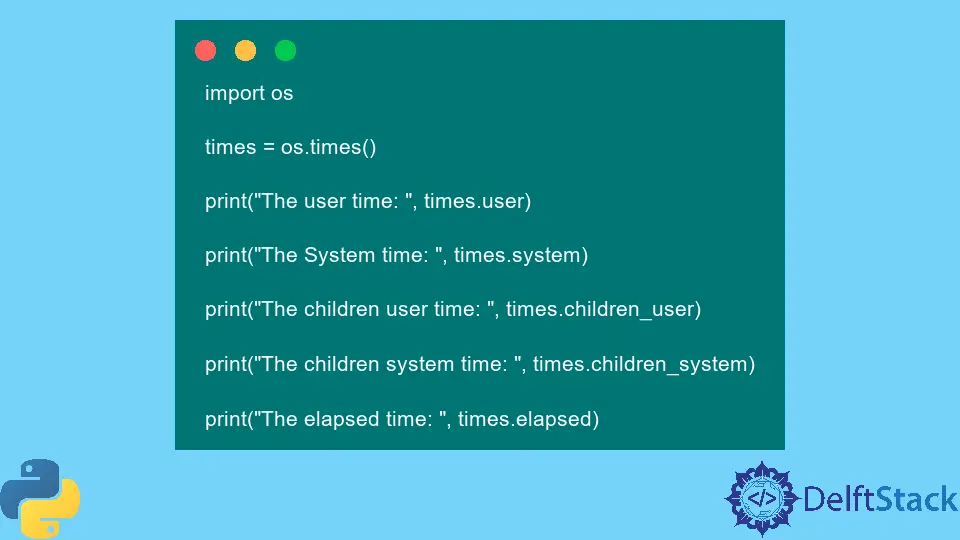
The os.times()
method in Python offers a powerful way to gather timing information about processes and the system as a whole. It provides detailed statistics about CPU usage, execution times, and more.
Python’s os.times()
method is an efficient way of finding the total time of all the current processes of the OS, including all the child processes. In this article, we’ll explore various methods for utilizing os.times()
effectively in Python.
Syntax of the Python os.times()
Method
os.times()
Parameters
This method has no parameters.
Return
os.times()
is part of the os
module in Python. It returns a named tuple containing information about process times. The named tuple includes the following fields:
user
- This attribute represents the total user time.system
- This attribute represents the total system time.children_user
- This attribute represents all the child processes user time.children_system
- This attribute represents all the child processes system time.elapsed
- This attribute represents the real-time that has elapsed since a specified point in the past.
Example 1: Use the os.times()
Method to Get the Current Global Process Time in Python
This example uses the os.times()
method to retrieve timing information about the current process and system.
It then prints out a detailed representation of these timings, including user time, system time, and elapsed time. The specific values will vary depending on the environment and workload.
import os
times = os.times()
print(times)
Output:
posix.times_result(user=1.19, system=0.26, children_user=0.07, children_system=0.01, elapsed=4294768.63)
user
and system
attributes have a known value, and the rest of the attributes have a value of 0
.Example 2: Retrieving Basic Timing Information With the os.times()
Method in Python
The most straightforward use of os.times()
is to retrieve basic timing information about the current process. This includes user time, system time, and more.
import os
times = os.times()
print("The user time: ", times.user)
print("The System time: ", times.system)
print("The children user time: ", times.children_user)
print("The children system time: ", times.children_system)
print("The elapsed time: ", times.elapsed)
Output:
The user time: 3.29
The System time: 0.66
The children user time: 0.07
The children system time: 0.01
The elapsed time: 4295744.82
In this example, we import the os
module to access its functions. Then, we call os.times()
to retrieve the process times information.
Finally, we print out various attributes of the returned named tuple, including user time, system time, children’s user time, children’s system time, and elapsed time.
os.times()
attributes are stored as elements of an array. For example, in the above code, the user time can be retrieved by writing times[0]
and so on.Example 3: Find the User Time of a Process Using the os.times()
Method in Python
This example measures the execution time of a recursive function f(y)
for a specified input y
. The function f(y)
recursively calculates a value based on the input.
The program uses os.times()
to record the process times before and after function execution, allowing it to calculate the user and system times.
import os
# A recursive function to evaluate the time used to execute it
def f(y):
if y <= 1:
return 1
else:
return f(y - 1) + f(y - 2)
num = 35
times1 = os.times()
value = f(num)
print("number=%d, value=%d" % (num, value))
times2 = os.times()
userTime = times2[0] - times1[0]
systemTime = times2[1] - times1[1]
print("User Time=%s, System Time=%s" % (userTime, systemTime))
Output:
number=35, value=14930352
User Time=3.710000000000001, System Time=0.0
The results, including the input value, calculated result, user time, and system time, are printed to the console. The code provides insights into the performance of the recursive function for a given input.
os.times()
for Unix might work differently.Example 4: Monitoring CPU Usage in a Loop Using the os.times()
Method in Python
One practical application of os.times()
is monitoring CPU usage in a loop. This can be useful for tasks such as performance profiling.
import os
import time
# Define the number of iterations
num_iterations = 1000000
# Get the start time
start_time = os.times().elapsed
# Perform a CPU-intensive task
for i in range(num_iterations):
pass
# Get the end time
end_time = os.times().elapsed
# Calculate the CPU time
cpu_time = end_time - start_time
print(f"CPU time for {num_iterations} iterations: {cpu_time}")
In this program, we first import the os
module and the time
module. We define the number of iterations for our CPU-intensive task.
We then use os.times().elapsed
to get the start time. Next, we perform a CPU-intensive task (in this case, a loop that does nothing) for the specified number of iterations.
We get the end time using os.times().elapsed
. Then, we calculate the CPU time by subtracting the start time from the end time.
Finally, we print out the CPU time.
Output:
CPU time for 1000000 iterations: 0.049999999813735485
Example 5: Measuring the Execution Time of a Function Using the os.times()
Method in Python
You can use os.times()
to measure the execution time of a specific function. This is useful for profiling specific parts of your code.
import os
# Define a function to measure
def time_consuming_operation():
for _ in range(1000000):
pass
# Get start time
start_time = os.times().elapsed
# Call the function
time_consuming_operation()
# Get end time
end_time = os.times().elapsed
# Calculate function execution time
execution_time = end_time - start_time
print(f"Execution time: {execution_time}")
In the code example, we define a function named time_consuming_operation()
that performs a CPU-intensive task.
We then use os.times().elapsed
to get the start time. We call the time_consuming_operation()
function, which performs the CPU-intensive task.
Next, we get the end time using os.times().elapsed
. We calculate the execution time by subtracting the start time from the end time.
Lastly, we print out the execution time.
Output:
Execution time: 0.03000000026077032
Use Cases
Understanding the process times of a program can be valuable in various scenarios:
- Performance Profiling:
os.times()
can be used for performance profiling to identify which parts of a program consume the most CPU time. - Resource Management: It allows you to monitor the resource utilization of a program and take actions based on the collected data.
- Benchmarking: When benchmarking code or algorithms,
os.times()
can provide insights into how efficiently the code utilizes system resources.
Conclusion
Mastering the usage of os.times()
in Python provides valuable insights into the timing and resource utilization of your programs. Whether you’re monitoring CPU usage in a loop, measuring the execution time of a specific function, or simply retrieving basic timing information, os.times()
empowers you to make informed decisions about optimizing your code for performance and efficiency.
By incorporating these techniques into your Python programming toolkit, you’ll be better equipped to build high-performance applications.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn