Python os.supports_follow_symlinks Object
- Understanding Symbolic Links
-
Syntax of Python
os.supports_follow_symlinks
Object -
Example 1: Use the
os.supports_follow_symlinks
Object in Python -
Example 2: Use the
os.supports_follow_symlinks
Object to Check if a Method Allowsfollow_symlinks
Parameter - Example 3: Check Support for Following Symbolic Links
- Example 4: Handle File Operations Based on Symbolic Link Support
- Example 5: Conditional Behavior in Opening Files
- Use Cases
- Conclusion
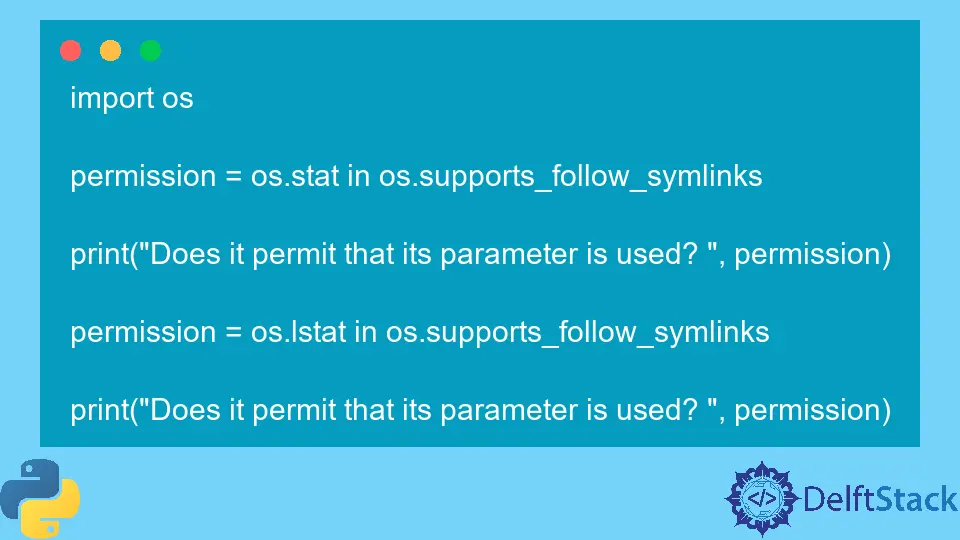
The os.supports_follow_symlinks
attribute in Python is a feature introduced in Python 3.8 that provides information about the platform’s support for symbolic link handling within the os
module. It indicates whether the operating system and its file system support following symbolic links when performing various file-related operations.
Python os.supports_follow_symlinks
object is an efficient way of checking whether a specific OS module allows a method to use its follow_symlinks
parameter or not. Many platforms provide various functionality, so we need to check if a function is available for use in Python.
Understanding Symbolic Links
Symbolic links, often referred to as symlinks or soft links, are pointers to other files or directories in a filesystem. They act as references or shortcuts to access files and directories.
When a program or user interacts with a symbolic link, the operating system can either resolve it to the target file or directory it points to or treat it as a distinct entity.
Purpose of os.supports_follow_symlinks
The os.supports_follow_symlinks
attribute is used to check the platform’s capability to follow symbolic links during file system operations.
It helps in determining whether the operating system supports handling symbolic links transparently or whether it treats symbolic links as distinct entities during file operations like copying, moving, and opening files.
Syntax of Python os.supports_follow_symlinks
Object
os.supports_follow_symlinks
Parameters
It is a non-callable object, so no parameter is required.
Return Value
The return type of this method is a set object representing all the methods in the OS module that allows the use of their follow_symlinks
argument.
True
: Indicates that the operating system and its file system support following symbolic links when performing file operations. The file system operations treat symbolic links as references to their target files or directories.False
: Denotes that the operating system and its file system do not support following symbolic links transparently. File system operations treat symbolic links as distinct entities instead of resolving them to their target files or directories.
Example 1: Use the os.supports_follow_symlinks
Object in Python
This example attempts to retrieve the value of os.supports_follow_symlinks
and assigns it to the variable List
. It displays information about methods or functions that accept a follow_symlinks
parameter based on the value of os.supports_follow_symlinks
.
import os
List = os.supports_follow_symlinks
print(
"Following are the method that allows their follow_symlinks parameter to be used: ",
List,
)
Output:
Following are the method that allows their follow_symlinks parameter to be used: {<built-in function link>, <built-in function access>, <built-in function chown>, <built-in function utime>, <built-in function stat>}
Note that to determine whether any Python method permits using its follow_symlinks
parameter, we can use the in
operator on the supports_follow_symlinks
set.
Example 2: Use the os.supports_follow_symlinks
Object to Check if a Method Allows follow_symlinks
Parameter
This example aims to check whether certain functions (os.stat
and os.lstat
) are present within os.supports_follow_symlinks
.
os.stat
: It’s a function used to get information about a file. The code checks ifos.stat
allows thefollow_symlinks
parameter.os.lstat
: Similar toos.stat
,os.lstat
gets file information, but it specifically handles symbolic links by returning information about the link itself, not the linked file. The code checks ifos.lstat
allows thefollow_symlinks
parameter.
import os
permission = os.stat in os.supports_follow_symlinks
print("Does it permit its parameter to be used? ", permission)
permission = os.lstat in os.supports_follow_symlinks
print("Does it permit its parameter to be used? ", permission)
Output:
Does it permit its parameter to be used? True
Does it permit its parameter to be used? False
In the above code, we are checking whether the os.stat()
and os.lstat
methods allow their follow_symlinks
parameters to be used on local platforms or not.
Example 3: Check Support for Following Symbolic Links
This example demonstrates a simple check to determine if the platform supports following symbolic links.
import os
if os.supports_follow_symlinks:
print("This platform supports following symbolic links.")
else:
print("This platform does not support following symbolic links.")
Output:
This platform supports following symbolic links.
In this code example, os.supports_follow_symlinks
is accessed to check if the platform supports following symbolic links. The code uses a conditional statement to print a message based on the result.
Example 4: Handle File Operations Based on Symbolic Link Support
Here, we’ll illustrate how to handle file operations based on whether the platform supports following symbolic links.
import os
import shutil
source_file = "./source.txt"
target_file = "./target.txt"
# Check if the platform supports following symbolic links
if os.supports_follow_symlinks:
# Create a symbolic link if supported
os.symlink(source_file, target_file)
print(f"Symbolic link created: {target_file}")
else:
# Copy the file if symbolic links are not followed
shutil.copyfile(source_file, target_file)
print(f"File copied: {source_file} -> {target_file}")
Output:
Symbolic link created: ./target.txt
In this example, the code first checks if the platform supports following symbolic links using os.supports_follow_symlinks
.
If supported, it creates a symbolic link (target_file
) pointing to source_file
. Otherwise, it uses shutil.copyfile()
to copy the content of source_file
to target_file
.
Example 5: Conditional Behavior in Opening Files
This example demonstrates conditional behavior when opening files based on symbolic link support.
import os
file_path = "./data.txt"
# Check if the platform supports following symbolic links
if os.supports_follow_symlinks:
# Open the file directly if symbolic links are followed
with open(file_path, "r") as file:
data = file.read()
print(f"Data read from file: {data}")
else:
# Resolve the symbolic link and open the target file if not followed
resolved_path = os.path.realpath(file_path)
with open(resolved_path, "r") as file:
data = file.read()
print(f"Data read from resolved file: {data}")
Output:
Data read from file: Conditional Behavior in Opening Files
In the provided example, it first checks if the platform supports following symbolic links using os.supports_follow_symlinks
.
If supported, it directly opens and reads file_path
. Otherwise, it resolves the symbolic link to its target file using os.path.realpath()
and opens the resolved file.
Use Cases
- File Operations: Knowing whether symbolic links are followed can be crucial when performing file operations like copying, moving, or opening files. Depending on the support for following symlinks, the behavior of these operations can vary.
- Cross-Platform Compatibility: It helps in writing code that behaves consistently across different platforms by handling symbolic links according to the platform’s capabilities.
Considerations
- Platform-Specific Behavior: The support for following symbolic links can vary across operating systems and file systems. It’s important to account for platform-specific behavior when handling symbolic links.
- Python Version: This attribute is available in Python 3.8 and later versions. Code using this attribute should ensure compatibility with the specific Python version.
Conclusion
os.supports_follow_symlinks
provides valuable information about the platform’s capability to handle symbolic links during file system operations. It aids in writing platform-aware code that ensures consistent behavior when dealing with symbolic links, enhancing the reliability and portability of Python applications across various environments.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn