Python os.strerror() Method
-
Understanding
os.strerror()
Method in Python -
Syntax of Python
os.strerror()
Method -
Example 1: Retrieving Error Message for Specific Error Codes Using the
os.strerror()
Method in Python -
Example 2: Use the
os.strerror()
Method to Print First 20 Errors -
Example 3: Use the
os.strerror()
Method to Check for an Invalid Error Code - Example 4: Handling Errors Within Exception Handling Blocks
-
Example 5: Using
os.strerror()
With Custom Error Codes - Conclusion
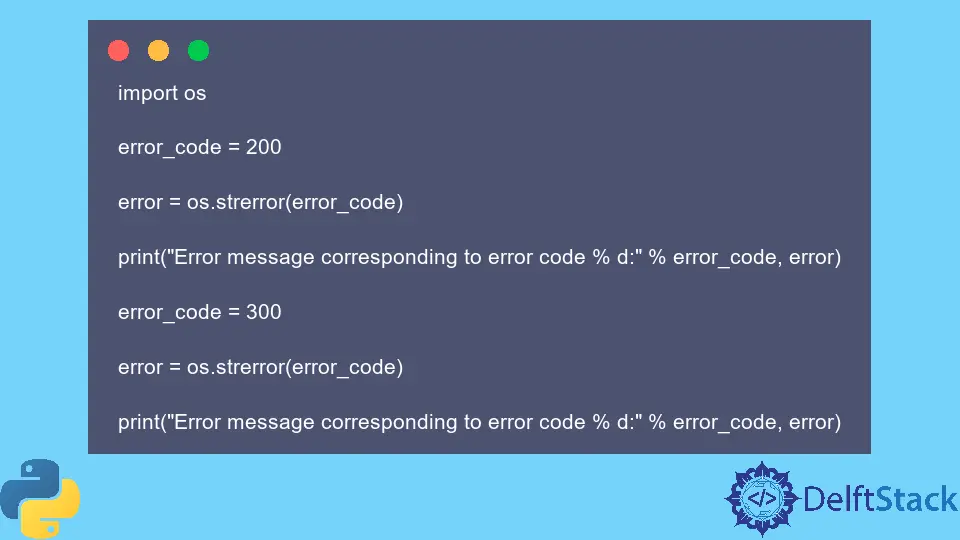
Python’s os
module offers a range of functionalities for interacting with the operating system. Among these, the os.strerror()
method stands out as a powerful tool for obtaining system-specific error messages corresponding to error codes.
This method provides insights into the nature of errors encountered during system-level operations.
Python’s os.strerror()
method is an efficient way of getting an error message corresponding to the input error code. An OSError
exception is raised when an invalid or inaccessible file path is accessed or the specific operating system does not accept the arguments.
Understanding os.strerror()
Method in Python
The os.strerror()
method retrieves the system-specific error message corresponding to a given error code. It aids in understanding errors encountered during various file operations, system calls, and interactions with the operating system.
Syntax of Python os.strerror()
Method
import os
error_message = os.strerror(error_code)
Parameters
Here, error_code
represents the error code for which the corresponding error message is required, and error_message
holds the retrieved error message.
Return Value
The return type of this method is a string representing an error message corresponding to the input error code.
Example 1: Retrieving Error Message for Specific Error Codes Using the os.strerror()
Method in Python
This method is useful when an error code is shown as output, and you need to figure out what the error is related to.
This example showcases the usage of os.strerror()
in Python to retrieve error messages corresponding to specific error codes.
import os
error_code = 1
error = os.strerror(error_code)
print(
"The error message corresponding to the input error code % d is:" % error_code,
error,
)
error_code = 5
error = os.strerror(error_code)
print(
"The error message corresponding to the input error code % d is:" % error_code,
error,
)
Output:
The error message corresponding to the input error code 1 is: Operation not permitted
The error message corresponding to the input error code 5 is: Input/output error
This code essentially demonstrates how os.strerror()
can retrieve and display error messages for specified error codes. It sets specific error codes, retrieves their corresponding error messages using os.strerror()
, and prints out the error messages along with their respective error codes.
Example 2: Use the os.strerror()
Method to Print First 20 Errors
This example demonstrates the usage of the os.strerror()
method to retrieve error messages corresponding to error codes within a specific range. The range of valid error codes might depend on the OS being used.
import os
error_num = 20
for i in range(1, error_num + 1):
error = os.strerror(i)
print("The error message corresponding to the input error code % d is:" % i, error)
Output:
This code snippet effectively loops through a range of error codes, using os.strerror()
to fetch the corresponding error messages for each code and display them in the console. It’s a simple and concise way to demonstrate how os.strerror()
can be utilized to retrieve system-specific error messages for various error codes within a specified range.
Example 3: Use the os.strerror()
Method to Check for an Invalid Error Code
This example utilizes the os.strerror()
method in Python’s os
module to retrieve error messages corresponding to specific error codes. On some platforms, strerror()
might return NULL
when we input an unknown error code.
import os
error_code = 200
error = os.strerror(error_code)
print("Error message corresponding to error code % d:" % error_code, error)
error_code = 300
error = os.strerror(error_code)
print("Error message corresponding to error code % d:" % error_code, error)
Output:
Error message corresponding to error code 200: Unknown error 200
Error message corresponding to error code 300: Unknown error 300
In summary, this code demonstrates the usage of os.strerror()
to fetch error messages based on specified error codes (200
and 300
in this case). It dynamically retrieves and displays the system-specific error messages related to the provided error codes, aiding in understanding and handling errors encountered during system-level operations.
Example 4: Handling Errors Within Exception Handling Blocks
os.strerror()
can be beneficial within exception handling blocks to provide detailed error messages.
Example:
import os
file_path = "nonexistent_file.txt"
try:
with open(file_path, "r") as file:
content = file.read()
except FileNotFoundError as e:
error_code = e.errno
error_message = os.strerror(error_code)
print(f"Error: {error_message}")
Output:
Error: No such file or directory
In this example, an attempt is made to open a file that doesn’t exist. Upon encountering a FileNotFoundError
, os.strerror()
retrieves the error message corresponding to the error code, providing a more informative output about the specific error.
Example 5: Using os.strerror()
With Custom Error Codes
You can utilize os.strerror()
with custom error codes, enhancing error handling and reporting in your applications.
Example:
import os
custom_error_code = 1001
custom_error_message = os.strerror(custom_error_code)
print(f"Custom Error code {custom_error_code}: {custom_error_message}")
Output:
Custom Error code 1001: Unknown error 1001
This code demonstrates how os.strerror()
can retrieve error messages for custom error codes defined within an application, aiding in custom error handling and reporting.
Conclusion
The os.strerror()
method in Python’s os
module is a valuable resource for retrieving system-specific error messages corresponding to error codes. Whether for handling standard error codes or custom errors, its versatility aids in understanding and reporting errors encountered during system-level operations.
By employing os.strerror()
effectively, developers can enhance error handling and debugging within their Python applications, gaining deeper insights into system errors and facilitating more informative error messages.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn