Python os.startfile Method
-
Syntax of
os.startfile()
Method: - Parameter(s)
- Returns
-
Example Code: Use of the
os.startfile()
Method -
Example Code:
FileNotFoundError
Exception in theos.startfile()
Method -
Example Code: Operation Parameter in the
os.startfile()
Method -
Example Code: Open URLs With the
os.startfile()
Method -
Example Code:
os.startfile()
Method for Windows OS &os.open()
Method for Linux OS
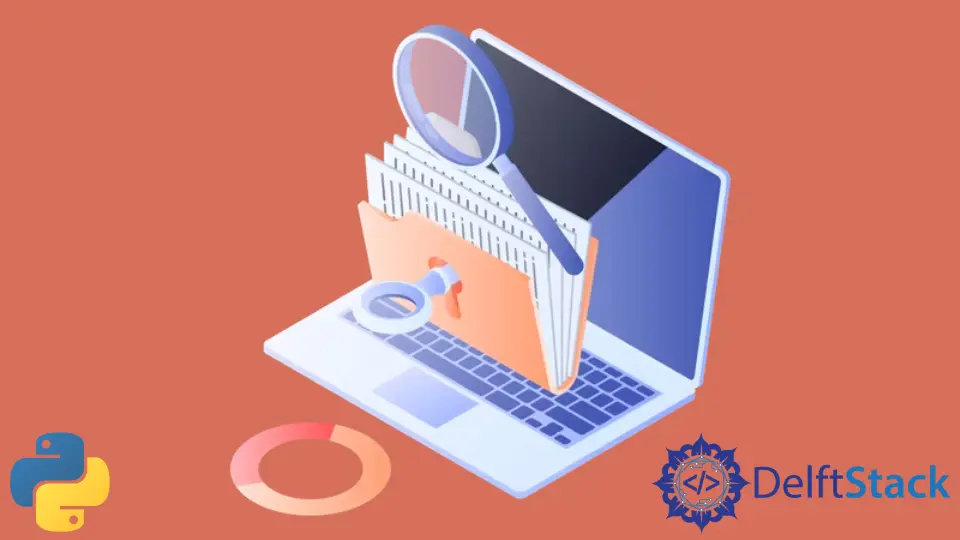
In python, we have the os
module to communicate with the operating system. This os
module provides us with a method known as startfile()
, which takes the file name as a string argument and opens that file.
The startfile()
method returns the exception if the system finds no file. To further elaborate the startfile()
method, let’s check its syntax, parameters, return type and source code below.
Syntax of os.startfile()
Method:
os.startfile(path, operation, arguments, cwd, show_cmd)
Parameter(s)
path |
Compulsory parameter, which is a directory or a file path as a string type |
operation |
This is an optional parameter, which has a fixed string command used to perform on file and directory |
arguments |
Optional parameter, pass as a single string. No effect on the method when using to work with document |
cwd |
Optional parameter, where default working directory is overridden by this argument, which requires an absolute path |
show_cmd |
Optional parameter, to override the window style by default |
Returns
This method doesn’t return anything. Instead, it opens the file or a directory, which is passed as a parameter to this method.
Example Code: Use of the os.startfile()
Method
In python, we use the method os.startfile()
to start or open the file. To use this method, we pass a parameter to the method in string data type, which shows a valid path
of a file or a directory.
In case the path
is given invalid, it shows FileNotFoundError
exception. The method os.startfile()
also opens different file extensions for example; .txt
files, .pdf
files, image file types (like .jpg
/.png
), .doc
(word) file.
Let’s see the example and source code below on using the os.startfile()
method and different file extensions.
# use the os module to use the operating system method associated with it
import os
# this method opens the txt file
os.startfile("C:/Users/lenovo/Downloads/dictionary.txt")
# opens the pdf file
os.startfile("C:/Users/lenovo/Downloads/Firebase+article.pdf")
# opens the word file
os.startfile("C:/Users/lenovo/Downloads/requirement.docx")
# opens the jpg file
os.startfile("C:/Users/lenovo/Downloads/R.jpg")
print("All Files opened successfully.")
# opens the directory
os.startfile("C:/Users/lenovo/Downloads/")
print("I opened Directory as well")
Output:
# console shows the respective output if there is no exception.
All Files opened successfully.
I opened Directory as well
Example Code: FileNotFoundError
Exception in the os.startfile()
Method
In method os.startfile()
takes the string parameter that highlights the path
of a directory or a file. So, if you provide the parameter that is not valid or not found, then the method will return the FileNotFoundError
exception.
This exception occurs on an invalid or unavailable file or a directory. Now, see the below source code on this exception and how we handle the situation.
import os
# handling exception by the use of try & except
try:
# start an invalid directory with startfile() method
os.startfile("C:/Users/lenovo/Downlo/")
# handling the FileNotFoundError exception
except FileNotFoundError as not_found:
print("The directory was not found and handled exceptions successfully.")
# when an exception is not handled
os.startfile("C:/Users/lenovo/Downloads/Dicts.txt")
Output:
# output where file handling is implemented
The directory was not found and handled exceptions successfully.
# file handling is not implemented
FileNotFoundError: [WinError 2] The system cannot find the file specified: 'C:/Users/lenovo/Downloads/Dicts.txt'
Example Code: Operation Parameter in the os.startfile()
Method
In python, with the os.startfile()
method, when we use a single parameter, it opens a file or a directory without doing any particular operation.
With os.startfile()
method, we have an additional and optional parameter which is an operation
parameter. This parameter specifies what we should do with the os.startfile()
method.
The parameter has different operation commands with the file and directory. For example, with a file, we use print
and edit
operation parameters, and with directory, we use explore
and find
operation parameters.
File Operation Parameter
print
–> use the specified file for a printedit
–> open the specified file for an edit
Directory Operation Parameter
explore
–> open the specified path directoryfind
–> provide the search bar on the specified directory for file search
The source code below demonstrates the method os.startfile()
with the second optional parameter.
import os
# file operations print and edit on startfile() method
os.startfile("C:/Users/lenovo/Downloads/dictionary.txt", "print")
os.startfile("C:/Users/lenovo/Downloads/dictionary.txt", "edit")
# directory operations explore and find on startfile() method
os.startfile("C:/Users/lenovo/Downloads/", "explore")
os.startfile("C:/Users/lenovo/Downloads/", "find")
# print the statement, if no exception
print("All operations are done successfully.")
Output:
# output, when there is no exception, occurs in the above source code
All operations are done successfully.
Example Code: Open URLs With the os.startfile()
Method
In addition to the previous examples, we use the method os.startfile()
to open or start a URL from the browser. As we know, this method takes the parameter string as a path or a directory.
In the below example, we send the URL parameter in string type to the os.startfile()
method. The method does not check the validity of the URL (string), instead opens the browser and browses the provided URL.
If the URL is valid, it opens the website; otherwise, the browser shows a This site cannot be reached
message. See the below example on how we can browse or start a URL from the os.startfile
method.
import os
# module to display web-based interface to users
import webbrowser
# takes two parameters, i.e. URL and html
def open_result(outfile, target):
# check if the target parameter is html and OS is Windows
if target == "html":
webbrowser.open_new_tab(outfile)
elif sys.platform == "win32":
os.startfile(outfile)
# check for the mac operating system
elif "mac" in sys.platform or "darwin" in sys.platform:
os.system("open %s" % outfile)
print(outfile)
elif "posix" in sys.platform or "linux" in sys.platform:
os.system("xdg-open %s" % outfile)
# calling the user-defined function here
open_result("www.google.com", "html")
print("Provided URL opens on the browser successfully.")
Output:
# browser opens and shows the browsed URL after displaying the result on the console
Provided URL opens on the browser successfully.
Example Code: os.startfile()
Method for Windows OS & os.open()
Method for Linux OS
The method os.startfile()
has support for Windows operating system only. If we need a similar method in the Linux operating system, we use another similar method to the os.startfile()
, the os.open()
method.
The syntax of this method is os.open(filename, flag)
where the name file is in string data type and flag contains contents like O_RDONLY
and O_WRONLY
.
These constants are available in both Windows and Linux operating systems. This method also returns a file object for reading and writing methods. To use this method, see the below example with source code implementation.
import os
import sys
# make an fd to pass to write() method as a parameter
file_descriptor = os.open("myfile.txt", os.O_CREAT | os.O_RDWR)
# writing the byte code into the file
os.write(file_descriptor, b"Write here anything")
# closing the file
os.close(file_descriptor)
print("The file is closed successfully!!")
Output:
# output when the method shows support for Windows and Linux OS
The file is closed successfully!!
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn