Python os.removedirs() Method
-
Syntax of the
os.removedirs()
Method -
Example Codes: Working With the
os.removedirs()
Method in Python -
Example Codes: Handle Errors While Using the
os.removedirs()
Method in Python
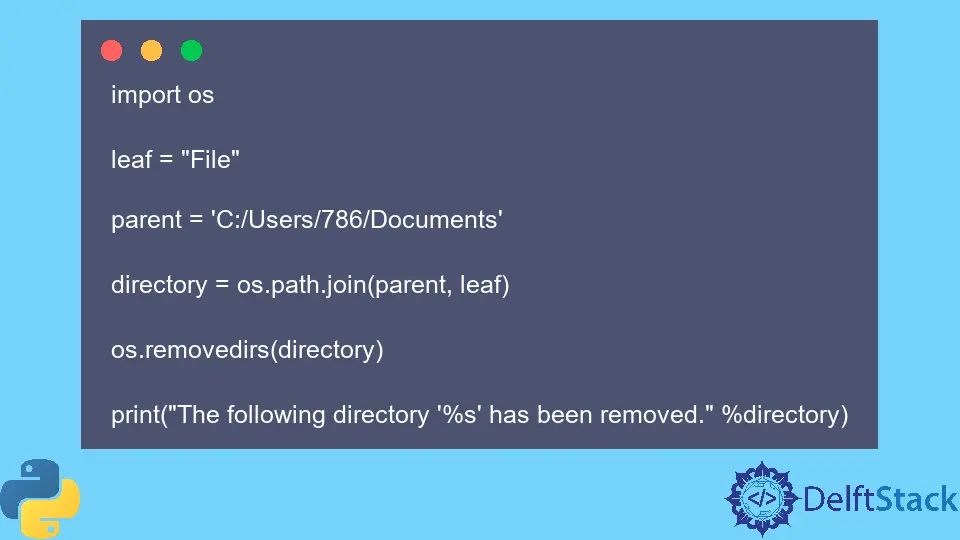
Python os.removedirs()
method is an efficient way of removing empty directories recursively. If the leaf directory is removed successfully, then the os.removedirs()
method tries to remove every parent directory in the path until an error occurs.
Syntax of the os.removedirs()
Method
os.removedirs(path address)
Parameters
path address |
It is an address object of a file system path or a symlink. The object can either be an str or bytes. |
Return
In the execution process, this method does not return any value.
Example Codes: Working With the os.removedirs()
Method in Python
import os
leaf = "File"
parent = "C:/Users/786/Documents"
directory = os.path.join(parent, leaf)
os.removedirs(directory)
print("The following directory '%s' has been removed." % directory)
Output:
The following directory 'C:/Users/786/Documents\File' has been removed.
The os.removedirs()
method will work only if the directory to be deleted is empty.
Example Codes: Handle Errors While Using the os.removedirs()
Method in Python
import os
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
path_address = "/home/File.txt"
try:
os.removedirs(path_address)
print("The directory has been removed successfully")
except NotADirectoryError:
print("The symlink is not a directory.")
except PermissionError:
print("The permission has been denied.")
except OSError as error:
print(error)
print("The directory can not be removed.")
Output:
The symlink is not a directory.
A few errors might occur while using this method. The above error appears most often, and it is due to an invalid or non-existing directory.
If you don’t have the required access to remove a directory, then a PermissionError
is thrown.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn