Python os.putenv() Method
-
Syntax of Python
os.putenv()
Method -
Example 1: Use the
os.putenv()
Method in Python -
Example 2: Understanding the
os.putenv()
Method in Python -
Example 3: Practical Example of the
os.putenv()
Method in Python -
Encountering Error in Using the
os.putenv()
Method in Python - Conclusion
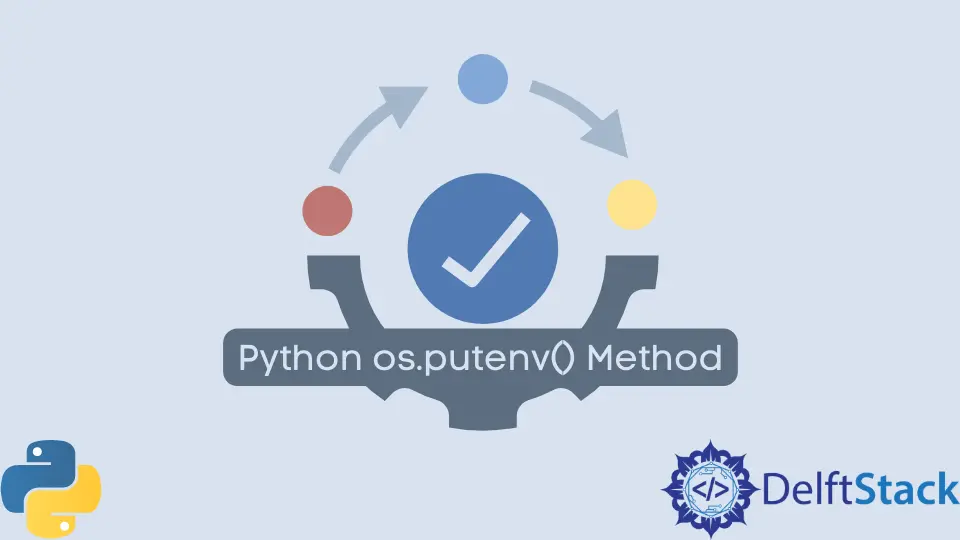
The os.putenv()
function is a powerful tool that allows developers to set or update environment variables within their Python scripts. Python’s os.putenv()
method is an efficient way of changing the environment variable and its subprocesses.
All the assignments to items in os.environ
are directly translated into corresponding calls to os.putenv()
.
Let’s explore how to use os.putenv()
effectively to enhance the flexibility and portability of our applications.
Syntax of Python os.putenv()
Method
os.putenv(key, value)
Parameters
key |
It is the environment variable to be set. |
value |
The string value to which the environment variable is translated. |
Return
There is no return type for this method.
Example 1: Use the os.putenv()
Method in Python
The code snippet below showcases the basic usage of the os.putenv()
function in Python. It sets a new environment variable named key
with the value value
.
Example code:
import os
os.putenv("key", "value")
print("Environment variable set successfully.")
First, the os
module, which provides access to various operating system functionalities in Python, is imported.
The os.putenv()
function is then utilized to set a new environment variable named key
with the corresponding value value
. This function takes two arguments: the name of the environment variable (key
in this case) and its value (value
in this case).
Finally, a confirmation message is printed out using print("Environment variable set successfully.")
, indicating that the environment variable has been successfully set.
Output:
Environment variable set successfully.
Note that the changes made to the environment affect subprocesses, including the following: os.system()
, popen()
, and execv()
.
The changes made to environment variables in a Python script using os.putenv()
may not persist beyond the lifetime of the script. This means that while the script is running, the environment variable will be set, but once the script terminates, the variable may not remain set.
Example 2: Understanding the os.putenv()
Method in Python
The code snippet below showcases the usage of the os.putenv()
function to set the PATH
environment variable to a specific directory on a Windows system.
Example code:
import os
os.putenv("PATH", "C:\\WINNT\\system32")
print("Environment variable set successfully.")
The code snippet begins by importing the os
module, which provides functions for interacting with the operating system.
The os.putenv()
function is then employed to set the PATH
environment variable. In this example, we’re setting it to "C:\\WINNT\\system32"
. This directory contains critical system executables on Windows systems.
Lastly, a confirmation message is printed using print("Environment variable set successfully.")
to indicate that the environment variable has been successfully set.
Output:
Environment variable set successfully.
On some platforms, like FreeBSD
and macOS
, setting the environment using the os.putenv()
may cause memory leaks.
Example 3: Practical Example of the os.putenv()
Method in Python
In this example, let’s consider a more practical example where we set multiple environment variables using os.putenv()
.
The code below will simulate setting up environment variables for a hypothetical Python web application.
Example code:
import os
# Define environment variables for a Python web application
env_variables = {
"DATABASE_URL": "mysql://user:password@localhost/mydatabase",
"SECRET_KEY": "my_secret_key",
"DEBUG": "True",
"ALLOWED_HOSTS": "localhost,127.0.0.1",
}
# Set each environment variable using os.putenv()
for key, value in env_variables.items():
os.putenv(key, value)
# Confirm successful setting of environment variables
print("Environment variables set successfully.")
The code snippet started by importing the os
module, which provides functions for interacting with the operating system.
Next, it defines a dictionary env_variables
containing key-value pairs representing various environment variables required for Python web applications. These variables include:
DATABASE_URL
: The connection string for our MySQL database.SECRET_KEY
: A secret key used for session encryption.DEBUG
: A boolean indicating whether debugging is enabled.ALLOWED_HOSTS
: A comma-separated list of allowed hostnames.
Next, the code iterates over the items in the env_variables
dictionary using a for
loop. For each key-value pair, it uses os.putenv()
to set the corresponding environment variable.
Finally, it prints a confirmation message indicating that the environment variables have been successfully set.
Output:
Environment variable set successfully.
Encountering Error in Using the os.putenv()
Method in Python
Unfortunately, os.putenv()
doesn’t raise exceptions for errors like setting an invalid environment variable name or encountering an invalid value. Instead, it simply returns None
if the operation fails, but it does not raise any errors that Python would handle.
In this example, the code will try to trigger an error by attempting to set an environment variable with a name that’s too long.
Example Code:
import os
# Attempt to set an environment variable with a name that's too long
try:
# The maximum length of an environment variable name is system-dependent,
# but typically it's limited to a certain number of characters (e.g., 255)
os.putenv("A" * 1000, "value")
except Exception as e:
print("Error:", e)
In this example, the code is trying to set an environment variable with a name that’s excessively long. Depending on the system’s limit for the length of environment variable names, this could potentially trigger an error.
However, because os.putenv()
doesn’t raise exceptions for such errors, Python won’t handle it and won’t show any error message printed out.
Please note that the behavior may vary depending on the system and the Python interpreter being used. In many cases, the operation will simply fail silently without raising an error.
Conclusion
The os.putenv()
method in Python is a powerful tool for setting or updating environment variables within scripts. While it offers flexibility, it lacks built-in error handling for certain scenarios, like setting an invalid environment variable name or encountering an invalid value.
In our example, attempting to set an excessively long environment variable name, Python doesn’t raise an error, resulting in silent failure.
This highlights the importance of thorough testing and validation when using os.putenv()
, ensuring correct usage and avoiding unexpected behavior.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn