Python os.path.splitdrive() Method
-
Syntax of Python
os.path.splitdrive()
Method -
Example 1: Use the
os.path.splitdrive()
Method in Python -
Example 2: Use the
os.path.splitdrive()
Method on Windows OS -
Example 3: Use the
os.path.splitdrive()
Method on UNIX -
Example 4: Create a Different File Extension Using the
os.path.splitdrive()
Method on Window OS - Usage and Understanding
- Conclusion
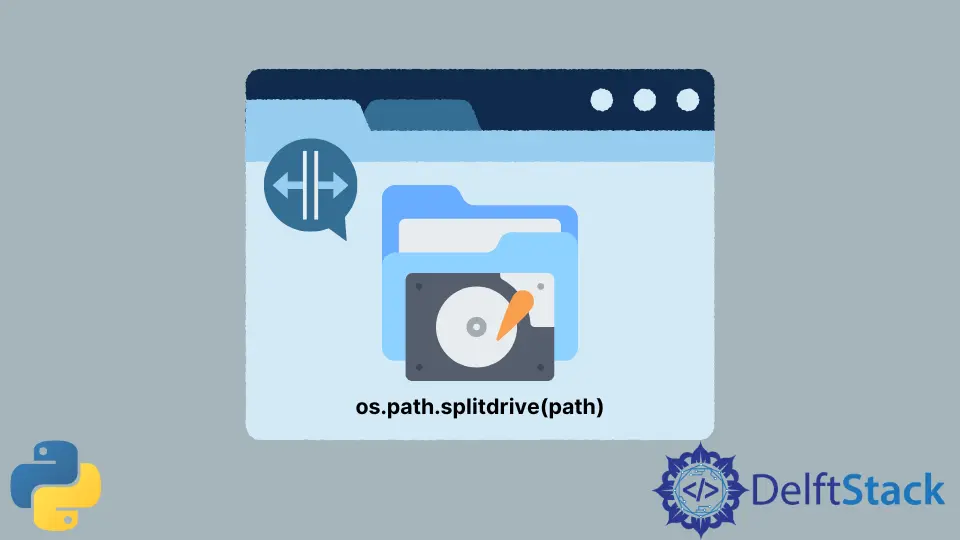
The os.path.splitdrive()
method is a part of the os.path
module in Python and is used for parsing a path into its drive and tail parts. This method is primarily useful for systems that designate a drive letter or volume label for file paths, such as Windows-based systems.
Python os.path.splitdrive()
method is an efficient way to split a path name into a pair drive
and tail
. A drive
is the share point or an empty string; the rest of the path component is the tail
.
Different OS might have a drive
or not, based on their specification.
Syntax of Python os.path.splitdrive()
Method
os.path.splitdrive(path)
Parameters
path |
A valid file path or file name. It’s a string representing the path that needs to be split into drive and tail parts. The object can either be an str or bytes. |
Return Value
The os.path.splitdrive()
method returns a tuple containing the drive part and the tail part of the specified path.
- If the path contains a drive specifier (like
C:\
on Windows), the first element of the tuple will be the drive part, and the second element will be the tail part. - If the path doesn’t contain a drive specifier, the first element of the tuple will be an empty string, and the second element will be the entire path.
Example 1: Use the os.path.splitdrive()
Method in Python
This example employs os.path.splitdrive()
to divide a given path into its drive and tail components. It separates the provided path string into the drive part and the remaining tail.
The print
statements then display the drive and tail components of the path for further analysis or processing. This functionality allows users to extract drive information and the rest of the path for subsequent operations within Python.
import os
path = "home/user/File.txt"
split = os.path.splitdrive(path)
print("The drive of path '%s': " % path, split[0])
print("The tail of path '%s': " % path, split[1])
Output:
The drive of path 'home/user/File.txt':
The tail of path 'home/user/File.txt': home/user/File.txt
As seen in the above code, the first element of the tuple is the drive
, and the subsequent one is the tail
component. In any case, concatenating the drive
and tail
components will always make the original path.
Example 2: Use the os.path.splitdrive()
Method on Windows OS
This example uses os.path.splitdrive()
to break down the provided path into its drive and tail components. Specifically, it analyzes the path "C:/home/user/File.txt"
.
The os.path.splitdrive()
function dissects the path and separates it into two parts: the drive component ("C:"
) and the tail component ("/home/user/File.txt"
).
The code then utilizes print()
statements to display these components separately, aiding in the extraction and inspection of drive-related information and the remaining path details for further processing or analysis.
import os
path = "C:/home/user/File.txt"
split = os.path.splitdrive(path)
print("The drive of path %s: " % path, split[0])
print("The tail of path %s: " % path, split[1])
Output:
The drive of path C:/home/user/File.txt: C:
The tail of path C:/home/user/File.txt: /home/user/File.txt
Note that the Windows OS uses drive specification, so the drive
component of the tuple is never empty.
Example 3: Use the os.path.splitdrive()
Method on UNIX
This example utilizes the os.path.splitdrive()
function to separate the provided path "C:/home/user/File.txt"
into its drive and tail components. In this scenario, the path consists of a drive specifier ("C:"
) and a tail ("/home/user/File.txt"
).
The code uses split[0]
to access and display the drive component ("C:"
) of the path and split[1]
to showcase the remaining tail part ("/home/user/File.txt"
).
These print()
statements help in visualizing the division of the path into its constituent parts, offering insight into the hierarchical structure of the file system path.
import os
path = "C:/home/user/File.txt"
split = os.path.splitdrive(path)
print("The drive of path '%s': " % path, split[0])
print("The tail of path '%s': " % path, split[1])
Output:
The drive of path 'C:/home/user/File.txt':
The tail of path 'C:/home/user/File.txt': C:/home/user/File.txt
Note that the UNIX OS does not use drive specifications, so the drive
component of the tuple is always empty.
Example 4: Create a Different File Extension Using the os.path.splitdrive()
Method on Window OS
This example utilizes the os.path.splitdrive()
function to split the provided path "C:/home/user/File.txt"
into its root and ext
components. In this case, the split[0]
represents the root part ("C:"
) of the path, while split[1]
holds the ext
part ("/home/user/File.txt"
).
The subsequent part of the code concatenates the root part obtained from split[0]
with "/Desktop/File.txt"
to create a new full path address, resulting in "C:/Desktop/File.txt"
.
This showcases how you can manipulate path components in Python using the obtained split components to construct new file paths or addresses within the file system.
import os
path = "C:/home/user/File.txt"
split = os.path.splitdrive(path)
print("The root part is: ", split[0])
print("The ext part is: ", split[1])
path_address = split[0] + "/Desktop/File.txt"
print("The full path address is: ", path_address)
Output:
The root part is: C:
The ext part is: /home/user/File.txt
The full path address is: C:/Desktop/File.txt
Using the above code, you can easily have a new file address with a new name.
Usage and Understanding
1. Paths With Drive Specifier
In Windows-based systems, file paths often start with a drive specifier, such as C:\
or D:\
, indicating the specific drive where the file resides. The os.path.splitdrive()
method effectively separates the drive part from the rest of the path.
2. Paths Without Drive Specifier
For paths that don’t include a drive specifier, the method returns an empty string as the drive part and the entire path as the tail part. This behavior ensures compatibility when working with paths that are not tied to a specific drive or when using systems that do not designate drives in their paths.
3. Cross-Platform Usage
While this method is primarily designed for systems using drive specifiers like Windows, it also works on Unix-based systems. On Unix-like platforms where drive specifiers are not used, the method will return an empty string as the drive part for all paths.
4. Handling Parsed Path Parts
The drive part returned by os.path.splitdrive()
can be utilized to perform specific operations based on the drive where the file is located. It’s particularly useful for scenarios where different drives might require different handling or processing.
5. Compatibility and Path Manipulation
Understanding and utilizing os.path.splitdrive()
is essential when working on code that needs to handle paths across different operating systems. It aids in parsing paths correctly and ensures compatibility when dealing with paths involving drive specifiers.
The os.path.splitdrive()
method plays a vital role in parsing file paths and extracting drive information, offering flexibility and compatibility in handling paths across various systems.
Conclusion
This comprehensive guide explores the os.path.splitdrive()
method in Python, showcasing its functionality in parsing paths, extracting drive information, and ensuring cross-platform compatibility in path handling operations. Understanding this method empowers developers to work with file paths more efficiently, catering to diverse operating system environments.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn