Python os.path.sameopenfile() Method
-
Syntax of Python
os.path.sameopenfile()
Method -
Example 1: Open a Single File Using the
os.path.sameopenfile()
Method in Python -
Example 2: Open Two Different Files Using the
os.path.sameopenfile()
Method in Python -
Example 3: Use File Descriptors Obtained From
os.open()
- Example 4: Check if a File Is the Same as Its Duplicate
-
Example 5: Use
os.dup()
to Create File Descriptor Copies - Important Points to Note
- Use Cases
- Conclusion
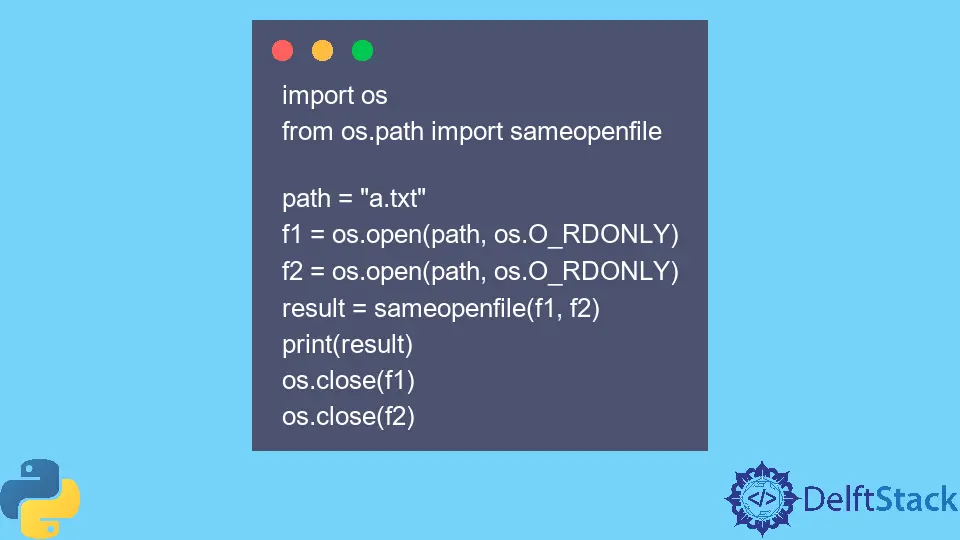
The os.path.sameopenfile()
method is a part of the os.path
module in Python, specifically designed to assist in file operations and path manipulations.
This method is used to determine whether two file descriptor references refer to the same file or not. It checks if the file descriptors fd1
and fd2
are associated with the same file.
The os
and the path
modules provide procedures to interact with the operating system and file system paths, respectively. The sameopenfile()
method checks if two file descriptors refer to the same resource or not.
Under the hood, os.path.sameopenfile()
uses system-specific file identifier mechanisms. It compares the underlying file descriptors of the given files to determine if they point to the same file on the filesystem.
Syntax of Python os.path.sameopenfile()
Method
os.path.sameopenfile(fd1, fd2)
Parameters
Type | Explanation | |
---|---|---|
fd1 |
integer | A file descriptor associated with a valid resource. |
fd2 |
integer | A file descriptor associated with a valid resource. |
Return Value
The sameopenfile()
method returns a Boolean value. It returns True
if both the file descriptors refer to the same resource; otherwise, it returns False
.
Example 1: Open a Single File Using the os.path.sameopenfile()
Method in Python
This example opens the file a.txt
twice, obtaining two different file descriptors (f1
and f2
) using os.open()
.
Then, it uses sameopenfile()
to compare these descriptors to check if they refer to the same file. After the comparison, it prints the result and closes both file descriptors to release the associated system resources.
The output of print(result)
will indicate whether f1
and f2
refer to the same file (True
) or not (False
). If the file descriptors point to the same underlying file, it will return True
; otherwise, it will return False
.
import os
from os.path import sameopenfile
path = "a.txt"
f1 = os.open(path, os.O_RDONLY)
f2 = os.open(path, os.O_RDONLY)
result = sameopenfile(f1, f2)
print(result)
os.close(f1)
os.close(f2)
Output:
True
Since the Python code opened the same file twice concurrently using two file descriptors, the sameopenfile()
method returns True
.
Example 2: Open Two Different Files Using the os.path.sameopenfile()
Method in Python
This example opens two files, file1.txt
and file2.txt
, obtains their respective file descriptors, compares these descriptors using os.path.sameopenfile()
, closes the files, and finally determines whether the two files refer to the same underlying file based on the comparison result.
import os
# Open two files
file1 = open("file1.txt", "r")
file2 = open("file2.txt", "r")
# Get the file descriptors
fd1 = file1.fileno()
fd2 = file2.fileno()
# Check if the files are the same
result = os.path.sameopenfile(fd1, fd2)
# Close the files
file1.close()
file2.close()
# Display the result
if result:
print("The files refer to the same file.")
else:
print("The files do not refer to the same file.")
Output:
The files do not refer to the same file.
Since the Python code is opening two different files, the file descriptors will be different; hence, the sameopenfile()
method returns "The files do not refer to the same file."
.
Example 3: Use File Descriptors Obtained From os.open()
This example demonstrates the usage of os.open()
to open file1.txt
and file2.txt
, obtaining their file descriptors (fd1
and fd2
) directly from the operating system. It then uses os.path.sameopenfile()
to compare these file descriptors and determine if they point to the same file.
import os
# Open two files using os.open()
fd1 = os.open("file1.txt", os.O_RDONLY)
fd2 = os.open("file2.txt", os.O_RDONLY)
# Check if the files are the same
result = os.path.sameopenfile(fd1, fd2)
# Display the result
if result:
print("The files refer to the same file.")
else:
print("The files do not refer to the same file.")
# Close the file descriptors
os.close(fd1)
os.close(fd2)
Output:
The files do not refer to the same file.
Example 4: Check if a File Is the Same as Its Duplicate
In essence, this example opens example.txt
twice to create two file objects (original_file
and duplicate_file
), retrieves their respective file descriptors, checks if they refer to the same file using os.path.sameopenfile()
, prints the comparison result, and finally closes both file objects.
import os
# Open a file
file_path = "example.txt"
original_file = open(file_path, "r")
# Duplicate the file by opening it again
duplicate_file = open(file_path, "r")
# Get file descriptors
fd_original = original_file.fileno()
fd_duplicate = duplicate_file.fileno()
# Check if the files are the same
result = os.path.sameopenfile(fd_original, fd_duplicate)
# Display the result
if result:
print("The original file and its duplicate refer to the same file.")
else:
print("The original file and its duplicate do not refer to the same file.")
# Close the files
original_file.close()
duplicate_file.close()
Output:
The original file and its duplicate refer to the same file.
Example 5: Use os.dup()
to Create File Descriptor Copies
In essence, this example opens data.txt
, duplicates its file descriptor using os.dup()
, compares the original file descriptor with its duplicate using os.path.sameopenfile()
, prints the comparison result, and finally closes both the file and its duplicated file descriptor to release associated resources.
import os
# Open a file
file = open("data.txt", "r")
# Duplicate the file descriptor
fd_copy = os.dup(file.fileno())
# Check if the file and its duplicate are the same
result = os.path.sameopenfile(file.fileno(), fd_copy)
# Display the result
if result:
print("The file and its duplicate file descriptor refer to the same file.")
else:
print("The file and its duplicate file descriptor do not refer to the same file.")
# Close the file and its duplicate
file.close()
os.close(fd_copy)
Output:
The file and its duplicate file descriptor refer to the same file.
Important Points to Note
- File Descriptors:
os.path.sameopenfile()
operates on file descriptors, not file objects directly. You need to obtain the file descriptors using thefileno()
method of file objects. - Platform Dependency: The behavior of this method might vary across different operating systems due to underlying system calls.
- File Closure: It’s important to close the files after performing operations to avoid resource leaks.
Use Cases
- File Handling: When managing multiple file operations, this method helps in determining if two files are referencing the same underlying file.
- System-level Operations: Useful in scenarios where low-level file manipulation and identification are required.
Conclusion
The os.path.sameopenfile()
method is a valuable tool for file management in Python. It aids in checking whether two file descriptors refer to the same file, assisting in various file-related operations and enabling developers to handle file resources more effectively.
Understanding its usage and nuances can significantly benefit when dealing with file operations in Python, ensuring accurate file comparisons and better resource management within your applications.