Python os.path.normpath() Method
-
Syntax of the
os.path.normpath()
Method -
Example 1: Working With the
os.path.normpath()
Method in Python -
Example 2: Use the
os.path.normpath()
Method on Windows OS -
Example 3: Two First Slashes
//
in theos.path.normpath()
Method in Python -
Example 4: Combining Paths in the
os.path.normpath()
Method in Python - Conclusion
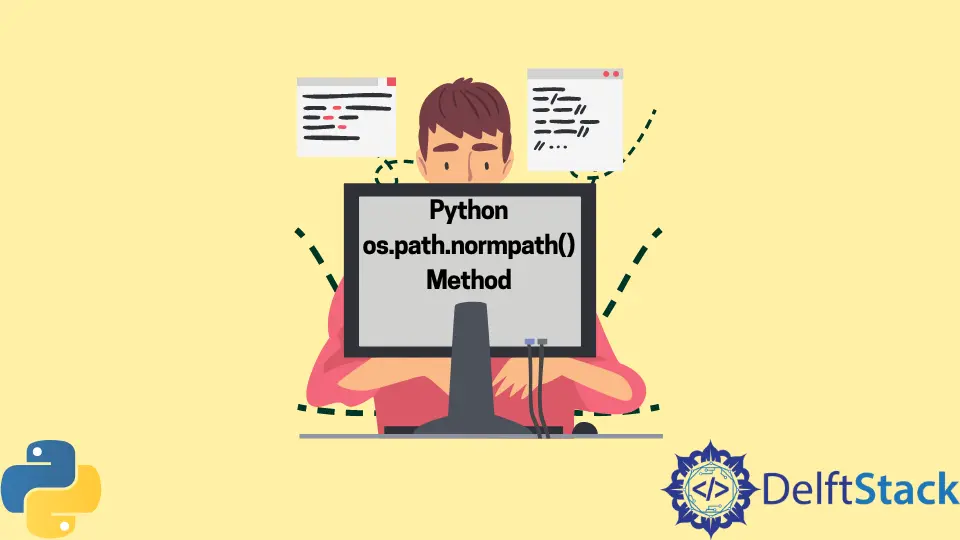
When working with file and directory paths in Python, it’s crucial to ensure that the paths are in a standardized and normalized format to avoid unexpected issues, especially when dealing with different operating systems.
The os.path.normpath()
method comes to the rescue in such scenarios, providing a reliable way to normalize paths.
Python’s os.path.normpath()
method is the most efficient way of normalizing a specified path. Path normalization includes collapsing all redundant separators, such as backslash, period, and up-level references in a path address.
Syntax of the os.path.normpath()
Method
os.path.normpath(path)
Parameters
path |
It is an address object of a file system path or a symlink. The object can either be a string or bytes. |
Return
The return type of this method is a string value. A path string containing the normalized address of a file is returned.
Example 1: Working With the os.path.normpath()
Method in Python
The Python code below demonstrates the usage of the os.path.normpath()
method on different path scenarios.
Let’s break down the code and understand each part.
Example Code:
import os
# Example 1: Path with multiple consecutive slashes
path1 = "/home///user/Documents"
normalized_path1 = os.path.normpath(path1)
print("Original Path 1:", path1)
print("Normalized Path 1:", normalized_path1)
# Example 2: Path with relative current directory reference
path2 = "/home/./Documents"
normalized_path2 = os.path.normpath(path2)
print("\nOriginal Path 2:", path2)
print("Normalized Path 2:", normalized_path2)
# Example 3: Path with relative parent directory reference
path3 = "/home/user/temp/../Documents"
normalized_path3 = os.path.normpath(path3)
print("\nOriginal Path 3:", path3)
print("Normalized Path 3:", normalized_path3)
The code above demonstrates the usage of the os.path.normpath()
method for path normalization.
The os
module is imported to access operating system-dependent functionalities, particularly those related to path manipulation.
The code then presents three distinct examples, each showcasing a different path scenario. In the first example, a path with multiple consecutive slashes is normalized using os.path.normpath()
.
The second example involves a path with a relative current directory reference (./
), and in the third example, a path with a relative parent directory reference (../
) is normalized.
After applying the normalization method in each case, the original and normalized paths are printed, providing a clear view of the transformation.
Output:
The os.path.normpath()
converts A//B
, A/B/
, A/./B
, and A/home/../B
all to A/B
. On Windows, this method converts forward slashes to backward slashes.
The output clearly demonstrates the effectiveness of the os.path.normpath()
method.
It removes redundant slashes, resolves relative path references, and provides standardized paths, making them more reliable for various file and directory operations in a Python program.
Example 2: Use the os.path.normpath()
Method on Windows OS
The os.path.normpath()
method in Python provides a solution by standardizing and simplifying Windows paths.
This code snippet demonstrates the application of os.path.normpath()
to different Windows path scenarios, ensuring a uniform and reliable representation of file paths.
Example Code:
import os
# Example 1: Basic Windows path
path1 = r"C:/Users"
normalized_path1 = os.path.normpath(path1)
print("Original Path 1:", path1)
print("Normalized Path 1:", normalized_path1)
# Example 2: Windows path with a relative current directory reference
path2 = r"C:\Users\.\Documents"
normalized_path2 = os.path.normpath(path2)
print("\nOriginal Path 2:", path2)
print("Normalized Path 2:", normalized_path2)
# Example 3: Windows path with a relative parent directory reference
path3 = r"C:\Users\admin\temp\..\Documents"
normalized_path3 = os.path.normpath(path3)
print("\nOriginal Path 3:", path3)
print("Normalized Path 3:", normalized_path3)
The code begins by importing the os
module, which provides operating system-dependent functionalities, including path manipulation.
The first example (path1
) represents a basic Windows path. The os.path.normpath()
method is applied to normalize the path.
In the second example (path2
), a Windows path includes a relative current directory reference (.\
). The os.path.normpath()
method is used to normalize this path.
The third example (path3
) involves a Windows path with a relative parent directory reference (..\
). The os.path.normpath()
method is employed for normalization.
For each example, the original and normalized paths are printed, providing a visual comparison of the transformations.
Output:
The output demonstrates the effectiveness of the os.path.normpath()
method in handling Windows paths.
It successfully normalizes paths, removing unnecessary components and ensuring a consistent representation.
Example 3: Two First Slashes //
in the os.path.normpath()
Method in Python
The following code snippet demonstrates the usage of os.path.normpath()
on a directory path.
Example Code:
import os
# Example: Directory path with double slashes preserved
directory = os.path.normpath("//user/Home")
# Print the normalized directory path with preserved double slashes
print("Normalized Directory Path:", directory)
The code starts by importing the os
module, which provides operating system-dependent functionalities, including path manipulation.
The directory variable is assigned a path (//user/Home
) that contains unconventional separators. The os.path.normpath()
method is applied to normalize the directory path, resolving the unconventional separators.
Lastly, The normalized directory path is printed to observe the transformation.
Output:
The os.path.normpath()
eliminates all the double slashes, but in this case, it doesn’t remove them because, on Windows, there is a path ambiguity that Python preserves.
The behavior is consistent with the idea that double forward slashes at the beginning (//
) may be treated differently due to network or UNC paths. //user
and /user
are fundamentally different paths, and Python preserves them to avoid unintended changes in the path.
On Windows, these paths may be interpreted as network paths.
Example 4: Combining Paths in the os.path.normpath()
Method in Python
The os.path.normpath()
function, in combination with os.path.join()
, provides an effective solution to achieve standardized paths.
The following code exemplifies this process by combining a base path ("/home/user")
with a relative path ("../documents")
and then normalizing the result.
Example Code:
import os
# Combine paths and normalize
base_path = "/home/user"
relative_path = "../documents"
combined_path = os.path.normpath(os.path.join(base_path, relative_path))
# Print the combined and normalized path
print("Combined and Normalized Path:", combined_path)
The code begins by importing the os
module, offering operating system-dependent functionalities, including path manipulation.
Next, it defines a base path (/home/user
) and a relative path (../documents
) to be combined.
Then, it utilizes os.path.join()
to combine the paths and os.path.normpath()
to normalize the result. This ensures the combined path is in a standardized format.
The combined and normalized path is printed, providing a clear view of the standardized output.
Output:
Conclusion
In conclusion, the os.path.normpath()
method in Python plays a crucial role in ensuring standardized and normalized representations of file and directory paths.
By resolving redundant separators, handling relative path references, and accommodating platform-specific intricacies, this method promotes consistency across different operating systems.
The presented examples illustrate its versatility, whether dealing with Unix-like paths, Windows paths, or scenarios involving double slashes.
Python’s os.path.normpath()
emerges as a reliable tool for robust path manipulation, contributing to the portability and reliability of Python applications across diverse environments.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn