Python os.path.isabs() Method
-
Syntax of Python
os.path.isabs()
Method -
Example 1: Use the
os.path.isabs()
Method to Check Specified Path in Python -
Example 2: Use the
os.path.isabs()
Method Withif
Condition in Python -
Example 3: Use the
os.path.isabs()
Method to Check Valid Directory in Python -
Example 4: Difference Between
isabs()
andis_absolute()
Methods in Python - Usage and Understanding
- Conclusion
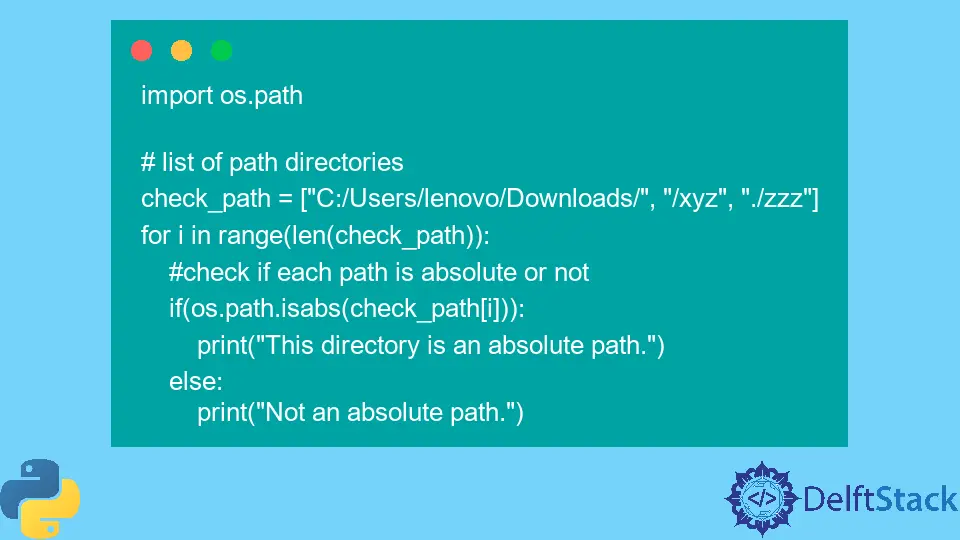
The os.path.isabs()
method is a utility function within Python’s os.path
module, designed to determine whether a given path is an absolute path or a relative path. This function assists in validating and discerning paths based on their absolute or relative nature, aiding in path handling within various file system operations.
The isabs()
method is used to check whether the provided path is an absolute path or not. The absolute path is the one that shows full details of the location of the file or directory.
It shows the starting point, i.e., the root (parent) element to the ending subdirectories.
Syntax of Python os.path.isabs()
Method
os.path.isabs(path)
Parameters
path |
A string representing a file path or directory. It’s the path to be evaluated for its absoluteness or relativity. |
Return Value
The os.path.isabs()
method returns a Boolean value:
True
if the provided path is an absolute path.False
if the provided path is a relative path.
Example 1: Use the os.path.isabs()
Method to Check Specified Path in Python
This example demonstrates the use of os.path.isabs()
to check if a given path is an absolute path or not.
check_path = "C:/Users/lenovo/Downloads/main.py"
: This represents a full path from the drive to the file name. Theos.path.isabs()
method returnsTrue
since it’s an absolute path.check_path = "C:/Users/lenovo/Downloads/"
: Here, the path includes the drive name and a subdirectory. Again,os.path.isabs()
returnsTrue
because it’s still an absolute path.check_path = "/Users/lenovo/Downloads/"
: This path starts with a slash but lacks a drive specifier, making it a relative path.os.path.isabs()
returnsTrue
if the current operating system considers it as absolute (like in Unix-based systems), otherwiseFalse
.check_path = "Users/lenovo/Downloads/"
: This path doesn’t include a drive name or a leading slash, so it’s a relative path. Therefore,os.path.isabs()
returnsFalse
since it’s not an absolute path.
# importing os.path module
import os.path
# Full path starting from drive to file name
check_path = "C:/Users/lenovo/Downloads/main.py"
# Passing the path to the method
check_abs = os.path.isabs(check_path)
print(check_abs)
# Path with drive name - root to subdirectory
check_path = "C:/Users/lenovo/Downloads/"
# Passing the path to the method
check_abs = os.path.isabs(check_path)
print(check_abs)
# Not a full path, includes slash symbol for checking
check_path = "/Users/lenovo/Downloads/"
check_abs = os.path.isabs(check_path)
print(check_abs)
# Again, not a full path, includes no slash symbol or drive name
check_path = "Users/lenovo/Downloads/"
check_abs = os.path.isabs(check_path)
print(check_abs)
Output:
True
True
True
False
The method returns whether the specified path is an absolute path or not. An absolute path can start with the drive name and follow the subdirectories path, or it can start with a slash symbol (/
).
Note that the output depends on the operating system where you run the code because determining an absolute path differs on Unix and Windows.
Example 2: Use the os.path.isabs()
Method With if
Condition in Python
This example employs os.path.isabs()
to evaluate whether a set of given paths is absolute or relative. It uses a for
loop to iterate through a list of paths and checks each one using os.path.isabs()
.
If a path is absolute, it prints "This directory is an absolute path."
; otherwise, it prints "Not an absolute path."
. This code helps distinguish between absolute and relative paths in a given list.
import os.path
# List of path directories
check_path = ["C:/Users/lenovo/Downloads/", "/xyz", "./zzz"]
for i in range(len(check_path)):
# check if each path is absolute or not
if os.path.isabs(check_path[i]):
print("This directory is an absolute path.")
else:
print("Not an absolute path.")
Output:
This directory is an absolute path.
This directory is an absolute path.
Not an absolute path.
We have a list of paths, and each path is checked with the os.path.isabs()
method and accesses different conditions accordingly.
Example 3: Use the os.path.isabs()
Method to Check Valid Directory in Python
This example demonstrates the os.path.isabs()
method in Python, which is used to determine whether a given path is an absolute path or a relative path.
It checks various input path strings and determines their absolute or relative nature based on the presence of a drive specifier or the initial slash symbol. The method returns True
if the path is absolute and False
if it’s a relative path.
import os
# sent a random value as a parameter to this method with a slash symbol
print(os.path.isabs("//\\\\xmls\\hello.txt"))
# no slash symbol
print(os.path.isabs("\\\\xmls\\"))
# value with the drive name
print(os.path.isabs("C://\\\\xmls"))
# value with no drive or symbol
print(os.path.isabs("a/b"))
Output:
True
False
True
False
The method returns True
for the said parameter. The reason is that the os.path.isabs()
method considers the slash (/
) symbol at the start as an absolute path.
The method does not check if it is a valid path or not. So, the returned value may be an absolute but not a valid directory.
Example 4: Difference Between isabs()
and is_absolute()
Methods in Python
This example uses the pathlib
module to check whether specified paths are absolute or not. It creates Path
and PurePath
objects for different paths and then utilizes the is_absolute()
method to determine if each path is absolute.
The method returns True
for absolute paths and False
for relative paths.
# Import pathlib module
import pathlib
# check whether the is_absolute() method on current file path
path = pathlib.Path(__file__)
print(path.is_absolute())
# check a particular location
path = pathlib.PurePath("C:/Users/Downloads/main.py")
print(path.is_absolute())
Output:
True
True
We use the os.path
module to interact with different Python methods, and the PurePath
module also provides different methods to work with. However, it is preferable to work with the PurePath
method as it has more support with Python 3.1+ versions.
The PurePath
performs operations on path strings, and the path accesses the original file system.
Both methods check whether a specified path is absolute, but os.path.isabs()
operates on strings representing paths directly, while pathlib.Path.is_absolute()
requires a Path
object created using pathlib.Path
.
Use os.path.isabs()
for traditional string-based path handling and pathlib.Path.is_absolute()
for working with pathlib
objects in modern Python code.
Usage and Understanding
1. Distinguishing Absolute and Relative Paths
os.path.isabs()
helps in determining whether a path is absolute or relative, which is crucial for path validation and manipulation within file system operations.
2. Absolute Paths
An absolute path refers to the complete path starting from the root directory (/
in Unix-based systems). os.path.isabs()
identifies such paths by returning True
if the provided path starts with the root directory.
3. Relative Paths
Relative paths are defined concerning the current working directory or another referenced directory. os.path.isabs()
identifies relative paths by returning False
if the provided path is not absolute.
4. Platform Independence
os.path.isabs()
handles path identification uniformly across different operating systems, ensuring consistent behavior and results regardless of the underlying platform.
5. Path Validation
When processing user-provided paths or validating file paths within applications, os.path.isabs()
proves helpful in confirming path types before performing operations.
6. Path Handling in File Operations
For filesystem-related tasks, especially while manipulating and working with file paths, distinguishing between absolute and relative paths using os.path.isabs()
aids in accurate path resolution.
7. Input Sanitization
In scenarios where applications accept user input for file paths, verifying whether the provided path is absolute or relative using os.path.isabs()
ensures proper handling and prevents errors due to incorrect path formats.
8. Path Normalization
Before utilizing paths in filesystem operations, it’s beneficial to validate and normalize them. os.path.isabs()
is a starting point in this process, ensuring that paths meet the required criteria for subsequent operations.
The os.path.isabs()
method in Python’s os.path
module serves as a fundamental tool for discerning between absolute and relative paths. By returning a Boolean value based on the nature of the provided path, it enables developers to accurately determine and validate paths before using them in file system operations.
Conclusion
This detailed guide delves into the os.path.isabs()
method in Python, elucidating its role in discerning between absolute and relative paths. By providing a simple Boolean evaluation, this method facilitates accurate path validation, ensuring proper handling of paths in various file system operations.
Understanding and utilizing os.path.isabs()
aid in robust path handling within Python applications, ensuring accuracy and consistency in working with file paths across diverse platforms and scenarios.
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn