Python os.path.getmtime() Method
-
Syntax of
os.path.getmtime()
Method -
Example Code 1: Use the
os.path.getmtime()
Method to Get Modification Time -
Example Code 2: The
os.path.getmtime()
Method ReturnsFileNotFoundError
-
Example Code 3: Difference Between
os.path.getmtime()
andos.path.getctime()
Methods -
Example Code 4: Use the
datetime.now()
Method to Get Time Difference Between Two Timestamps -
Example Code 5: Handle the
os.path.getmtime()
MethodFileNotFoundError
Exception -
Example Code 6: Handle the
os.path.getmtime()
MethodPermissionError
Exception - Conclusion
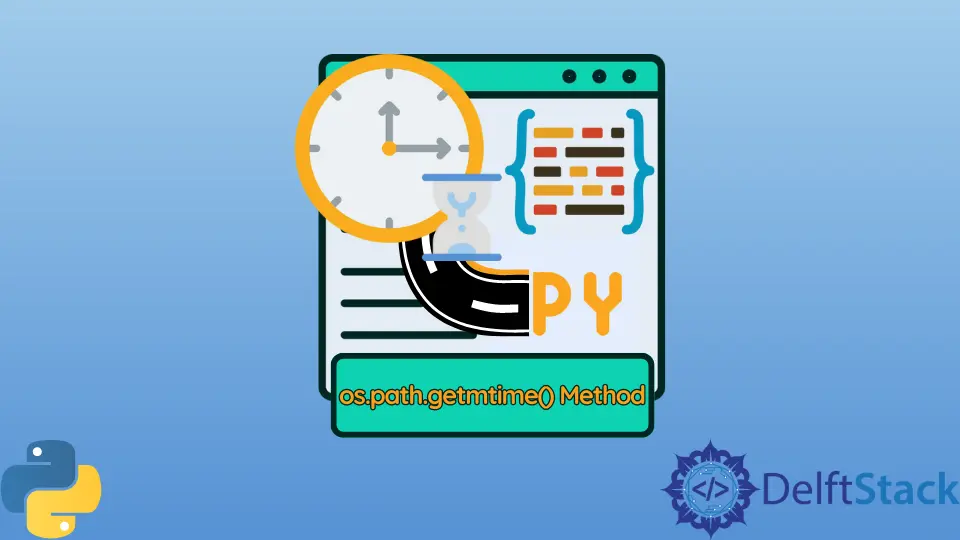
In Python, handling file operations is a common task, whether it’s reading, writing, or simply getting information about files. One crucial aspect of file handling is knowing when a file was last modified.
This information can be vital for various applications, such as monitoring changes in files, syncing data, or implementing version control systems.
One way to obtain the last modification time of a file in Python is by using the os.path.getmtime()
method. This method allows you to retrieve the timestamp representing the time when the specified file was last modified.
The os.path.getmtime()
method returns time (in seconds) for the directory and path of the file. The output time of the modified file is shown in the form of seconds on the console.
Syntax of os.path.getmtime()
Method
os.path.getmtime(path)
Parameters
path |
The specified file or directory path to get the modification time. |
Return
This method returns the seconds as a floating point value of the modified file time. We can change that number to a local time stamp using the ctime()
method.
Example Code 1: Use the os.path.getmtime()
Method to Get Modification Time
This code snippet demonstrates how to utilize the os.path.getmtime()
method in Python to obtain the last modification time of both a directory and a file.
file.txt
:
This is a text file.
Example code:
# Importing required modules
import os
import time
# Path of the directory
# Complete example of path: C:/Users/lenovo/Downloads/
file_path = "/"
# Get the time of last modification in the directory
file_modification_time = os.path.getmtime(file_path)
print("Last modification time of the directory: ", file_modification_time)
# Convert the timestamp to local time format
local_time = time.ctime(file_modification_time)
print("Last modification time in Date Time format: ", local_time)
# Path of the file
# Complete example path of the file: C:/Users/lenovo/Desktop/file.txt
file_path = "file.txt"
# Get the time of last modification of the file
file_modification_time = os.path.getmtime(file_path)
print("Last modification time of the file: ", file_modification_time)
# Convert the timestamp to local time format
local_time = time.ctime(file_modification_time)
print("Last modification time in Date Time format: ", local_time)
The code begins by importing necessary modules, namely os
and time
, essential for file operations and time-related functionalities, respectively.
Subsequently, it specifies the directory path (file_path
) whose last modification time is sought. Utilizing os.path.getmtime()
, it retrieves the last modification time of the specified directory.
Following this, the obtained timestamp is converted to the local time format through time.ctime()
and stored in the local_time
variable. The file path (file_path
) is then updated to target a specific file (myfile.txt
) to retrieve its last modification time using the same method.
Again, the obtained timestamp is converted to the local time format and stored in local_time
.
Output:
The output of the code will display the last modification time of both the directory and the file in two different formats: the timestamp in seconds since the epoch and the corresponding local date and time format.
Example Code 2: The os.path.getmtime()
Method Returns FileNotFoundError
This code snippet demonstrates the usage of os.path.getmtime()
to obtain the last modification time of a file located at "C:/Users/lenovo/Downloads/myfile.txt"
.
file.txt
:
This is a text file.
Example code:
import os
# Path of the file
# Complete example path of the file: C:/Users/lenovo/Desktop/file.txt
file_path = "file.txt"
# Get the last modification time of the file
file_modification_time = os.path.getmtime(file_path)
# Print the last modification time
print("Last modification time of the file: ", file_modification_time)
This code snippet showcases the utilization of the os.path.getmtime()
function in Python to retrieve the last modification time of a specified file. It begins by importing the necessary os
module.
The file path of the target file, file.txt
, is then defined. The os.path.getmtime()
function is called with this file path to obtain the last modification time, which is stored in the file_modification_time
variable.
Finally, the obtained modification time is printed to the console.
Output:
The os.path.getmtime()
method returns an error exception when the specified path does not exist.
FileNotFoundError: The system cannot find the file specified: 'C:/Users/lenovo/Downloads/myfile.txt'
Example Code 3: Difference Between os.path.getmtime()
and os.path.getctime()
Methods
This code demonstrates how to utilize the os.path.getctime()
function to retrieve the creation time of a directory and a file and then convert these timestamps into a human-readable date and time format using the time.ctime()
function.
Additionally, it showcases how file creation time remains constant and never changes.
file.txt
:
This is a text file.
Example code:
import os
import time
# Path of the directory
# Complete example of path: C:/Users/lenovo/Downloads/
file_path = "/"
# Get the creation time of the directory
file_modification_time = os.path.getctime(file_path)
print("Last modification time of the directory: ", file_modification_time)
# Convert the timestamp to local time format
local_time = time.ctime(file_modification_time)
print("Last modification time in Date Time format: ", local_time)
# Path of the file
# Complete example path of the file: C:/Users/lenovo/Desktop/file.txt
file_path = "file.txt"
# Get the creation time of the file
file_modification_time = os.path.getctime(file_path)
print("Last modification time of the file: ", file_modification_time)
# Convert the timestamp to local time format
local_time = time.ctime(file_modification_time)
print("Last modification time in Date Time format: ", local_time)
The code snippet above showcases the usage of os.path.getctime()
to obtain the creation time of both a directory and a file in Python.
After importing the necessary modules os
and time
, it defines the paths for the directory and the file. Then, it calls os.path.getctime()
with each path to retrieve the respective creation times.
These timestamps are then converted into a human-readable format using time.ctime()
, allowing for easy interpretation.
It also highlights that the creation time of a file remains constant and does not change, providing insight into file management tasks and behavior.
Output:
As we have discussed earlier, the os.path.getmtime()
method returns the time of modification of a file or directory, and the os.path.getctime()
returns the creation time of the file or path.
Example Code 4: Use the datetime.now()
Method to Get Time Difference Between Two Timestamps
This example illustrates how to utilize the os.path.getmtime()
function to retrieve the modification time of a specified file and then calculate the time difference between the current time and the file’s modification time.
Additionally, it employs the pytz
library to handle time zones, enabling the conversion of timestamps to datetime
objects with the appropriate timezone information.
file.txt
:
This is a text file.
Example code:
import os
from datetime import datetime
import pytz
# Function to get current date and time in a specified timezone
def getDateTime(timezone):
tz = pytz.timezone(timezone)
dateTime = datetime.now().astimezone()
return dateTime.astimezone(tz)
# Function to convert timestamp to datetime object in a specified timezone
def timestamp(timestamp, timezone):
tz = pytz.timezone(timezone)
dateTime = datetime.fromtimestamp(timestamp).astimezone()
return dateTime.astimezone(tz)
# Specify the desired timezone
time_zone = "Asia/Karachi"
# Get the modification timestamp of the file
# Complete example path of the file: C:/Users/lenovo/Desktop/file.txt
file_timestamp = os.path.getmtime("file.txt")
# Get current date and time
currentDateTime = getDateTime(time_zone)
print("Current time stamp of the device: ", currentDateTime)
# Convert file timestamp to datetime object with specified timezone
file_datetime = timestamp(file_timestamp, time_zone)
print("Creation time of the specified file: ", file_datetime)
# Calculate the time difference between current time and file modification time
time_difference = currentDateTime - file_datetime
print("Difference of time stamp between the above two times: ", time_difference)
This Python code snippet showcases how to retrieve the last modification time of a specified file using os.path.getmtime()
and calculate the time difference between the current time and the file’s modification time.
It starts by defining two functions: getDateTime()
and timestamp()
, which are responsible for obtaining the current date and time in a specified timezone and converting a timestamp to a datetime
object in the same timezone, respectively.
The desired timezone is specified as Asia/Karachi
. The modification timestamp of the file C:/Users/lenovo/Desktop/myfile.txt
is retrieved using os.path.getmtime()
.
Then, the current date and time are obtained and converted to the specified timezone. The file’s modification timestamp is also converted to a datetime
object in the same timezone.
Finally, the time difference between the current time and the file’s modification time is calculated and printed.
Output:
Current time stamp of the device: 2022-08-09 23:10:06.151655+05:00
Creation time of the specified file: 2022-08-09 21:37:11.666461+05:00
Difference of time stamp between the above two times: 1:32:54.485194
The output of the code will display the current timestamp of the device, the creation time of the specified file, and the difference in time stamps between the two times since the methods getDateTime()
and timestamp()
were used.
Example Code 5: Handle the os.path.getmtime()
Method FileNotFoundError
Exception
This code snippet demonstrates how to use os.path.getmtime()
to retrieve the last modification time of a specified file, along with handling exceptions for non-existent paths.
file.txt
:
This is a text file.
Example code:
import os
import sys
import time
# Path of the file
path = "C:/Users/lenovo/Downloads/myfile.txt"
# Try to retrieve the last modification time
try:
file_modification_time = os.path.getmtime(path)
print("Last modification time of the file:", file_modification_time)
except OSError:
# Handle the case where the file does not exist
print("Path '%s' does not exist. Please recheck the path." % path)
# Exit the program if the file does not exist
sys.exit()
# Convert modification time to local time format
time = time.ctime(file_modification_time)
print("Last modification in Local time:", time)
The code snippet above demonstrates how to retrieve the last modification time of a specified file using Python’s os.path.getmtime()
function, along with error handling for non-existent paths.
Initially, the necessary modules os
, sys
, and time
are imported. The file path is defined, and within a try
block, os.path.getmtime()
is used to obtain the last modification time of the file.
If the file does not exist, an OSError
exception is caught, and an error message is printed. The program exits using sys.exit()
to avoid further execution.
Finally, the obtained modification time is converted into a human-readable local time format using time.ctime()
and displayed.
Output:
The output of the code will display the last modification time of the specified file, followed by the same time in a local time format.
Example Code 6: Handle the os.path.getmtime()
Method PermissionError
Exception
Another error that can be encountered when using the os.path.getmtime()
method is the PermissionError
.
This error occurs when the user does not have the necessary permissions to access the file or directory specified in the path.
Example code:
import os
# Example path to a file that requires root permissions
file_path = "/root/private_file.txt"
try:
modification_time = os.path.getmtime(file_path)
print("Last modification time:", modification_time)
except PermissionError:
print("Permission denied to access the file.")
In this example, we attempt to retrieve the modification time of a file located at "/root/private_file.txt"
, which typically requires root permissions to access Unix-like systems.
When os.path.getmtime()
is called with this path without the necessary permissions, it raises a PermissionError
.
To handle this error gracefully, we use a try-except
block to catch the exception and print a helpful error message indicating that permission to access the file is denied.
Output:
Permission denied to access the file.
Conclusion
In conclusion, the os.path.getmtime()
method in Python plays a crucial role in file handling tasks by enabling users to retrieve the last modification time of a specified file or directory.
This information is invaluable for various applications such as monitoring changes, syncing data, or implementing version control systems. Despite its utility, it’s important to handle potential errors gracefully, such as FileNotFoundError
and PermissionError
, which can occur when the specified file or directory does not exist or when the user lacks necessary permissions, respectively.
Python developers can ensure reliability in their applications and streamline file management operations by knowing how to use this method effectively and handle related errors.
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn