Python os.path.getctime() Method
-
Syntax of
os.path.getctime()
Method -
Example 1: Use the
os.path.getctime()
Method in Python -
Example 2: Handling Errors in the
os.path.getctime()
Method -
Example 3: Use the
os.path.getctime()
Method Withif else
Statement - Conclusion
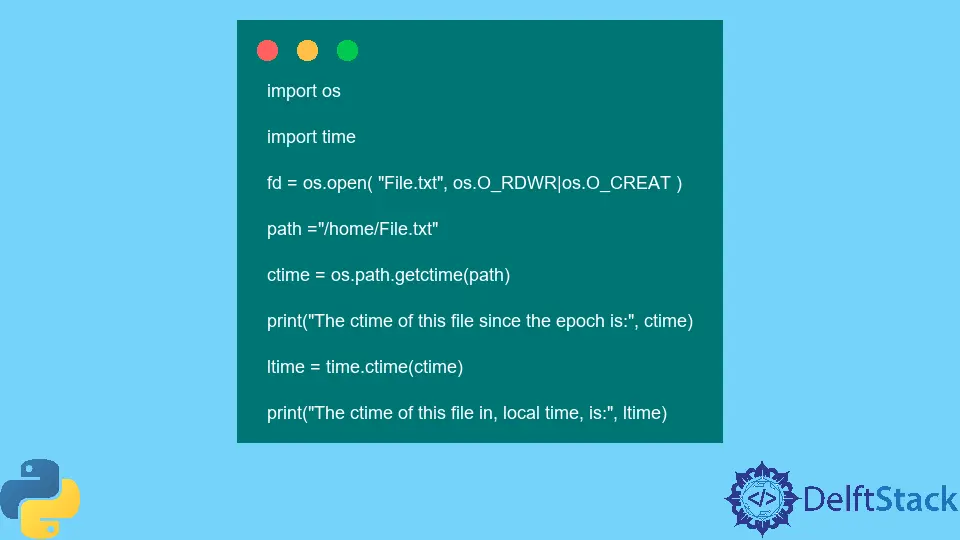
Python os.path.getctime()
method is an efficient way of knowing a file’s ctime
. The term ctime
in UNIX systems means the latest change in the metadata for a specified path.
In Windows, it means the creation time of that file/folder.
Syntax of os.path.getctime()
Method
os.path.getctime(path)
Parameters
path
- An address object of a file system path or a symlink. The object can either be an str
or bytes.
Return
The return type of this method is a floating-point value representing the recent access time of a specified path in seconds.
Example 1: Use the os.path.getctime()
Method in Python
import os
import time
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
path = "/home/File.txt"
ctime = os.path.getctime(path)
print("The ctime of this file since the epoch is:", ctime)
ltime = time.ctime(ctime)
print("The ctime of this file in, local time, is:", ltime)
Output:
The ctime of this file since the epoch is: 1698781786.9729118
The ctime of this file in, local time, is: Wed Nov 1 03:49:46 2023
In this code, we first import the os
and time
modules in Python. We then use the os.open()
method to open the file named "File.txt"
in read and write mode, creating it if it doesn’t exist.
We specify the file path as "/home/File.txt"
. Next, we use the os.path.getctime()
method to retrieve the creation time (ctime
) of the specified file and store it in the variable ctime
.
We print the ctime
of the file since the epoch using the print()
function. Additionally, we use the time.ctime()
function to convert the ctime
into a more human-readable local time format and store it in the variable ltime
.
Finally, we print the ctime
of the file in local time. The output provides information about when the file was created, both in terms of seconds since the epoch and in a more readable local time format.
The epoch
means where the time starts for a particular system and is platform-dependent. For example, in Unix systems, the epoch
is January 1, 1970, 00:00:00 (UTC)
.
Example 2: Handling Errors in the os.path.getctime()
Method
import os
import time
import sys
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
path = "/home/user/File.txt"
try:
c_time = os.path.getctime(path)
print("The ctime of this file since the epoch is:", ctime)
except OSError:
print("The path '%s' does not exist or is inaccessible." % path)
sys.exit()
ltime = time.ctime(ctime)
print("The ctime of this file in, local time, is:", ltime)
Output:
The path '/home/user/File.txt' does not exist or is inaccessible.
In this code, we begin by importing the necessary modules: os
, time
, and sys
. We then use the os.open()
method to open a file named "File.txt"
in read and write mode, creating it if it doesn’t already exist.
The file path is specified as "/home/user/File.txt"
. Inside a try-except
block, we attempt to retrieve the creation time (ctime
) of the file using os.path.getctime()
.
If the operation encounters an OSError
, indicating that the specified file path is inaccessible or does not exist, we print an error message stating the issue and exit the program using sys.exit()
. Assuming the file is accessible, we print the ctime
of the file since the epoch
.
Lastly, we use the time.ctime()
function to convert the ctime
into a more human-readable local time format, storing it in the variable ltime
, and print the ctime
in local time.
But in the above output, the code raises an OSError
exception because the file does not exist. You might have a similar error if the file is inaccessible or the path is invalid.
Example 3: Use the os.path.getctime()
Method With if else
Statement
import os
import time
file_path = "/home/File.txt"
if os.path.exists(file_path):
ctime = os.path.getctime(file_path)
print(f"The ctime of the file since the epoch is: {ctime}")
ltime = time.ctime(ctime)
print(f"The ctime of the file in local time is: {ltime}")
else:
print(f"The file at {file_path} does not exist.")
Output:
The ctime of the file since the epoch is: 1698781786.9729118
The ctime of the file in local time is: Wed Nov 1 03:49:46 2023
In this code, we start by defining the file path as "/home/File.txt"
. We use the os
module to check if the specified file path exists with the os.path.exists()
function.
If the file exists, we proceed to retrieve its creation time (ctime
) using os.path.getctime()
and print the ctime
since the epoch
. Additionally, we use the time.ctime()
function to convert the ctime
into a human-readable local time format and print that information as well.
On the other hand, if the file does not exist, we print an error message stating that the file at the specified path does not exist. In summary, this code provides details about the creation time of an existing file and handles the case where the file is not found.
Conclusion
Python’s os.path.getctime()
method efficiently retrieves a file’s creation time (ctime
). It is versatile and applicable to both UNIX and Windows systems, with a simple syntax and a return value representing the access time in seconds.
Through examples, we demonstrated its practical use, including displaying creation time, handling errors, and gracefully managing non-existent files. This method proves valuable for managing and understanding file metadata in Python.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn