Python os.path.getatime() Method
-
Syntax of the
os.path.getatime()
Method -
Example 1: Use the
os.path.getatime()
Method in Python -
Example 2: Use the
os.path.getatime()
Method With a Loop in Python -
Example 3: Handling Errors in the
os.path.getatime()
Method - Conclusion
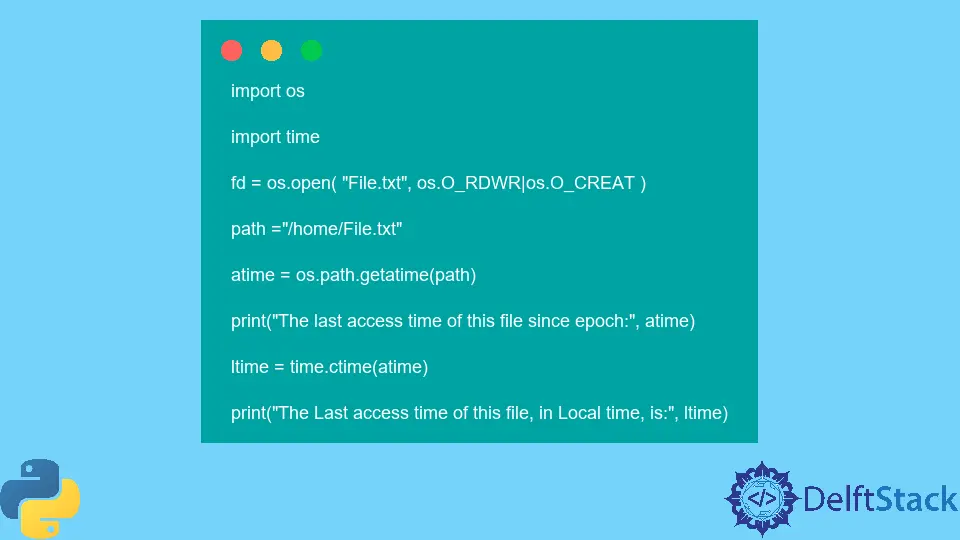
In this article, we’ll explore Python’s os.path.getatime()
method, which is used for retrieving access times of files and directories. This method is essential for tracking when files were last accessed, and we’ll cover its syntax and practical applications.
Syntax of the os.path.getatime()
Method
os.path.getatime(path)
Parameters
path
- an address object of a file system path or a symlink
. The object can either be an str
or bytes.
Return
The return type of this method is a floating-point value representing the recent access time of a specified path in seconds.
Example 1: Use the os.path.getatime()
Method in Python
In this code example, we use the os
and time
modules to work with file access times. First, we open a file named "File1.txt"
using the os.open()
with the flags os.O_RDWR | os.O_CREAT
.
This opens the file in both read and write modes and creates it if it doesn’t exist. The file path is specified as "/Users/Public/File1.txt"
.
Next, we retrieve the access time of the file using os.path.getatime(path)
, where path
is the full path to the file. The access time is a floating-point number representing the time of the most recent access to the file since the epoch
(a reference point in time, often January 1, 1970
, in Unix-like systems).
Then, we print this value with the message "The last access time of this file since epoch:"
. To make this timestamp more human-readable, we use time.ctime(atime)
to convert it to a local time format.
The atime
value is passed to time.ctime()
, and the result is printed with the message "The Last access time of this file, in Local time, is:"
.
import os
import time
fd = os.open("/Users/Public/File1.txt", os.O_RDWR | os.O_CREAT)
path = "/Users/Public/File1.txt"
atime = os.path.getatime(path)
print("The last access time of this file since epoch:", atime)
ltime = time.ctime(atime)
print("The Last access time of this file, in Local time, is:", ltime)
Output:
The last access time of this file since epoch: 1698781698.5668397
The Last access time of this file, in Local time, is: Wed Nov 1 03:48:18 2023
The output shows the access time since the epoch
and the access time in a more user-friendly local time format. In this example, the last access time of the file File1.txt
is displayed, which is the time when the file was last accessed since the epoch
(in seconds) and its equivalent local time in the Wed Nov 1 03:48:18 2023
format.
The epoch
is a common term in computing that refers to a specific point in time that serves as a reference or starting point for measuring time. In most computer systems, the epoch is set to a particular date and time from which all other time values are calculated or measured.
Example 2: Use the os.path.getatime()
Method With a Loop in Python
In this code example, we have a list of file paths named file_paths
, which includes "file1.txt"
, "file2.txt"
, and "file3.txt"
. Then, we start iterating through the list of file_paths
using a for
loop.
Inside the for
loop, we construct the full path to each file by joining the base directory "/Users/Public"
with the file name using os.path.join()
. This creates the full_path
variable, which is used for subsequent operations.
For each file, we retrieve the access time using os.path.getatime(full_path)
. This provides us with a floating-point value representing the time of the most recent access to the file since the Unix epoch.
We then print this access time with a message that includes the file name, such as "The last access time of file1.txt since epoch: {atime}"
. To make this timestamp more user-friendly, we use time.ctime(atime)
to convert it to a local time format.
The result is printed with a message that includes the file name, like "The last access time of file1.txt, in Local time, is: {ltime}"
. Lastly, the code iterates through each file in the list, displaying both the access time since the epoch
and the corresponding local time format.
import os
import time
file_paths = ["file1.txt", "file2.txt", "file3.txt"]
for file_path in file_paths:
atime = os.path.getatime(full_path)
print(f"The last access time of {file_path} since epoch: {atime}")
ltime = time.ctime(atime)
print(f"The last access time of {file_path}, in Local time, is: {ltime}")
Output:
The last access time of file1.txt since epoch: 1698781698.5668397
The last access time of file1.txt, in Local time, is: Wed Nov 1 03:48:18 2023
The last access time of file2.txt since epoch: 1698781732.837468
The last access time of file2.txt, in Local time, is: Wed Nov 1 03:48:52 2023
The last access time of file3.txt since epoch: 1698781737.0606296
The last access time of file3.txt, in Local time, is: Wed Nov 1 03:48:57 2023
The output displays the access times for three files: "file1.txt"
, "file2.txt"
, and "file3.txt"
. It presents these times in two formats: the time in seconds since the Unix epoch
(January 1, 1970
) and the corresponding local time.
For each file, the code provides the access time since the epoch
in seconds and the local time format. This information is useful for tracking when these files were last accessed and gives a human-readable timestamp for each access event.
Example 3: Handling Errors in the os.path.getatime()
Method
In this code example, we import three crucial modules: os
, time
, and sys
. These modules enable us to work with the operating system, manage time-related operations, and handle system-related functionalities.
The code starts by attempting to open a file named "File1.txt"
using the os.open()
function. We specify that the file should be opened in both read and write modes (os.O_RDWR
) and created if it doesn’t exist (os.O_CREAT
).
Next, we define the variable path
, which represents the full path to the file as "/Users/Public/File1.txt"
. We aim to retrieve and display the access time for this file.
Inside a try
block, we call os.path.getatime(path)
to obtain the access time for the specified file path. The access time is a floating-point number that represents the time of the most recent access to the file.
If the file exists and the operation is successful, we use the print()
function to display the access time. The message "The last access time of this file is:"
is printed, followed by the access time in seconds since the Unix epoch
.
However, the code also includes a try
block, anticipating potential exceptions. In this case, if an OSError
occurs (e.g., if the file doesn’t exist or is inaccessible), we handle the exception within the except
block.
Then, we print the message "The path '/Users/Public/File1.txt' does not exist or is inaccessible"
, which informs us about the issue and the inaccessible or non-existent file path.
import os
import time
import sys
fd = os.open("File1.txt", os.O_RDWR | os.O_CREAT)
path = "/Users/Public/File1.txt"
try:
atime = os.path.getatime(path)
print("The last access time of this file is:", atime)
except OSError:
print("The path '%s' does not exist or is inaccessible." % path)
sys.exit()
ltime = time.ctime(atime)
print("The Last access time of this file, in Local time, is:", ltime)
Output:
The path '/Users/Public/File1.txt.txt' does not exist or is inaccessible.
The output indicates that the code tried to retrieve the access time for a file located at the path "/Users/Public/File1.txt"
, but it encountered a problem. Specifically, it couldn’t find the file at the specified path, or it was unable to access it due to some restrictions or issues.
Conclusion
In conclusion, the article introduced the os.path.getatime()
method in Python for accessing file and folder access times. It discussed its syntax, parameters, and practical examples, including error handling for inaccessible or non-existent files.
Overall, the os.path.getatime()
method is a powerful tool for working with access times in Python, aiding in various file management and tracking scenarios.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn