Python os.path.expandvars() Method
- What Are Environment Variables?
-
the Purpose of
os.path.expandvars()
-
Syntax of
os.path.expandvars()
Method -
Example 1: Basic Expansion Using the
os.path.expandvars()
Method in Python -
Example 2: Use the
os.path.expandvars()
Method to Dynamically Resolve Environment Variable References -
Example 3: Use the
os.path.expandvars()
Method on Windows OS -
Example 4: Use an Unspecified Environment Variable in the
os.path.expandvars()
Method -
Example 5: Iterating Through Paths in a List Using the
os.path.expandvars()
Method - Key Points to Remember
- Conclusion
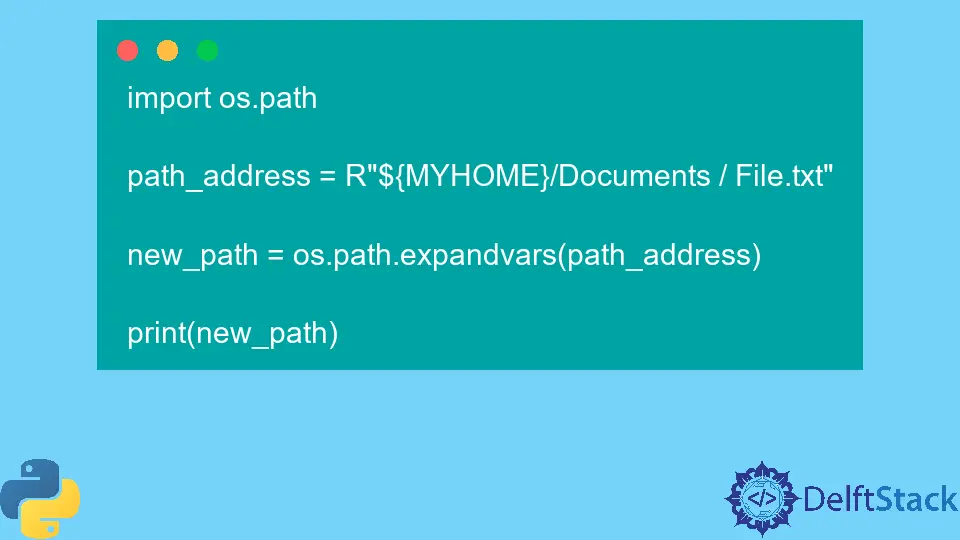
Python’s os.path
module is a powerful tool for handling file paths and directory operations. Among its functionalities, the os.path.expandvars()
method stands out as a convenient means to expand environment variables within a given string.
This method enables the manipulation and resolution of environment variable references, providing enhanced flexibility in path handling.
Python’s os.path.expandvars()
method is an efficient way of expanding the environment variables in a specified path. All the substrings of the form $name
or ${name}
are replaced with the set value of the environment variable name.
What Are Environment Variables?
Before delving into the specifics of os.path.expandvars()
, it’s crucial to comprehend the concept of environment variables. Environment variables are dynamic values that define the environment in which a process runs.
They contain information such as system configuration settings, paths to executable files, user preferences, etc. These variables are accessed by software programs and scripts to adapt behavior based on the environment they are executing in.
the Purpose of os.path.expandvars()
The os.path.expandvars()
method specifically targets strings containing environment variable references. When given a string as an argument, this method scans the string for substrings in the format $VAR
or ${VAR}
and replaces them with their corresponding values from the operating system’s environment variables.
Syntax of os.path.expandvars()
Method
import os
expanded_string = os.path.expandvars(input_string)
Parameters
Here, input_string
represents the string containing the environment variable references, and expanded_string
holds the modified string after the expansion process.
Return Value
The return type of this method is a string value representing the new environment variable after expanding it based on the input parameter.
Example 1: Basic Expansion Using the os.path.expandvars()
Method in Python
The most straightforward use involves directly passing a string with environment variable references to os.path.expandvars()
for expansion.
Let’s illustrate the usage of os.path.expandvars()
with a simple example:
import os
# Define a string containing environment variable reference
path_with_var = "$HOME/Documents"
# Expand the environment variable reference in the string
expanded_path = os.path.expandvars(path_with_var)
print(expanded_path)
Output:
/User/username/Documents
Suppose the $HOME
environment variable is set to /User/username
. In this case, os.path.expandvars()
will replace $HOME
in path_with_var
with /User/username
, resulting in expanded_path
being /User/username/Documents
.
Example 2: Use the os.path.expandvars()
Method to Dynamically Resolve Environment Variable References
In this example, we have replaced the values of the environment variable HOME
and USER
with corresponding values.
import os.path
path_address = "$HOME/File.txt"
new_path = os.path.expandvars(path_address)
print(new_path)
os.environ["HOME"] = "/home/Documents"
path_address = "$HOME/User/File.txt"
new_path = os.path.expandvars(path_address)
print(new_path)
os.environ["USER"] = "programmer"
path_address = "/home/${USER}/File.txt"
new_path = os.path.expandvars(path_address)
print(new_path)
Output:
/root/File.txt
/home/Documents/User/File.txt
/home/programmer/File.txt
In this code, os.path.expandvars()
is used to dynamically resolve environment variable references within strings. Initially, the code defines a path_address
containing the $HOME
variable, and os.path.expandvars(path_address)
expands it to the corresponding value.
The code then reassigns the $HOME
environment variable to a new path and demonstrates how os.path.expandvars()
updates path_address
accordingly. Additionally, it sets the $USER
variable and shows how ${USER}
within a path string gets resolved to its value using os.path.expandvars()
.
Overall, the code showcases the method’s ability to adaptively handle and update paths based on changes in environment variable values using os.path.expandvars()
in Python.
Example 3: Use the os.path.expandvars()
Method on Windows OS
In this example, we have replaced the values of the environment variables HOME
, USERNAME
, and TEMP
with corresponding values.
import os.path
path_address_1 = R"% HOME %\Documents\File.txt"
path_address_2 = R"C:\User\$USERNAME\HOME\File.txt"
path_address_3 = R"${TEMP}\File.txt"
new_path1 = os.path.expandvars(path_address_1)
new_path2 = os.path.expandvars(path_address_2)
new_path3 = os.path.expandvars(path_address_3)
print(new_path1)
print(new_path2)
print(new_path3)
Output:
% HOME %\Documents\File.txt
C:\User\Admin\HOME\File.txt
C:\Users\Admin\AppData\Local\Temp\File.txt
In this code executed on a Windows OS, the os.path.expandvars()
method is utilized to manipulate paths containing environment variable references. However, there are some issues in the defined path strings that might affect the expected results when expanded.
When executed on a Windows OS, the os.path.expandvars()
method will attempt to resolve these variable references. However, due to the incorrect formatting of the environment variables in the path strings, the resulting new_path
variables might not reflect the intended expansions, potentially leading to paths that include the environment variable references verbatim.
Example 4: Use an Unspecified Environment Variable in the os.path.expandvars()
Method
No environment variable is defined in the below code, so the path is unchanged and displayed as-is.
import os.path
path_address = R"${MYHOME}/Documents / File.txt"
new_path = os.path.expandvars(path_address)
print(new_path)
Output:
${MYHOME}/Documents / File.txt
This code uses os.path.expandvars()
to modify a path string that includes an environment variable reference. However, the reference ${MYHOME}
within the path isn’t in the format recognized as a typical environment variable on most systems.
Consequently, when the method is executed, the ${MYHOME}
reference isn’t recognized or expanded as an environment variable. Therefore, the resulting new_path
likely retains the original path string, including the ${MYHOME}
reference, without any modification.
Example 5: Iterating Through Paths in a List Using the os.path.expandvars()
Method
You can apply os.path.expandvars()
within a loop to expand environment variable references within a list of paths.
import os
# Define a list of paths with environment variable references
paths = ["$HOME/Documents/file1.txt", "$HOME/Documents/file2.txt"]
# Expand environment variable references in the list of paths
expanded_paths = [os.path.expandvars(path) for path in paths]
print(expanded_paths)
Output:
['/root/Documents/file1.txt', '/root/Documents/file2.txt']
This code demonstrates a list comprehension where each path in the paths
list is individually expanded using os.path.expandvars()
, resulting in a list of expanded paths.
Key Points to Remember
- The method only expands environment variables that are set in the system’s environment.
- If an environment variable is not set, the method will leave the reference unchanged.
- It supports both
$VAR
and${VAR}
formats for referencing variables.
Use Cases
The os.path.expandvars()
method finds applications in various scenarios:
- Cross-platform Compatibility: It ensures cross-platform compatibility by resolving environment variables specific to the system.
- Configuration Files: When working with configuration files, this method allows the dynamic referencing of paths or values based on environment variables.
- Scripting: In scripts requiring dynamic path manipulation, such as file operations, it provides a convenient way to reference environment-specific paths.
Limitations and Considerations
While os.path.expandvars()
is a versatile tool, there are certain considerations to keep in mind:
- Dependency on Environment Variables: The method’s effectiveness depends on the presence and definition of environment variables.
- Security Concerns: Blindly expanding user-defined strings containing environment variable references might pose security risks if not validated properly.
Conclusion
The os.path.expandvars()
in Python’s os.path
module is a powerful method that resolves environment variable references within strings, allowing for dynamic path manipulation. This functionality enables the replacement of substrings in the format $VAR
or ${VAR}
with their corresponding values from the system’s environment variables.
Understanding environment variables is key to using os.path.expandvars()
effectively, as these variables define the environment in which processes run. The method adapts behavior based on these variables by replacing references in strings with their set values.
Examples illustrate its versatility, showcasing applications like dynamic path construction, cross-platform compatibility, and configuration adaptation. However, it’s crucial to consider limitations such as dependency on defined environment variables and potential security risks when dealing with user-defined strings containing variable references.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn