Python os.open() Method
-
Syntax of the
os.open()
Method -
Example Codes: Working With the
os.open()
Method in Python -
Example Codes: Read the File After Using the
os.open()
Method in Python -
Example Codes: Use the
os.fdopen
Method With theos.open()
Method in Python - Conclusion
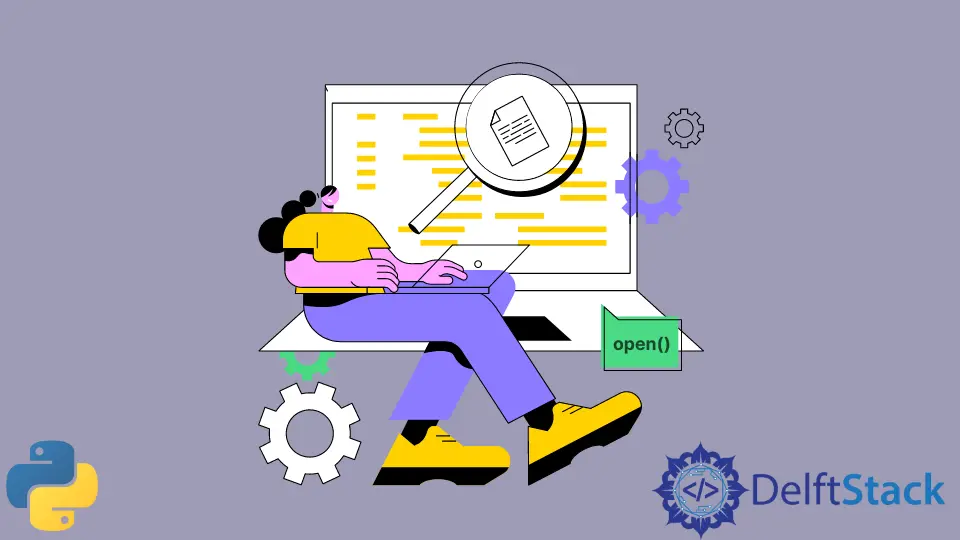
Python os.open()
method is an efficient way of creating OS-level file descriptors and setting various flags and modes accordingly.
Syntax of the os.open()
Method
os.open(path address, flag, mode)
os.open(path address, flag, mode, fd_dir)
Parameters
path address |
It is an address object of a file system path or a symlink. The object can either be str or bytes. |
flag |
List of all the flags that could be set on the new file: |
1. os.O_RDONLY : flag used to open a file for reading only |
|
2. os.O_WRONLY : flag used to open a file for writing only |
|
3. os.O_RDWR : flag used to open a file for reading and writing both |
|
4. os.O_NONBLOCK : flag used not to block an opened file |
|
5. os.O_APPEND : flag used to append a file on each write |
|
6. os.O_CREAT : flag used to create a file if it already doesn’t exist |
|
7. os.O_TRUNC : flag used to truncate a file size to 0 |
|
8. os.O_EXCL : flag used to show an error while creating a file that already exists |
|
9. os.O_SHLOCK : flag used to obtain a shared lock for a file atomically |
|
10. os.O_EXLOCK : flag used to obtain an exclusive lock for a file atomically |
|
11. os.O_DIRECT : flag used to remove or reduce cache effects in a file |
|
12. os.O_FSYNC : flag used to write file synchronously |
|
13. os.O_NOFOLLOW : flag used to set the file that doesn’t follow a symlink |
|
mode |
It is an optional parameter representing the mode of the newly opened file. The default value of the mode is an octal numeric integer 0o777 . |
fd_dir |
It is an optional parameter representing a file descriptor referring to a path. |
Return
This method returns the newly opened file’s path and file descriptor.
Example Codes: Working With the os.open()
Method in Python
In this example code, it creates or opens a file named File.txt
, writes the string Hello, World!
to it, and then closes the file. The final print
statement notifies the user that the file has been closed successfully.
File.txt
:
Hello, World!
Example Code:
import os
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
os.write(fd, "Hello, World!".encode())
os.close(fd)
print("The file has been closed successfully!")
The code begins by importing the os
module, providing access to operating system functionalities.
Subsequently, it opens or creates a file named File.txt
in read-write mode, obtaining a file descriptor (fd
) as an identifier for the opened file.
The use of os.O_CREAT
ensures file creation if it doesn’t exist. The os.write()
function is then employed to encode and write the string Hello, World!
to the file, utilizing the file descriptor obtained earlier.
Finally, the script closes the file using os.close(fd)
to release system resources.
A concluding print
statement informs the user that the file has been closed successfully, irrespective of the outcomes of file creation and writing operations.
Output:
The file has been closed successfully!
The output The file has been closed successfully!
indicates that the process was executed successfully.
While using the os.open()
method, we can use one or more modes simultaneously by using the vertical bar |
.
Example Codes: Read the File After Using the os.open()
Method in Python
The code snippet below demonstrates file handling using the os
module.
It begins by specifying a file path, setting file permissions, and defining flags for file creation and read-write access.
The code then opens a file, writes a string to it, reads the content, and finally closes the file. Throughout the process, it prints messages to inform the user about the progress and success of each operation.
File.txt
:
Hello, World!
Example Code:
import os
path_address = "File.txt"
mode_set = 0o666
flags = os.O_CREAT | os.O_RDWR
descriptor = os.open(path_address, flags, mode_set)
print("The new file has been created.")
line = "Hello, World! Programming is fun!"
os.write(descriptor, line.encode())
print("Text has been successfully entered in the file.")
os.lseek(descriptor, 0, 0)
line = os.read(descriptor, os.path.getsize(descriptor))
print("Reading the text from the file:")
print(line.decode())
os.close(descriptor)
print("The file descriptor has been closed successfully.")
In order to discuss further, the code utilizes the os
module for file handling operations. It begins by importing the os
module, providing access to various operating system functionalities, particularly those related to file operations.
The file path is defined as "./File.txt,"
setting the stage for new file creation or access within the current directory. The file permissions are specified as 0o666
, granting read and write permissions to the owner, group, and others.
The code script sets flags for file operations using os.O_CREAT
to create the file if it doesn’t exist and os.O_RDWR
for read-write access.
It then opens the file with the given path, flags, and permissions, obtaining a file descriptor (descriptor
) that serves as a low-level identifier for the opened file. A confirmation message is printed to indicate the successful creation or access of the new file.
A string, Hello, World! Programming is fun!
is generated and written to the file using the file descriptor. The user is notified of the successful text entry into the file.
The code script then positions the file cursor at the beginning of the file, preparing for reading. It reads the file’s content using the file descriptor and the size obtained from os.path.getsize()
. The user is informed of the upcoming display of the file content.
The content is decoded and printed to the console, providing a view of the text stored in the file.
Finally, the file is closed using the file descriptor to release system resources, and a closing success message is displayed.
Output:
The new file has been created.
Text has been successfully entered in the file.
Reading the text from the file:
Hello, World! Programming is fun!
The file descriptor has been closed successfully.
Keep in mind that it is a healthy practice for any programmer to close all the newly opened files using the os.close()
method as it ensures the proper release of system resources associated with the opened files.
Failing to close files may lead to resource leaks and potential issues, especially when dealing with a large number of files or long-running programs.
Example Codes: Use the os.fdopen
Method With the os.open()
Method in Python
In this example, the code script below employs the os
module to open or create a file named File.txt
with read-write access using os.open()
.
The file descriptor obtained is then used to open a file object with os.fdopen()
. Within a with
statement, the script writes the string Hello, World!
to the file using the file object.
Upon exiting the with
block, the file is automatically closed. A concluding message is printed to notify the user of the successful closure of the file descriptor.
File.txt
:
Hello, World!
Example Code:
import os
with os.fdopen(os.open("File.txt", os.O_CREAT | os.O_RDWR), "w") as fd:
fd.write("Hello, World!")
print("The file descriptor has been closed successfully.")
To do the file handling, the os
module is imported in the code snippet.
The code, with os.fdopen(os.open("File.txt", os.O_CREAT | os.O_RDWR), "w") as fd:
, combines os.open()
and os.fdopen()
, this line opens or creates the file File.txt
with read-write access.
The file descriptor is obtained using os.open()
, and os.fdopen()
is used to create a file object (fd
) associated with this descriptor. The w
mode indicates that the file will be opened in write mode.
fd.write("Hello, World!")
writes the string Hello, World!
to the file using the file object (fd
).
Lastly, it informs the user that the file descriptor has been automatically closed upon exiting the with
block.
Output:
The file descriptor has been closed successfully.
The output The file descriptor has been closed successfully.
indicates that the process was executed successfully.
Note: The returned file descriptor from this method is non-inheritable.
Conclusion
In conclusion, the os.open()
method in Python serves as a powerful tool for creating OS-level file descriptors with various flags and modes.
The method’s syntax involves specifying the file path, setting flags for operations like read, write, and creation, and providing optional parameters for file permissions and existing file descriptors.
The examples demonstrate its application in file creation, writing, reading, and automatic closure, showcasing its versatility by showcasing efficient file handling. Additionally, the use of os.fdopen()
within a with
statement simplifies the file handling process, ensuring proper resource management.
The method’s flexibility and comprehensive functionality make it a valuable asset for developers engaging in low-level file operations.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn