Python os.lseek() Method
-
Syntax of Python
os.lseek()
Method -
Example 1: Use
os.lseek()
to Seek the File From the Beginning -
Example 2: Use
os.lseek()
to Seek the File From a Specific Position -
Example 3: Use
os.lseek()
to Seek the File From the End -
OSError: [Errno 22] Invalid argument
Encounter in Usingos.lseek()
Method - Conclusion
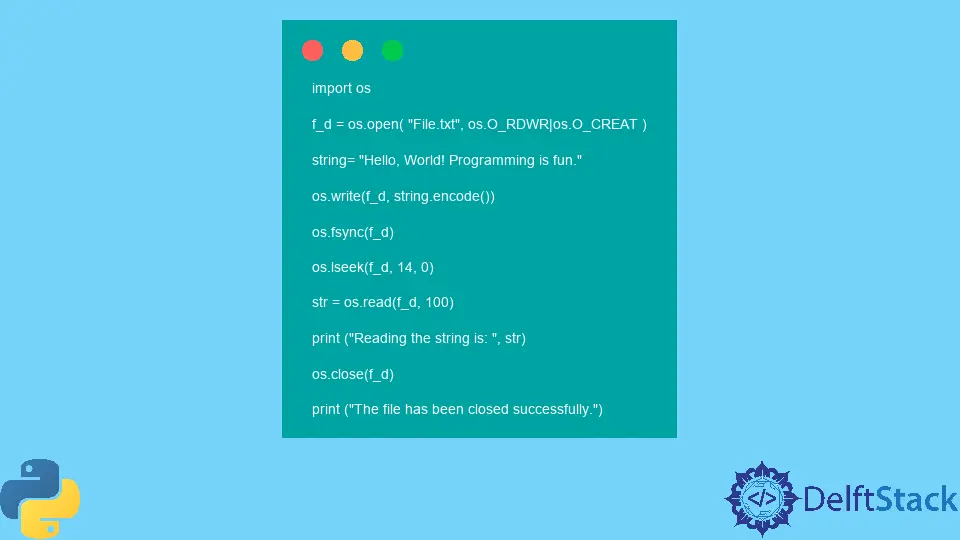
In Python programming, file manipulation is a common task, and the os
module provides several functions to work with files at a lower level than what’s available in the built-in open()
function.
One such function is os.lseek()
, which allows you to change the current file position within an open file.
Python os.lseek()
method is an efficient way of setting the current position of a file descriptor fd
to a new position pos
, depending on how
mode.
This can be particularly useful when you need to move the file pointer to a specific location within the file without reading or writing data.
Understanding how to use os.lseek()
effectively can enhance your file handling capabilities in Python.
Syntax of Python os.lseek()
Method
os.lseek(fd, pos, how)
Parameters
fd |
The file descriptor/path to be processed. |
pos |
It determines the position in the file depending on the following parameters: |
1. SEEK_SET/0 : It sets the position to the beginning of the file. |
|
2. SEEK_CUR/1 : It sets the position relative to the current position in the file. |
|
3. SEEK_END/2 : It sets the position relative to the end of the file. |
|
how |
It is the reference point in the file depending on the following parameters: |
1. SEEK_SET/0 : It sets the reference point to the beginning of the file. |
|
2. SEEK_CUR/1 : It sets the reference point to the current position in the file. |
|
3. SEEK_END/2 : It sets the reference point to the end of the file. |
Return
In the execution process, this method does not return any value.
Example 1: Use os.lseek()
to Seek the File From the Beginning
This example will walk you through a practical example of using os.lseek()
to manipulate a file in Python.
We’ll create a file, write some text into it, move the file pointer, read the content from the updated position, and finally close the file.
By the end, we’ll have a clear understanding of how to use os.lseek()
effectively in Python programs.
File.txt
:
New file text.
Example code:
import os
# Define the file path
path_address = "./File.txt"
# Set file permissions
mode_set = 0o666
# Define flags for file opening
flags = os.O_CREAT | os.O_RDWR
# Open the file with the specified flags and mode
descriptor = os.open(path_address, flags, mode_set)
# Inform the user that the file has been created
print("The new file has been created.")
# Write text into the file
line = "Hello, World! Programming is fun!"
os.write(descriptor, line.encode())
# Inform the user about successful text entry
print("The text has been successfully entered into the file.")
# Move the file pointer to the beginning of the file
os.lseek(descriptor, 0, 0)
# Read the text from the file
line = os.read(descriptor, os.path.getsize(descriptor))
# Inform the user about reading the text from the file
print("Reading the text from the file:")
# Decode and print the text
print(line.decode())
# Close the file descriptor
os.close(descriptor)
# Inform the user about successful file descriptor closure
print("The file descriptor has been closed successfully.")
The Python code above demonstrates the usage of various os
module functions, primarily focusing on file manipulation.
Initially, the code defines a file path, sets file permissions, and specifies flags for opening the file with os.open()
. After successfully creating the file, a string containing "Hello, World! Programming is fun!"
is encoded and written into the file using os.write()
.
Next, the code employs os.lseek()
to move the file pointer to the beginning of the file, ensuring that subsequent read operations start from the start.
The content of the file is then read using os.read()
and decoded into a human-readable format.
Finally, the file descriptor is closed using os.close()
.
Overall, the code provides a clear illustration of how to create, write, read, and close files in Python using the os
module functions, with particular emphasis on controlling the file pointer’s position using os.lseek()
.
Output:
This output demonstrates the successful creation of a file, writing text into it, reading the text from the file, and finally closing the file descriptor.
The use of os.lseek()
ensures that the file pointer is moved to the desired position within the file before reading its content.
Example 2: Use os.lseek()
to Seek the File From a Specific Position
This example will explore a Python code that demonstrates the usage of os
module functions for file manipulation, including os.open()
, os.write()
, os.fsync()
, os.lseek()
, os.read()
, and os.close()
.
Each function plays a crucial role in handling files efficiently, and by understanding how they work together, you can gain better control over file operations in your Python programs.
File.txt
:
New file text.
Example code:
import os
# Open or create a file in read-write mode
f_d = os.open("File.txt", os.O_RDWR | os.O_CREAT)
# Define a string to write into the file
string = "Hello, World! Programming is fun."
# Write the string into the file after encoding it
os.write(f_d, string.encode())
# Ensure that any changes to the file are synchronized with the underlying storage device
os.fsync(f_d)
# Move the file pointer to the 14th byte from the beginning of the file
os.lseek(f_d, 14, 0)
# Read up to 100 bytes from the current file pointer position
str = os.read(f_d, 100)
# Print the string read from the file
print("Reading the string is: ", str)
# Close the file descriptor
os.close(f_d)
# Inform the user about successful file closure
print("The file has been closed successfully.")
The provided code showcases a comprehensive file manipulation process in Python.
It begins by opening or creating a file using os.open()
, then writes a string into the file using os.write()
, ensuring changes are synchronized with the storage device with os.fsync()
.
The file pointer is then moved to a specific position using os.lseek()
, followed by reading a portion of the file with os.read()
.
Finally, the file is closed using os.close()
. This example highlights the versatility of the os
module functions in handling files efficiently and demonstrates how to perform various file operations seamlessly in Python.
Output:
Note that some new operating systems can support more values for pos
and how
parameters, like os.SEEK_HOLE
or os.SEEK_DATA
.
Example 3: Use os.lseek()
to Seek the File From the End
In this example, the os.lseek()
method seeks the file from the end and prints it.
example.txt
:
Hello, World!
Example code:
import os
# Open the file in read mode
file_path = "example.txt"
file_descriptor = os.open(file_path, os.O_RDONLY)
# Get the size of the file
file_size = os.path.getsize(file_path)
# Seek 10 bytes backward from the end of the file
os.lseek(file_descriptor, -10, os.SEEK_END)
# Read and print the content from the current file pointer position
print("Content from the position 10 bytes before the end of the file:")
print(os.read(file_descriptor, 10).decode())
# Close the file
os.close(file_descriptor)
Output:
OSError: [Errno 22] Invalid argument
Encounter in Using os.lseek()
Method
Sometimes, a program with no error handling could lead to trigger an error.
In this example, OSError: [Errno 22] Invalid argument
was triggered by seeking a negative offset using os.lseek()
. Seeking a negative offset is generally considered invalid and would likely result in an error:
example.txt
:
Hello, World!
Example code:
import os
# Open a file in read mode
file_path = "example.txt"
file_handle = os.open(file_path, os.O_RDONLY)
# Seek to a negative position (which is invalid)
offset = -100
os.lseek(file_handle, offset, os.SEEK_SET)
data = os.read(file_handle, 100)
print("Data read:", data)
# Close the file handle
os.close(file_handle)
The code imports the os
module, which provides functions for interacting with the operating system.
It opens a file named example.txt
in read-only mode using the os.open()
function, which returns a file descriptor.
The code attempts to seek a negative offset (-100
) within the file using the os.lseek()
function, which positions the file pointer to the specified offset relative to the beginning of the file (os.SEEK_SET
).
Since seeking a negative offset is invalid, an OSError
exception is expected to be raised.
Inside the try
block, the code attempts to read 100 bytes from the file using os.read()
.
If an OSError
occurs during the seek operation, the exception is caught in the except
block and an error message is printed.
The file handle is closed using os.close()
to release system resources.
Output:
Traceback (most recent call last):
File "C:/Users/Admin/Desktop/main.py", line 10, in <module>
os.lseek(file_handle, offset, os.SEEK_SET)
OSError: [Errno 22] Invalid argument
Upon executing the code, the OSError: [Errno 22] Invalid argument
error will show.
To avoid encountering errors when using the os.lseek()
function, especially when seeking a specific position within a file.
Always ensure that the offset value passed to os.lseek()
is within the valid range of file positions.
Conclusion
This article provides an overview of file manipulation in Python, emphasizing the os.lseek()
function’s role in adjusting the file pointer’s position.
The function allows precise positioning within a file, which is crucial for efficient file operations. Understanding its syntax and parameters is essential, as demonstrated in practical examples.
The code exhibits error handling, illustrating the importance of ensuring valid offset values to prevent errors like triggering an OSError
.
By adhering to best practices and proper error handling, programmers can effectively utilize os.lseek()
to enhance file manipulation capabilities in Python.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn