Python os.kill() Method
-
Understanding the
os.kill()
Method in Python -
Example Codes 1: Using
os.getpid()
Function -
Example Codes 2: Using
os.fork()
Function -
Example Codes 3: Using
time
Module - Conclusion
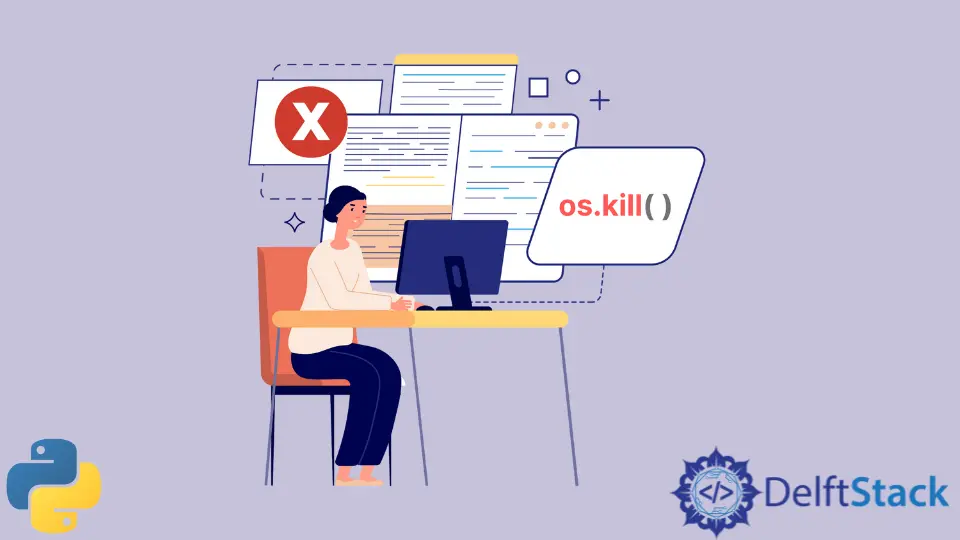
Python os.kill()
method is an efficient way of killing or terminating a process using its process identifier (pid
).
Syntax
The syntax for the os.kill()
method is as follows:
os.kill(pid, signal)
Parameters
pid |
The process ID to which the signal will be sent. |
signal |
The signal to send, which can be specified either as a signal number or a signal name. |
Return
In the execution process, this method does not return any value.
Understanding the os.kill()
Method in Python
The os.kill()
method is part of the os
module in Python and is used to send signals to processes.
A signal is a software interrupt delivered to a process, indicating a specific event or action. Common signals include termination signals (SIGTERM
) for termination and kill signals (SIGKILL
) for forced termination.
The PID uniquely identifies a running process in the operating system, and the signal specifies the action to be taken by the target process. Common signals include terminating a process, interrupting its execution, or requesting it to reload configuration.
When using os.kill()
, it’s essential to consider the platform on which your code is running due to the availability and behavior of signals can vary between different operating systems.
Note: The following code example best works at your local computer compiler as an online compiler environment may have restrictions or limitations that affect the termination of processes and subprocesses. It’s important to note that the behavior can vary depending on the platform and the specific environment.
Example Codes 1: Using os.getpid()
Function
The example Python code snippet below demonstrates a simple program that retrieves its own process ID (PID) using the os.getpid()
function, prints the PID to the console, and then terminates itself using the os.kill()
function with the signal.SIGKILL
signal.
import os
import signal
def process():
print("The process ID:", os.getpid())
os.kill(os.getpid(), signal.SIGKILL)
process()
In the above code, we use the os.getpid()
to retrieve the process ID of the current process. The print("The process ID:", os.getpid())
prints the process ID.
Finally, the os.kill(os.getpid(), signal.SIGKILL)
sends the SIGKILL
signal to the current process, causing it to terminate abruptly.
Output:
The process ID: 6362
Every time we run the above code, a new process executes; thus, different process IDs are obtained. In the example code, the variable signal.SIGKILL
is used to kill the current process.
It’s important to note that using signal.SIGKILL
results in an immediate and ungraceful termination of the process, and any data that is not saved may be skipped.
Example Codes 2: Using os.fork()
Function
This example Python code illustrates the use of the os.fork()
function to create a new process (child process) in a parent-child relationship. The parent process sends signals to control the execution of the child process using os.kill()
and specific signals, such as signal.SIGSTOP
(stop) and signal.SIGCONT
(continue).
The os.fork()
function is specific to Unix-like operating systems (e.g., Linux, macOS), and it is not available on all platforms, including some Windows systems. If you’re using an Integrated Development Environment (IDE) that doesn’t support forking, or if you’re working in a Windows environment, you might encounter an error like "AttributeError: module 'os' has no attribute 'fork'"
.
The child process prints a simple "Hello, World!"
message.
import os
import signal
pID = os.fork()
if pID:
print("---PARENT PROCESS---")
os.kill(pID, signal.SIGSTOP)
print("The parent process has been killed.")
os.kill(pID, signal.SIGCONT)
else:
print("Hello, World!")
In the above code example, pID = os.fork()
creates a new process by duplicating the existing process. The return value (pID
) is used to differentiate between the parent and child processes.
If pID
is non-zero, it means the script is in the parent process. The print("---PARENT PROCESS---")
line prints a message indicating that it’s the parent process.
The os.kill(pID, signal.SIGSTOP)
sends the SIGSTOP
signal to the child process, causing it to pause its execution. And the os.kill(pID, signal.SIGCONT)
sends the SIGCONT
signal to the child process, allowing it to resume execution.
If pID
is zero, it means the script is in the child process.
Output:
---PARENT PROCESS---
The parent process has been killed.
Hello, World!
This illustrates basic process control using signals, with the parent process pausing and resuming the child process. It’s important to note that the os.fork()
method is specific to Unix-like systems, and the behavior may differ on other operating systems.
Example Codes 3: Using time
Module
Let’s consider a practical scenario where we want to gracefully terminate a subprocess after a certain period with the help of the time
module.
The example Python code snippet demonstrates how to use the subprocess
module to launch a separate subprocess and control it using signals. The example starts a subprocess that runs an infinite loop and then sends a SIGTERM
signal after a certain duration to terminate the subprocess gracefully.
We also used the os.getpid()
to print the process ID (PID) for visual output.
import os
import signal
import subprocess
import time
def run_subprocess():
# Start a subprocess
process = subprocess.Popen(["python", "-c", "while True: pass"])
# Will print the process ID
print("The process ID:", os.getpid())
# Wait for 5 seconds
time.sleep(5)
# Send a SIGTERM signal to terminate the subprocess
os.kill(process.pid, signal.SIGTERM)
# Wait for the subprocess to complete
process.wait()
# Call the function to run the example
run_subprocess()
We start by importing the necessary modules - os
, signal
, subprocess
, and time
. These are the fundamentals to call the functions needed for running the program.
We use subprocess.Popen()
to initiate a subprocess that runs an infinite loop (while True: pass
). This loop simulates an ongoing process. We print the process ID by using os.getpid()
to see different results every time we run the code.
To allow the subprocess to run for a specified duration, we use time.sleep(5)
to pause the execution of the main program.
We employ os.kill()
to send a SIGTERM
signal to the subprocess, indicating that it should terminate gracefully. Lastly, we use process.wait()
to ensure that the subprocess completes its execution.
Output:
The process ID: 12400
Note: The process ID will differ every time you run the code.
Conclusion
The os.kill()
method provides a flexible and efficient means of interacting with processes in Python, offering control over their execution and allowing developers to implement process management methods in their applications.
Common termination signal (SIGTERM
) is used for termination of the process and performs cleanup operation before exiting, and (SIGKILL
) is used for forced termination of the process in which it does not give a chance to clean up resources. The method does not return any value.
Always exercise caution and thoroughly test your code, especially when dealing with process manipulation and termination. Document your assumptions and make sure your script behaves predictably across different operating systems and environments.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn