Python os.isatty() Method
-
Understanding the
os.isatty()
Method -
Syntax of Python
os.isatty()
Method -
Example 1: Use the
os.isatty()
Method to Check if the File Descriptor Is Open and Connected to thetty(-like)
Device -
Example 2: Create a New File and Use the
os.isatty()
Method - Example 3: Determining if the Script Is Running in an Interactive Shell
- Example 4: Conditional Behavior in Script Output
- Example 5: Redirecting Output Based on Terminal Presence
- Considerations and Caveats
- Conclusion
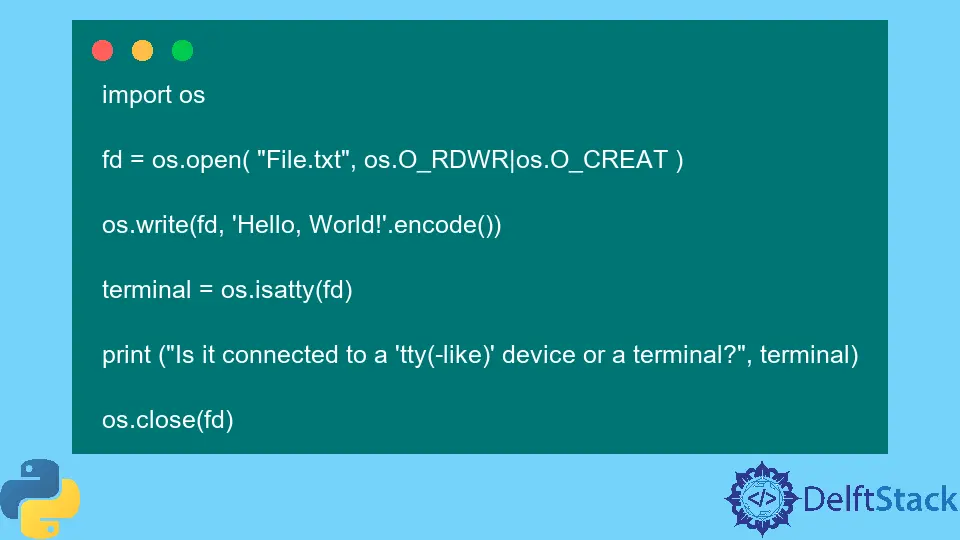
Python, a versatile and powerful programming language, provides a rich set of modules in its standard library to interact with the operating system. Among these modules, the os
module stands out, offering functionalities for interacting with the operating system. One particularly interesting method within this module is os.isatty()
.
Python os.isatty()
method is an efficient way of checking whether a specified file descriptor (fd
) is open or not and connected to a tty(-like)
device. The tty(-like)
device means any device that can act like a teletype, i.e., a terminal.
In this article, we will delve into the details of the os.isatty()
method, exploring its purpose, syntax, and practical applications.
Understanding the os.isatty()
Method
The os.isatty()
method is designed to check whether a given file descriptor refers to a terminal or not. A file descriptor, in this context, is an integer associated with an open file, socket, or other input/output resource.
The os.isatty()
method returns True
if the file descriptor is associated with a terminal and False
otherwise.
Syntax of Python os.isatty()
Method
os.isatty(fd)
Parameters
fd
: It is a file descriptor that needs to be checked.
Return
The return type of this method is a Boolean value. The Boolean value True
is returned if the specified fd
is open and connected to any tty(-like)
device; otherwise, False
is returned.
Example 1: Use the os.isatty()
Method to Check if the File Descriptor Is Open and Connected to the tty(-like)
Device
The example below demonstrates the use of os.isatty()
to check if certain file descriptors are connected to a terminal or terminal-like device. The code first creates a regular pipe and checks the read end to determine its connection to a terminal.
It then creates a pseudo-terminal pair and checks the master side for a similar connection. The os.isatty()
method helps identify whether the associated file descriptors are linked to terminal devices, providing insights into the communication environment or terminal-related operations in the program.
import os
r, w = os.pipe()
print("Is it connected to a 'tty(-like)' device or a terminal?: ", os.isatty(r))
master, slave = os.openpty()
print("Is it connected to a 'tty(-like)' device or a terminal?: ", os.isatty(master))
Output:
Is it connected to a 'tty(-like)' device or a terminal?: False
Is it connected to a 'tty(-like)' device or a terminal?: True
We open a new pseudo-terminal pair in the above code using the os.openpty()
method. It will return us a master
and slave
pair file descriptor, and we then use the os.isatty()
method to check if it can connect to a terminal or not.
Example 2: Create a New File and Use the os.isatty()
Method
The example below utilizes the os
module to perform file operations. It opens a file named "File.txt"
, and writes the string "Hello, World!"
to it, checks whether the file descriptor is connected to a terminal using os.isatty()
, and prints the result.
Finally, the file is closed. The script demonstrates a typical sequence of file-handling operations, including checking the connection to a terminal.
import os
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
os.write(fd, "Hello, World!".encode())
terminal = os.isatty(fd)
print("Is it connected to a 'tty(-like)' device or a terminal?", terminal)
os.close(fd)
Output:
Is it connected to a 'tty(-like)' device or a terminal? False
A file’s fd
is an integer value corresponding to resources, like a pipe or network socket.
Example 3: Determining if the Script Is Running in an Interactive Shell
In this example, the script checks if the standard input (file descriptor 0
) is associated with a terminal. If it is, the script prints a message indicating that it is running in an interactive shell.
import os
if os.isatty(0):
print("Running in an interactive shell.")
else:
print("Running in a non-interactive shell or script.")
Output:
Running in a non-interactive shell or script.
Example 4: Conditional Behavior in Script Output
Here, the script conditionally formats the output message based on whether the standard output (file descriptor 1
) is associated with a terminal. In this example, the message is displayed in green if the output is a terminal.
import os
def display_message(message):
if os.isatty(1): # Check if standard output is a terminal
# Apply green color if output is a terminal
print(f"\033[1;32m{message}\033[0m")
else:
print(message)
display_message("This message has conditional formatting.")
Output:
This message has conditional formatting.
Example 5: Redirecting Output Based on Terminal Presence
In this example, the script redirects the standard output to a file (output.txt
) if it is not associated with a terminal. This can be useful in scenarios where you want to capture or redirect script output based on the runtime environment.
import os
import sys
output_file = open("output.txt", "w")
if os.isatty(1):
# Output is a terminal redirect to a file
original_stdout = sys.stdout
sys.stdout = output_file
print("This message might be redirected to a file if not running in a terminal.")
if os.isatty(1):
# Reset stdout if redirected
sys.stdout = original_stdout
output_file.close()
Output in the "output.txt"
file:
This message might be redirected to a file if not running in a terminal.
Considerations and Caveats
- Platform Differences: The behavior of
os.isatty()
may vary between different operating systems. It’s essential to consider platform-specific behavior when using this method. - File Descriptors: Understanding file descriptors is crucial. The use of
0
for standard input,1
for standard output, and2
for standard error is conventional, but other file descriptors can be used.
Conclusion
The os.isatty()
method in Python’s os
module provides a straightforward way to determine whether a given file descriptor is associated with a terminal. Its versatility allows scripts to adapt their behavior based on the runtime environment, whether running in an interactive shell, redirecting output, or applying conditional formatting.
While simple in its implementation, the os.isatty()
method proves to be a valuable tool for enhancing the flexibility and adaptability of Python scripts in various operating environments.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn