Python os.getppid() Method
- Understanding Process Hierarchy and PID
-
Syntax of Python
os.getppid()
Method -
Example 1: Use the
os.getppid()
Method in Python -
Example 2: Make a Child Process in the
os.getppid()
Method -
Example 3: Make a Grandchild Process in the
os.getppid()
Method - Practical Use Cases
- Considerations and Platform Compatibility
- Conclusion
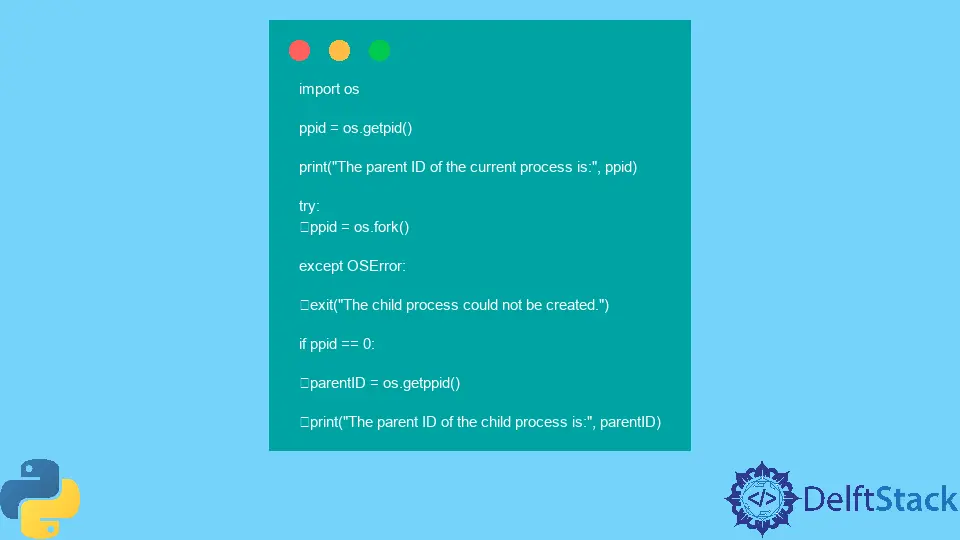
The os.getppid()
method in Python is a valuable utility for retrieving the Process ID (PID) of the parent process of the currently running process. This function, residing in the os
module, provides essential information about process hierarchy and is particularly useful in various system-related tasks and process management.
Understanding Process Hierarchy and PID
In a multitasking operating system, processes are executed independently, and each process is assigned a unique identifier known as the Process ID or PID.
These processes often form a hierarchical structure, with child processes spawned by parent processes. The os.getppid()
method specifically focuses on retrieving the PID of the parent process.
Syntax of Python os.getppid()
Method
os.getppid()
Parameters
This method doesn’t accept any parameters.
Return Value
The return type of this method is an integer value that represents the parent process ID of the process in the running.
Example 1: Use the os.getppid()
Method in Python
This example employs the os
module to access os.getppid()
for retrieving and displaying the Process ID (PID) of the parent process of the current script. The code defines a function, get_parent_process()
, which uses os.getppid()
to obtain the parent process PID and print it.
Executing this code provides insight into the hierarchical relationship between the script’s process and its parent process within the operating system environment.
import os
def get_parent_process():
parent_pid = os.getppid()
print(f"The PID of the parent process is: {parent_pid}")
# Calling the function to retrieve the parent process PID
get_parent_process()
Output:
The PID of the parent process is: 26160
While multiple processes are running, you can use the os.getppid()
method to know which parent function is being executed in that instance.
Example 2: Make a Child Process in the os.getppid()
Method
This example illustrates the usage of os.getpid()
to obtain the PID of the current process (parent process) and attempts to create a child process using os.fork()
.
Upon successful creation of the child process, it retrieves and displays the PID of the parent process of the child process, demonstrating the concept of process creation and hierarchy within an operating system environment.
import os
ppid = os.getpid()
print("The parent ID of the current process is:", ppid)
try:
ppid = os.fork()
except OSError:
exit("The child process could not be created.")
if ppid == 0:
parentID = os.getppid()
print("The parent ID of the child process is:", parentID)
Output:
The parent ID of the current process is: 2306
The parent ID of the child process is: 2306
On any UNIX system, when the parent process has exited, the id returned is of the init
process.
Example 3: Make a Grandchild Process in the os.getppid()
Method
This example demonstrates the creation of a child process from the original (parent) process using os.fork()
in a Unix-like environment. It displays the PIDs of the parent, child, and grandchild processes, illustrating the concept of process creation and hierarchy.
The child process inherits the parent’s PID, and the grandchild process inherits the parent ID from its parent (child process). This showcases the PID relationships among the processes spawned through forking.
import os
import sys
ppid = os.getpid()
print("The parent ID of the current process is:", ppid)
print("From the child process:")
print("My process id is:%d" % (os.getpid()))
sys.stdout.flush() # Explicitly flush the output buffer
val = os.fork()
if val == 0:
print("From the grandchild process:")
print("From the grandchild process: CHILD ID is:%d" % (os.getpid()))
print("From the grandchild process: PARENT ID is:%d" % (os.getppid()))
print("From the grandchild process: GRANDPARENT ID is %d" % (ppid))
print("The grandchild process is exiting.")
Output:
The parent ID of the current process is: 1716
From the child process:
My process id is:1716
From the grandchild process:
From the grandchild process: CHILD ID is:1720
From the grandchild process: PARENT ID is:1716
From the grandchild process: GRANDPARENT ID is 1716
The grandchild process is exiting.
On any Windows OS, when the parent process has exited, the id returned is the same ID, which is reusable.
Practical Use Cases
Process Management
The os.getppid()
method finds utility in process management tasks, allowing developers to identify and understand the hierarchical relationships between various processes running on the system. This information can be crucial when designing applications that involve multiple processes interacting with each other.
System Monitoring
System monitoring tools and utilities often utilize the os.getppid()
method to gather information about process hierarchies, aiding in system analysis, performance optimization, and troubleshooting.
Resource Allocation
In certain scenarios, understanding the parent-child relationships between processes assists in resource allocation and optimization strategies. By obtaining the parent PID, resource allocation decisions can be more informed and efficient.
Considerations and Platform Compatibility
It’s important to note that the behavior of os.getppid()
might vary across different operating systems. While it’s widely available in Unix-like systems (such as Linux and macOS), its behavior in Windows may differ, or it might not be directly available due to differences in the underlying system architecture.
Conclusion
The os.getppid()
method in Python’s os
module retrieves the Process ID (PID) of the parent process. This article explores its syntax, usage, and practical applications.
It showcases examples illustrating its role in understanding process hierarchy, creating child processes, and identifying PID relationships. Additionally, it highlights its usefulness in process management, system monitoring, and resource allocation.
Considering platform differences, the method’s behavior might vary across operating systems. Overall, os.getppid()
is a crucial tool for managing processes and gaining insights into their hierarchical relationships within an operating system environment.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn