Python os.getpid() Method
-
Syntax of Python
os.getpid()
Method -
Example 1: Use
os.getpid()
to Get Process ID of Any Process -
Example 2: Use
os.getpid()
to Get Process ID of Parent and Child Process
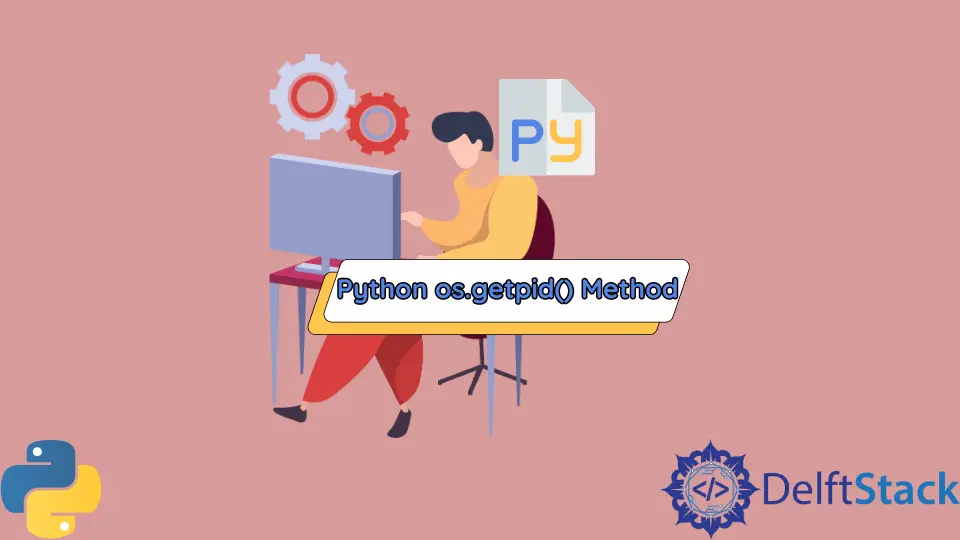
The getpid()
belongs to the os
module in the Python programming language. The os
module is an in-built library available in Python that offers various functions to communicate with the operating system.
The getpid()
method returns the process ID of the current process.
Syntax of Python os.getpid()
Method
os.getpid()
Parameters
This method doesn’t accept any parameters.
Return
The getpid()
method returns the process ID of the currently running process. The process ID is an integer value and a unique identifier assigned to every process running in the system so that the operating system can manage and identify them without hassle.
Example 1: Use os.getpid()
to Get Process ID of Any Process
import os
pid = os.getpid()
print(pid)
Output:
735
The process ID can be any integer value; hence, the output varies from machine to machine.
Example 2: Use os.getpid()
to Get Process ID of Parent and Child Process
The os
module offers a fork()
method that allows us to create a child process. It returns the process ID of the child process; a negative value means failure at creation.
The process that executes this method is known as the parent process.
import os
pid = os.fork()
if pid > 0:
print("Parent Process ID:", os.getpid())
print("Child Process ID:", pid)
elif pid == 0:
print("Parent Process ID:", os.getppid())
print("Child Process ID:", os.getpid())
else:
print("Can't create a child process :(")
Output:
Parent Process ID: 3672
Child Process ID: 3673
Parent Process ID: 3672
Child Process ID: 3673
The Python code above first creates a child process and stores the process ID in pid
. A child process was successfully executed if the process ID is a non-zero positive value.
Since the same instructions are being executed by two processes concurrently, parent and child, we use if else
blocks to separate instructions for each process. Code under if pid > 0:
is meant for the parent process (parent code) and code under elif pid == 0:
is meant for the child process (child code).
In the parent code, we use the os.getpid()
method to get the process ID of the parent process. On the flip side, in the child code, we use the os.getpid()
method to get the process ID of the child process and the os.getppid()
method to get the process ID of the parent process.
The output verifies that the process IDs retrieved are correct.