Python os.getlogin() Method
-
Syntax of Python
os.getlogin()
Method -
Example 1: The
os.getlogin()
Method in Python -
Example 2: Fix the
OSError: Inappropriate ioctl for device
in Python -
Example 3: Logging Using the
os.getlogin()
Method in Python - Conclusion
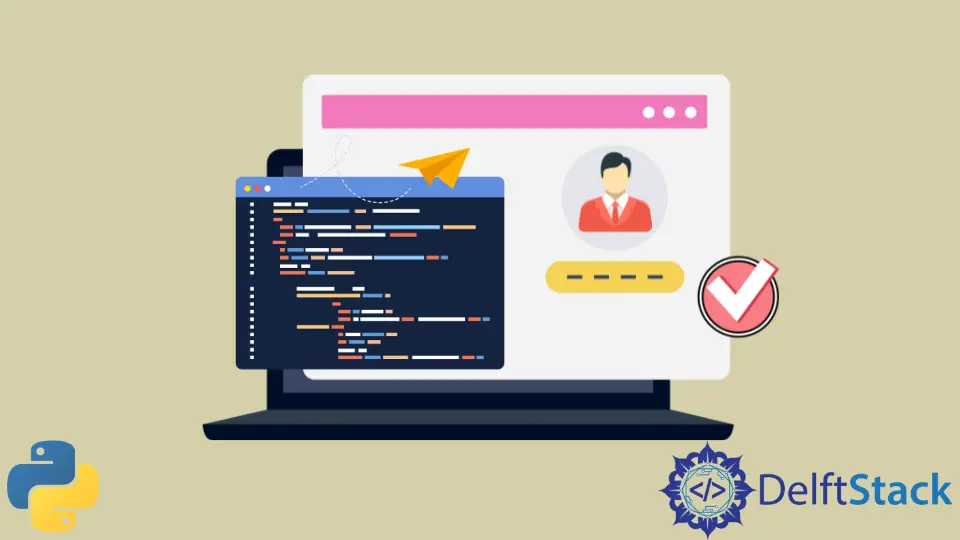
One way to obtain the login name of the current user is through the getlogin()
method.
This method, available in the os
module, provides a simple and reliable way to retrieve the user’s login name currently running the script or program.
This method returns the name of the currently logged-in user.
Syntax of Python os.getlogin()
Method
os.getlogin()
Parameters
This method doesn’t accept any parameters.
Return
The getlogin()
method returns the logged-in user’s name.
Example 1: The os.getlogin()
Method in Python
In the code snippet below, we are utilizing the os
module in Python to retrieve the current user’s login name using the os.getlogin()
method.
The login name represents the username of the individual currently executing the Python script or program.
This information can be valuable in scenarios where personalization or user-specific actions are required within the application.
Code:
import os
user = os.getlogin()
print(user)
The Python os
module is imported to leverage its functionalities for interacting with the operating system.
Specifically, the getlogin()
method, inherent to the os
module, is employed to retrieve the user’s login name executing the Python script.
The obtained login name is stored in a variable named user.
Subsequently, the print()
function displays the user’s login name on the console.
Output:
The output shows the name of the currently logged-in user.
Please note that os.getlogin()
might not work on all platforms, especially if the user is logged in via SSH or another remote login protocol
Example 2: Fix the OSError: Inappropriate ioctl for device
in Python
A controlling terminal refers to one that assigns a user the power to control the execution of tasks or jobs during the active session. Without such a terminal, the getlogin()
method throws the following error.
OSError: [Errno 25] Inappropriate ioctl for device
We can use the getpass.getuser()
method to solve this problem. The getpass
module is an in-built Python library that helps read user inputs as passwords.
This module has a method getuser()
that returns the logged-in user.
This method checks environment variables to resolve the logged-in user’s name. Namely, LOGNAME,
USER,
LNAME,
and USERNAME,
in this specific order, return the first non-empty string value.
Generally, environment variables in systems are used to store sensitive details about applications for security purposes. Hence, they become an ideal spot to search for information.
If this method fails to resolve a name from these environment variables, it looks up to the pwd
module. The pwd
stands for Password Database
.
Note that this module is only available in UNIX-based systems. The pwd
module grants access to user details and its password database.
One of its methods, getpwuid()
, helps retrieve the password database for a given numeric user ID. The user ID can be retrieved using the os.getuid()
method.
Putting all this together, we get pwd.getpwuid(os.getuid())[0]
that helps reveal the logged in user’s name.
Refer to the following Python code for the solution.
Code:
import getpass
print(getpass.getuser())
In the provided code snippet, we are using the getpass
module in Python to obtain the login name of the current user.
The getuser()
function from the getpass
module is employed for this purpose.
This code is particularly useful when you need to retrieve the user’s login information securely, as getpass
is designed to handle password-related functionality without echoing the input.
Output:
The output shows the name of the currently logged-in user.
The getuser()
function provides a secure way to access user information, making it especially useful in scenarios where password-related input is involved.
Example 3: Logging Using the os.getlogin()
Method in Python
In the code snippet below, we are using the os
module in Python to obtain the login name of the current user within a function called log_activity
.
The purpose of this code is to log user activities by associating them with the user’s login name. The os.getlogin()
method is utilized to retrieve the login name, and the function takes an “action” parameter, representing a specific activity.
The output serves as a simple logging mechanism, capturing the user and the corresponding action performed.
Code:
import os
def log_activity(action):
user = os.getlogin()
print(f"{user} performed action: {action}")
log_activity("save document")
The os
module is imported to access its features, notably the getlogin()
method, which is responsible for retrieving the login name of the user executing the script.
A function named log_activity
is introduced, designed to log user activities by associating them with the user’s login name.
Within this function, the os.getlogin()
method is employed to fetch the current user’s login name. A message is then printed, indicating both the user and the specific action performed.
Subsequently, the function is called with the argument save document
, exemplifying how it can be employed to log diverse user activities.
Output:
This code showcases a practical application of the os module for capturing user-related information in a logging context within a Python program.
Conclusion
In conclusion, the os.getlogin()
method in Python’s os
module serves as a valuable tool for retrieving the login name of the user executing a script or program.
The method provides a simple and reliable means of accessing the current user’s information, facilitating personalization and user-specific actions within applications. While the method may not work on all platforms, alternative solutions like getpass.getuser()
can be employed for secure user information retrieval.
Additionally, the code examples presented demonstrate the practical application of os.getlogin()
in various scenarios, from straightforward user name retrieval to logging user activities, showcasing its versatility in enhancing user-centric interactions within Python programs.